In this small recipe, we will create and understand an ActorSystem. Since we are done with our initial setup of the Hello-Akka project, we don't need to make another project for it. We need to import the SBT project in an IDE like IntelliJ Idea.
Creating and understanding ActorSystem
Getting ready
If you are using IntelliJ Idea, then you must have Scala and the SBT plugin install on it.
For importing an SBT Scala project in IntelliJ Idea, visit the following website:
https://www.jetbrains.com/help/idea/2016.1/getting-started-with-sbt.html?origin=old_help#import_project.
https://www.jetbrains.com/help/idea/2016.1/getting-started-with-sbt.html?origin=old_help#import_project.
How to do it...
It is very easy to create an ActorSystem in Akka:
- Create a package com.packt.chapter1 inside the Hello-Akka src folder. We will keep all the source code in this package.
- Inside the package, create a file, say HelloAkkaActorSystem.scala, which will contain the code.
- Create a small scala application object, HelloAkkaActorSystem, and create an ActorSystem inside it:
package com.packt.chapter1
Import akka.actor.ActorSystem
/**
* Created by user
*/
object HelloAkkaActorSystem extends App {
val actorSystem = ActorSystem("HelloAkka")
println(actorSystem)
}
- Now, run the application in IntelliJ Idea or in the console. It will print the output as follows:
akka://HelloAkka
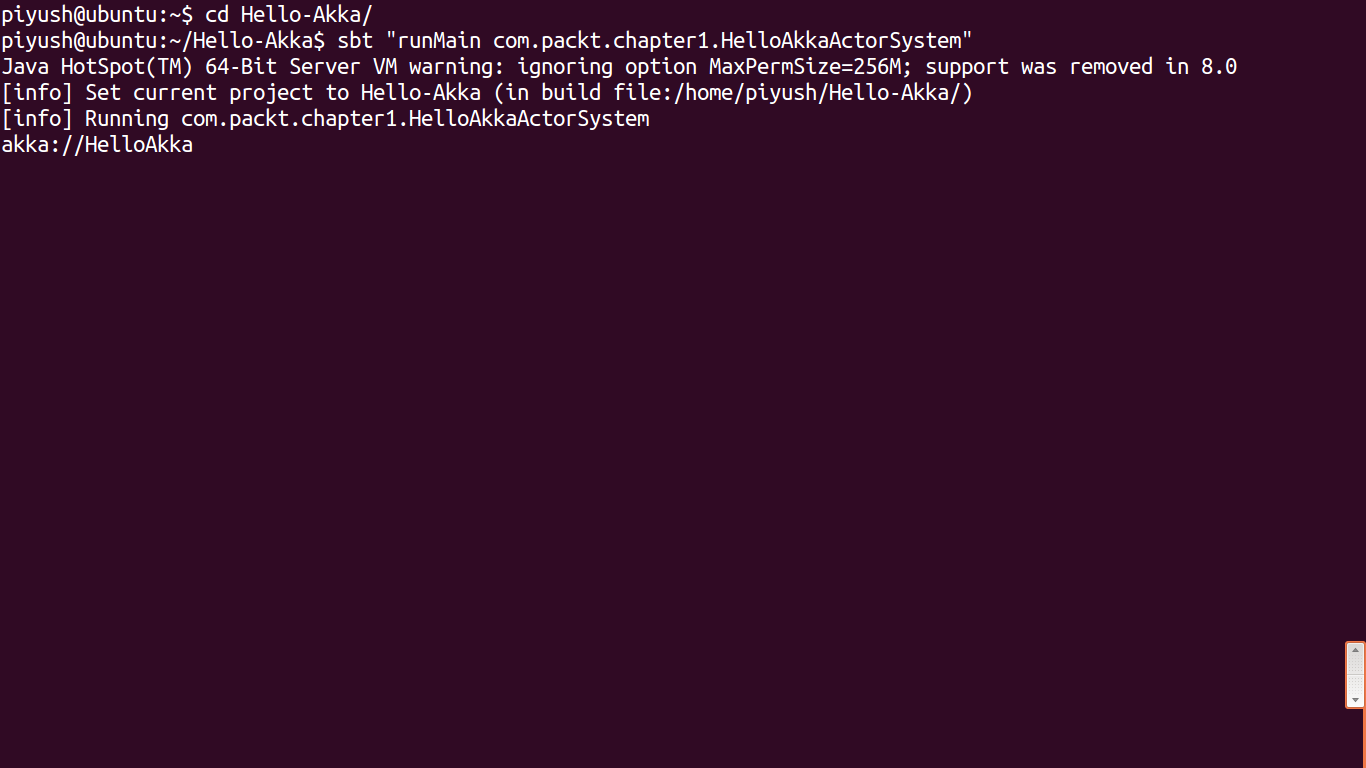
Downloading the Akka actor dependency
Here is a way to run a single Scala application using sbt from the console. Descend to the application root directory, and run the following command:
sbt "runMain com.packt.chapter1.HelloAkkaActorSystem"
sbt "runMain com.packt.chapter1.HelloAkkaActorSystem"
How it works...
In the recipe, we create a simple Scala object creating an ActorSystem, thereafter, we run the application.
Why we need ActorSystem
In Akka, an ActorSystem is the starting point of any Akka application that we write.
Technically, an ActorSystem is a heavyweight structure per application, which allocates n number of threads. Thus, it is recommended to create one ActorSystem per application, until we have a reason to create another one.
ActorSystem is the home for the actors in which they live, it manages the life cycle of an actor and supervises them.On creation, an ActorSystem starts three actors:
- /user - The guardian actor: All user-defined actors are created as a child of the parent actor user, that is, when you create your actor in the ActorSystem, it becomes the child of the user guardian actor, and this guardian actor supervises your actors. If the guardian actor terminates, all your actors get terminated as well.
- /system - The system guardian: In Akka, logging is also implemented using actors. This special guardian shut downs the logged-in actors when all normal actors have terminated. It watches the user guardian actor, and upon termination of the guardian actor, it initiates its own shutdown.
- / - The root guardian: The root guardian is the grandparent of all the so-called top-level actors, and supervises all the top-level actors. Its purpose is to terminate the child upon any type of exception. It sets the ActorSystem status as terminated if the guardian actor is able to terminate all the child actors successfully.
For more information on ActorSystem visit: http://doc.akka.io/docs/akka/2.4.1/general/actor-systems.html.