Deleting rows from a list
In this section, we will display a list of countries and use a swipe motion to delete items from the list one at a time.
Getting ready
Let's start by creating a SwiftUI app called DeleteRowFromList
.
How to do it…
We use the List
view's .onDelete(perform: …)
modifier to implement list deletion. The process is as follows:
- Add a state variable to the
ContentView
struct calledcountries
. The variable should contain an array of countries:@State var countries = ["USA", "Canada", "England","Cameroon", "South Africa", "Mexico" , "Japan", "South Korea"]
- Create a
List
view in theContentView
body that uses aForEach
loop to display the contents of thecountries
array:List { ForEach(countries, id: \.self) { country in Text(country) } }
- Add a
.onDelete(perform: self.deleteItem)
modifier to theForEach
loop. - Implement the
deleteItem()
function. The function should be placed below the body variable's closing brace:private func deleteItem(at indexSet: IndexSet){ self.countries.remove(atOffsets: indexSet) }
- Optionally, enclose the
List
view in a navigation view and add a.navigationBarTitle("Countries", displayMode: .inline)
modifier to the list. The resultingContentView
struct should be as follows:struct ContentView: View { @State var countries = ["USA", "Canada", "England","Cameroon", "South Africa", "Mexico" , "Japan", "South Korea"] var body: some View { NavigationView{ List { ForEach(countries, id: \.self) { country in Text(country) } .onDelete(perform: self.deleteItem) } .navigationBarTitle("Countries", displayMode: .inline) } } private func deleteItem(at indexSet: IndexSet){ self.countries.remove(atOffsets: indexSet) }
Run the canvas review by clicking the play button next to the canvas preview. A swipe from right to left on a row causes a Delete button to appear. Click on the button to delete the row:
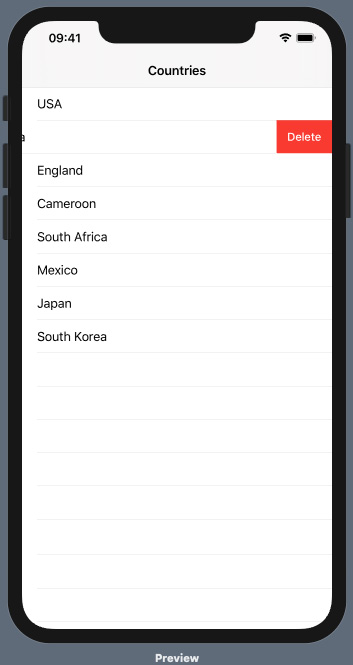
Figure 2.6 – DeleteRowFromList preview execution
Run the app live preview and admire the work of your own hands!
How it works…
Navigation views and list views were discussed earlier. Only the .onDelete(…)
modifier is new. The .onDelete(perform: self.deleteItem)
modifier triggers the deleteItem()
function when the user swipes from right to left.
The deleteItem(at indexSet: IndexSet)
function takes a single parameter, IndexSet
, which represents the index of the row to be removed/deleted. The .onDelete()
modifier automatically knows to pass the IndexSet
parameter to deleteItem(…)
.
There's more…
Deleting an item from a list view can also be performed by embedding the list in a navigation view and adding an EditButton
component.