In this section, we will review different types of data collections, such as as lists, tuples, and dictionaries. We will see methods and operations for managing these data structures and a practical example where we review the main use cases.
Python collections
Lists
Lists in Python are equivalent to structures as dynamic vectors in programming languages such as C. We can express literals by enclosing their elements between a pair of brackets and separating them with commas. The first element of a list has index 0. The indexing operator allows access to an element and is expressed syntactically by adding its index in brackets to the list, list [index].
Consider the following example: a programmer can construct a list by appending items using the append() method, print the items, and then sort them before printing again. In the following example, we define a list of protocols and use the main methods of a Python list as append, index, and remove:
>>> protocolList = []
>>> protocolList.append("ftp")
>>> protocolList.append("ssh")
>>> protocolList.append("smtp")
>>> protocolList.append("http")
>>> print protocolList ['ftp','ssh','smtp','http'] >>> protocolList.sort()
>>> print protocolList ['ftp','http','smtp','ssh'] >>> type(protocolList)
<type 'list'>
>>> len(protocolList) 4
>>> position = protocolList.index("ssh")
>>> print "ssh position"+str(position) ssh position 3 >>> protocolList.remove("ssh")
>>> print protocolList ['ftp','http','smtp'] >>> count = len(protocolList)
>>> print "Protocol elements "+str(count) Protocol elements 3
To print out the whole protocol list, use the following code. This will loop through all the elements and print them:
>>> for protocol in protocolList:
>> print (protocol) ftp
http
smtp
Lists also have methods, which help to manipulate the values inside them and allow us to store more than one variable inside it and provide a better method for sorting arrays of objects in Python. These are the most-used methods for manipulating lists:
- .append(value): Appends an element at the end of the list
- .count('x'): Gets the number of 'x' in the list
- .index('x'): Returns the index of 'x' in the list
- .insert('y','x'): Inserts 'x' at location 'y'
- .pop(): Returns the last element and also removes it from the list
- .remove('x'): Removes the first 'x' from the list
- .reverse(): Reverses the elements in the list
- .sort(): Sorts the list alphabetically in ascending order, or numerically in ascending order
Reversing a List
Another interesting operations that we have in lists is the one that offers the possibility of going back to the list through the reverse () method:
>>> protocolList.reverse()
>>> print protocolList ['smtp','http','ftp']
Another way to do the same operation use the -1 index. This quick and easy technique shows how you can access all the elements of a list in reverse order:
>>> protocolList[::-1]
>>> print protocolList ['smtp','http','ftp']
Comprehension lists
Comprehension lists allow you to create a new list of iterable objects. Basically, they contain the expression that must be executed for each element inside the loop that iterates over each element.
The basic syntax is:
new_list = [expression for_loop_one_or_more conditions]
List comprehensions can also be used to iterate over strings:
>>> protocolList = ["FTP", "HTTP", "SNMP", "SSH"]
>>> protocolList_lower= [protocol.lower() for protocol in protocolList]
>>> print(protocolList_lower) # Output: ['ftp', 'http', 'snmp', 'ssh']
Tuples
A tuple is like a list, but its size and elements are immutable, that is, its values cannot be changed nor can more elements be added than initially defined. A tuple is delimited by parentheses. If we try to modify an element of a tuple, we get an error indicating that the tuple object does not support the assignment of elements:
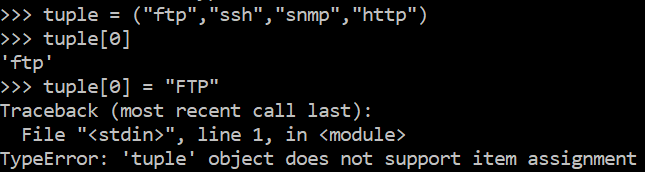
Dictionaries
The Python dictionary data structure allows us to associate values with keys. A key is any immutable object. The value associated with a key can be accessed with the indexing operator. In Python, dictionaries are implemented using hash tables.
A Python dictionary is a storage method for key:value pairs. Python dictionaries are enclosed in curly brackets, {}.Dictionaries, also called associative matrices, which owe their name to collections that relate a key and a value. For example, let's look at a dictionary of protocols with names and numbers:
>>> services = {"ftp":21, "ssh":22, "smtp":25, "http":80}
The limitation with dictionaries is that we cannot create multiple values with the same key. This will overwrite the previous value of the duplicate keys. Operations on dictionaries are unique. We can combine two distinct dictionaries into one by using the update method. Also, the update method will merge existing elements if they conflict:
>>> services = {"ftp":21, "ssh":22, "smtp":25, "http":80}
>>> services2 = {"ftp":21, "ssh":22, "snmp":161, "ldap":389}
>>> services.update(services2)
>>> print services
This will return the following dictionary:
{"ftp":21, "ssh":22, "smtp":25, "http":80,"snmp":161, "ldap":389}
The first value is the key and the second is the value associated with the key. As a key, we can use any immutable value: we could use numbers, strings, booleans, or tuples, but not lists or dictionaries, since they are mutable.
The main difference between dictionaries and lists or tuples is that the values stored in a dictionary are accessed not by their index, because they have no order, but by their key, using the [] operator again.
As in lists and tuples, you can also use this operator to reassign values:
>>> services["http"]= 8080
When constructing a dictionary, each key is separated from its value by a colon, and we separate items by commas. The .keys () method will return a list of all keys of a dictionary and the .items () method will return a complete list of elements in the dictionary.
Following are examples using these methods:
- services.keys() is method that will return all the keys in dictionary.
- services.items() is method that will return the entire list of items in dictionary.
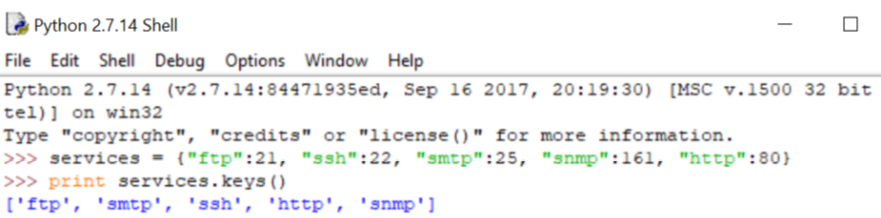
From the point of view of performance, the key within a dictionary is converted to a hash value when it is stored in order to save space and improve performance when searching or indexing the dictionary. It is also possible to print the dictionary and browse the keys in a specific order. The following code extracts the dictionary elements and then orders them:
>>> items = services.items()
>>> print items [('ftp', 21), ('smtp',25), ('ssh', 22), ('http', 80), ('snmp', 161)] >>> items.sort()
>>> print items [('ftp', 21), ('http', 80), ('smtp', 25), ('snmp', 161), ('ssh', 22)]
We can extract keys and values for each element in the dictionary:
>>> keys = services.keys()
>>> print keys ['ftp', 'smtp', 'ssh', 'http', 'snmp'] >>> keys.sort()
>>> print keys ['ftp', 'http', 'smtp', 'snmp', 'ssh'] >>> values = services.values()
>>> print values [21, 25, 22, 80, 161] >>> values.sort()
>>> print values [21, 22, 25, 80, 161] >>> services.has_key('http') True >>> services['http'] 80
Finally, you might want to iterate over a dictionary and extract and display all the "key:value" pairs:
>>> for key,value in services.items():
print key,value
ftp 21
smtp 25
ssh 22
http 80
snmp 161