Structure of an Angular application
We are about to take the first intrepid steps into examining our Angular application. The Angular CLI has already scaffolded our project and has carried much heavy lifting for us. All we need to do is fire up our favorite IDE and start working with the Angular project. We will use VS Code in this book, but feel free to choose any editor you are comfortable with:
- Open VS Code and select File | Open Folder… from the main menu.
- Navigate to the
my-app
folder and select it. VS Code will load the associated Angular project. - Navigate to the
src
folder.
When we develop an Angular application, we’ll likely interact with the src
folder. It is where we write the code and tests of our application. It is also where we define the styles of our application and any static assets we use, such as icons, images, and JSON files. It contains the following:
app
: Contains all the Angular-related files of the application. You interact with this folder most of the time during development.assets
: Contains static assets such as fonts, images, and icons.favicon.ico
: The icon displayed in the tab of your browser, along with the page title.index.html
: The main HTML page of the Angular application.main.ts
: The main entry point of the Angular application.styles.css
: Contains application-wide styles. These are CSS styles that apply globally to the Angular application. The extension of this file depends on the stylesheet format you choose when creating the application.
The app
folder contains the actual source code we write for our application. Developers spend most of their time inside that folder. The Angular application that is created automatically from the Angular CLI contains the following files:
app.component.css
: Contains CSS styles specific for the sample pageapp.component.html
: Contains the HTML content of the sample pageapp.component.spec.ts
: Contains unit tests for the sample pageapp.component.ts
: Defines the presentational logic of the sample pageapp.module.ts
: Defines the main module of the Angular application
The filename extension .ts
refers to TypeScript files.
In the following sections, we will learn about the purpose of each of those files in more detail.
Components
The files whose name starts with app.component
constitute an Angular component. A component in Angular controls part of a web page by orchestrating the interaction of the presentational logic with the HTML content of the page called a template. A typical Angular application has at least a main component called AppComponent
by convention.
Each Angular application has a main HTML file, named index.html
, that exists inside the src
folder and contains the following body
HTML element:
<body>
<app-root></app-root>
</body>
The app-root
tag is used to identify the main component of the application and acts as a container to display its HTML content. It instructs Angular to render the template of the main component inside that tag. We will learn how it works in Chapter 4, Enabling User Experience with Components.
When the Angular CLI builds an Angular app, it first parses the index.html
file and starts identifying HTML tag elements inside the body
tag. An Angular application is always rendered inside the body
tag and comprises a tree of components. When the Angular CLI finds a tag that is not a known HTML element, such as app-root
, it starts searching through the components of the application tree. But how does it know which components belong to the app?
Modules
The main module of our application is a TypeScript file that acts as a container for the main component. The component was registered with this module upon creating the Angular application; otherwise, the Angular framework would not be able to recognize and load it. A typical Angular application has at least a main module called AppModule
by convention.
The main module is also the starting point of an Angular application. The startup method of an Angular application is called bootstrapping, and it is defined in the main.ts
file inside the src
folder:
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { AppModule } from './app/app.module';
platformBrowserDynamic().bootstrapModule(AppModule)
.catch(err => console.error(err));
The main task of the bootstrapping file is to define the module that will be loaded at application startup. It calls the bootstrapModule
method of the platformBrowserDynamic
method and passes AppModule
as the entry point of the application.
As we have already learned, Angular can run on different platforms. The platformBrowserDynamic
method that we use targets the browser platform.
We will learn more about the capabilities of Angular modules in Chapter 3, Organizing Application into Modules.
Template syntax
Now that we have taken a brief overview of our sample application, it’s time to start interacting with the source code:
- Run the following command in a terminal to start the application:
ng serve
- Open the application with your browser at
http://localhost:4200
and notice the text next to the rocket icon that reads my-app app is running! The word my-app that corresponds to the name of our application comes from a variable declared in the TypeScript file of the main component. Open theapp.component.ts
file and locate thetitle variable
:import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'my-app'; }
The
title
variable is a property of the component and is currently used in the component template.
- Open the
app.component.html
file and go to line 344:<span>{{ title }} app is running!</span>
The
title
property is surrounded by double curly braces syntax called interpolation, which is part of the Angular template syntax. In a nutshell, interpolation converts the value of thetitle
property to text and displays it on the page.Angular uses template syntax to extend and enrich the standard HTML syntax in the template of an application. We will learn more about the syntax used in Angular templates in Chapter 4, Enabling User Experience with Components.
- Change the value of the
title
property in the TypeScript class toLearning Angular
and examine the application in the browser:
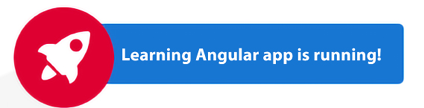
Figure 1.2: Landing page title
Congratulations! You have successfully used the Angular CLI to interact with the Angular application.
By now, you should have a basic understanding of how Angular works and what are the basic parts of a sample Angular application. As a reader, you had to swallow much information at this point. However, you will get a chance to get more acquainted with the components and modules in the upcoming chapters. For now, the focus is to get you up and running by giving you a powerful tool like the Angular CLI and showing you how just a few steps are needed to display an application on the screen.