Classification
Unlike regression, where you predict a continuous number, you use classification to predict a category. We will cover logistic regression here.
We will use a dataset of historical data of iPhone purchases, based on the age and the salary of the buyers, to predict whether a new potential buyer will purchase an iPhone.
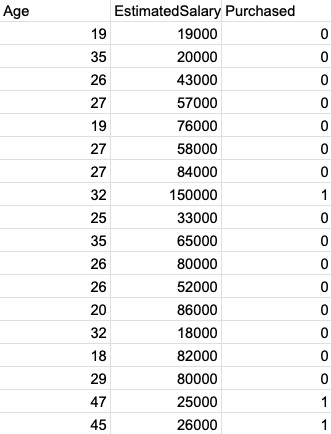
Let’s do the preparation first:
import numpy as np import pandas as pd import matplotlib.pyplot as plt dataset = pd.read_csv('Social_Network_Ads.csv') X = dataset.iloc[:,:-1].values y = dataset.iloc[:, -1].values from sklearn.model_selection import train_test_split X_train, X_test, y_train, y_test = train_test_split(X, y , test_size = 0.2, random_state=1) from sklearn.preprocessing import StandardScaler sc = StandardScaler() X_train = sc.fit_transform(X_train) X_test = sc.transform(X_test) print(X_train) [[-0.8 -1.19] [ 0.76 -1.37] [ 0.85 1.44] [-0.51 -1.49] [-1.49 0.38] ...