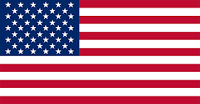

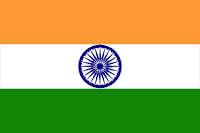
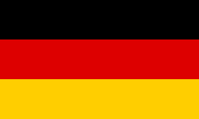
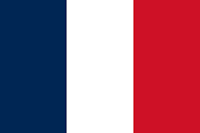

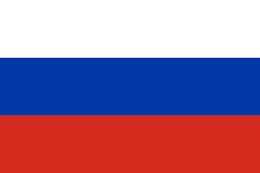
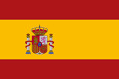



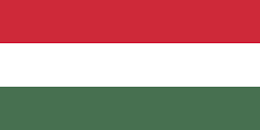

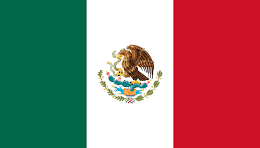
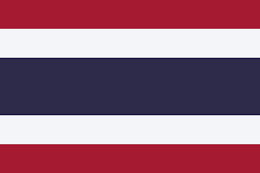
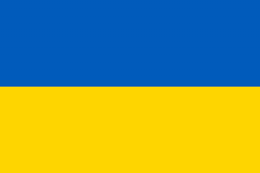
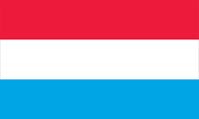

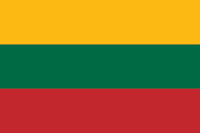

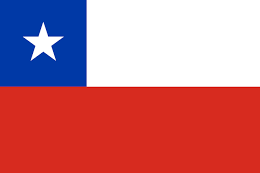

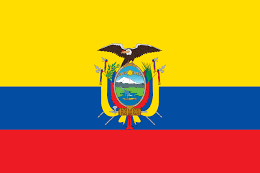

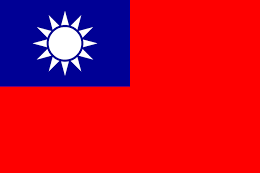

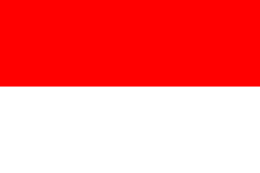
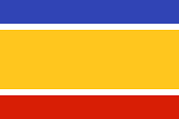
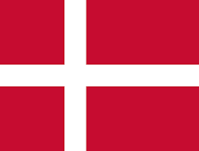
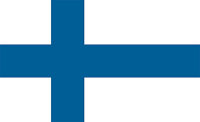


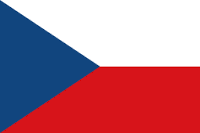
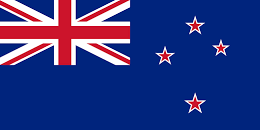
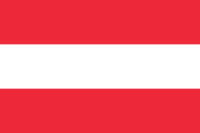
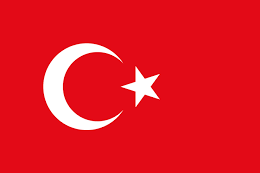
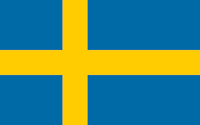
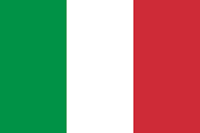
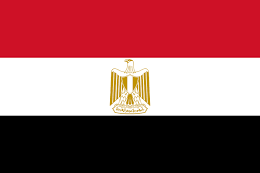

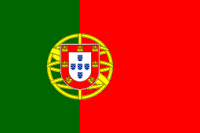
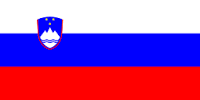

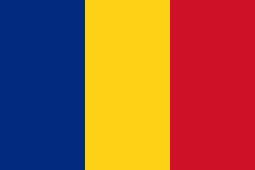
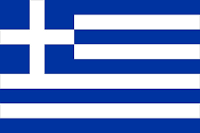

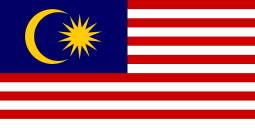
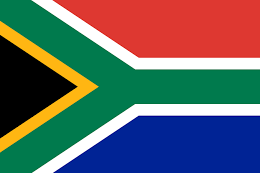

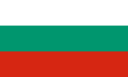
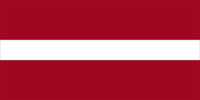


Using the ChatGPT API with Python is a relatively simple process. You'll first need to make sure you create a new PyCharm project called ChatGPTResponse as shown in the following screenshot:
Fig 1: New Project Window in PyCharm
Once you have that setup, you can use the OpenAI Python library to interact with the ChatGPT API. Open a new Terminal in PyCharm, make sure that you are in your project folder, and install the openai
package:
$ pip install openai
Next, you need to create a new Python file in your PyCharm project on the left top corner perform a Right-click on the folder ChatGPTResponse | New | Python File. Name the file app.py
and click hit Enter. You should now have a new Python file in your project directory:
Fig 2: New Python File
To get started, you'll need to import the openai
library into your Python file. Also, you'll need to provide your OpenAI API Key. You can obtain an API Key from the OpenAI website by following the steps outlined in the previous sections of this book. Then you'll need to set it as a parameter in your Python code. Once your API Key is set up, you can start interacting with the ChatGPT API.
import openai
openai.api_key = "YOUR_API_KEY"
Replace YOUR_API_KEY
with the API key you obtained from the OpenAI platform page. Now, you can ask the user a question using the input()
function:
question = input("What would you like to ask ChatGPT? ")
The input()
function is used to prompt the user to input a question they would like to ask the ChatGPT API. The function takes a string as an argument, which is displayed to the user when the program is run. In this case, the question string is "What would you Like to ask ChatGPT?"
. When the user types their question and presses enter, the input()
function will return the string that the user typed. This string is then assigned to the variable question.
To pass the user question from your Python script to ChatGPT, you will need to use the ChatGPT API Completion function:
response = openai.Completion.create(
engine="text-davinci-003",
prompt=question,
max_tokens=1024,
n=1,
stop=None,
temperature=0.8,
)
The openai.Completion.create()
function in the code is used to send a request to the ChatGPT API to generate a completion of the user's input prompt. The engine
parameter specifies the ChatGPT engine to use for the request, and in this case, it is set to "text-davinci-003"
. The prompt parameter specifies the text prompt for the API to complete, which is the user's input question in this case.
The max_tokens
parameter specifies the maximum number of tokens the response should contain. The n parameter specifies the number of completions to generate for the prompt. The stop parameter specifies the sequence where the API should stop generating the response.
The temperature
parameter controls the creativity of the generated response. It ranges from 0
to 1
. Higher values will result in more creative but potentially less coherent responses, while lower values will result in more predictable but potentially fewer interesting responses. Later in the book, we will delve into how these parameters impact the responses received from ChatGPT.
The function returns a JSON
object containing the generated response from the ChatGPT API, which then can be accessed and printed to the console in the next line of code.
print(response)
In the project pane on the left-hand side of the screen, locate the Python file you want to run. Right-click on the app.py
file and select Run app.py from the context menu. You should receive a message in the Run window that asks you to write a question to the ChatGPT.
Fig 3: Run Window
Once you have entered your question, press the Enter key to submit your request to the ChatGPT API. The response generated by the ChatGPT API model will be displayed in the Run window as a complete JSON
object:
{
"choices": [
{
"finish_reason": "stop",
"index": 0,
"logprobs": null,
"text": "\n\n1. Start by getting in the water. If you're swimming in a pool, you can enter the water from the side, ………….
}
],
"created": 1681010983,
"id": "cmpl-73G2JJCyBTfwCdIyZ7v5CTjxMiS6W",
"model": "text-davinci-003",
"object": "text_completion",
"usage": {
"completion_tokens": 415,
"prompt_tokens": 4,
"total_tokens": 419
}
}
This JSON
response produced by the OpenAI API contains information about the response generated by the GPT-3 model. This response consists of the following fields:
choices
field contains an array of objects with the generated responses, which in this case only contains one response object. text
field within the response object contains the actual response generated by the GPT-3 model.finish_reason
field indicates the reason why the response was generated; in this case it was because the model reached the stop
condition specified in the API request.created
field specifies the Unix timestamp of when the response was created. id
field is a unique identifier for the API request that generated this response.model
field specifies the GPT-3 model that was used to generate the response. object
field specifies the type of object that was returned, which in this case is text_completion
.usage
field provides information about the resource usage of the API request. It contains information about the number of tokens used for the completion, the number of tokens in the prompt, and the total number of tokens used.The two most important parameter from the response is the text field, that contains the answer to the question asked to ChatGPT API. This is why most API users would like to access only that parameter from the JSON object. You can easily separate the text from the main body as follows:
answer = response["choices"][0]["text"]
print(answer)
By following this approach, you can guarantee that the variable answer
will hold the complete ChatGPT API text response, which you can then print to verify. Keep in mind that ChatGPT responses can significantly differ depending on the input, making each response unique.
OpenAI:
1. Start by getting in the water. If you're swimming in a pool, you can enter the water from the side, ladder, or diving board. If you are swimming in the ocean or lake, you can enter the water from the shore or a dock.
2. Take a deep breath in and then exhale slowly. This will help you relax and prepare for swimming.
In this tutorial, you implemented a simple ChatGPT API response, by sending a request to generate a completion of a user's input prompt/question. You have also learned how to set up your API Key and how to prompt the user to input a question, and finally, how to access the generated response from ChatGPT in the form of a JSON object containing information about the response.
Martin Yanev is an experienced Software Engineer who has worked in the aerospace and medical industries for over 8 years. He specializes in developing and integrating software solutions for air traffic control and chromatography systems. Martin is a well-respected instructor with over 280,000 students worldwide, and he is skilled in using frameworks like Flask, Django, Pytest, and TensorFlow. He is an expert in building, training, and fine-tuning AI systems with the full range of OpenAI APIs. Martin has dual Master's degrees in Aerospace Systems and Software Engineering, which demonstrates his commitment to both practical and theoretical aspects of the industry.