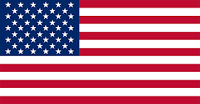

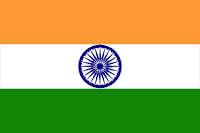
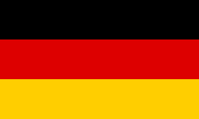
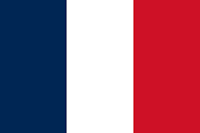

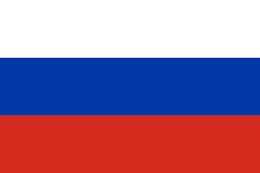
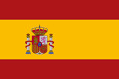



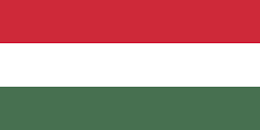
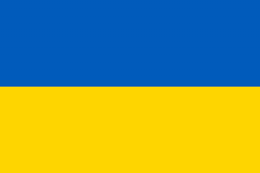
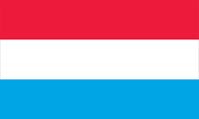

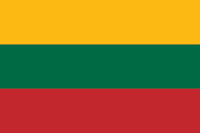

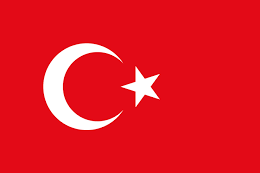


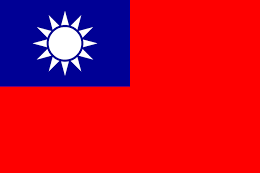
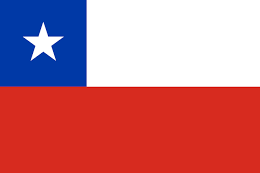

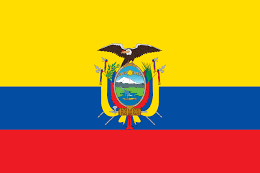
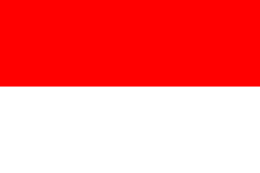
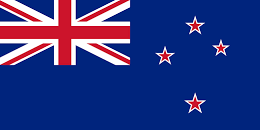
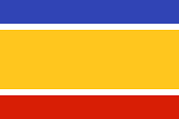
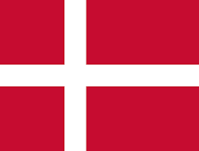
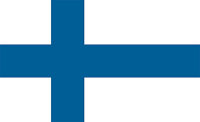


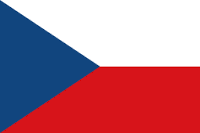
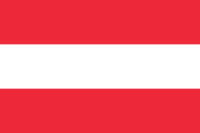
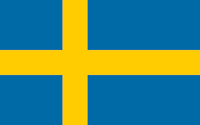
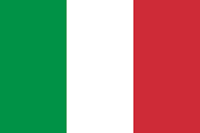
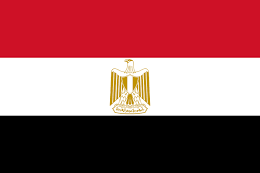

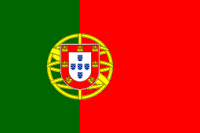
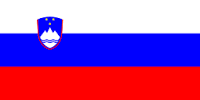

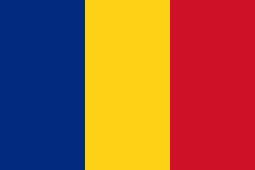
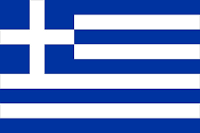


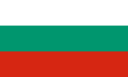
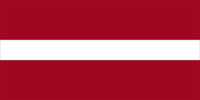
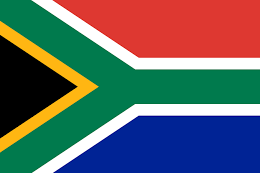
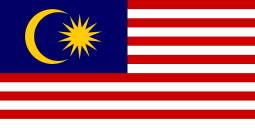



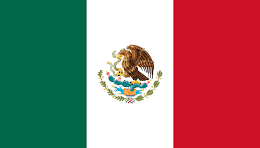
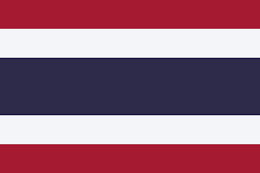
We will first look at the animation of objects as a whole. The most common ways to animate an object are rotation and translation (movement). We will begin by creating a class that will interpolate a position and rotation value between two extremes over a given amount of time. We could also have it interpolate between two scaling values, but it is very uncommon for an object to change size in a smooth manner during gameplay, so we will leave it out for simplicity's sake.
The ObjectAnimation class has a number of parameters—starting and ending position and rotation values, a duration to interpolate during those values, and a Boolean indicating whether or not the animation should loop or just remain at the end value after the duration has passed:
public class ObjectAnimation
{
Vector3 startPosition, endPosition, startRotation, endRotation;
TimeSpan duration;
bool loop;
}
We will also store the amount of time that has elapsed since the animation began, and the current position and rotation values:
TimeSpan elapsedTime = TimeSpan.FromSeconds(0);
public Vector3 Position { get; private set; }
public Vector3 Rotation { get; private set; }
The constructor will initialize these values:
public ObjectAnimation(Vector3 StartPosition, Vector3 EndPosition,
Vector3 StartRotation, Vector3 EndRotation, TimeSpan Duration,
bool Loop)
{
this.startPosition = StartPosition;
this.endPosition = EndPosition;
this.startRotation = StartRotation;
this.endRotation = EndRotation;
this.duration = Duration;
this.loop = Loop;
Position = startPosition;
Rotation = startRotation;
}
Finally, the Update() function takes the amount of time that has elapsed since the last update and updates the position and rotation values accordingly:
public void Update(TimeSpan Elapsed)
{
// Update the time
this.elapsedTime += Elapsed;
// Determine how far along the duration value we are (0 to 1)
float amt = (float)elapsedTime.TotalSeconds / (float)duration.
TotalSeconds;
if (loop)
while (amt > 1) // Wrap the time if we are looping
amt -= 1;
else // Clamp to the end value if we are not
amt = MathHelper.Clamp(amt, 0, 1);
// Update the current position and rotation
Position = Vector3.Lerp(startPosition, endPosition, amt);
Rotation = Vector3.Lerp(startRotation, endRotation, amt);
}
As a simple example, we'll create an animation (in the Game1 class) that rotates our spaceship in a circle over a few seconds:
We'll also have it move the model up and down for demonstration's sake:
ObjectAnimation anim;
We initialize it in the constructor:
models.Add(new CModel(Content.Load<Model>("ship"),
Vector3.Zero, Vector3.Zero, new Vector3(0.25f), GraphicsDevice));
anim = new ObjectAnimation(new Vector3(0, -150, 0),
new Vector3(0, 150, 0),
Vector3.Zero, new Vector3(0, -MathHelper.TwoPi, 0),
TimeSpan.FromSeconds(10), true);
We update it as follows:
anim.Update(gameTime.ElapsedGameTime);
models[0].Position = anim.Position;
models[0].Rotation = anim.Rotation;