Navigating options market data with the OpenBB Platform
Options are exchange-listed derivative contracts that convey the right (but not the obligation) to buy or sell the underlying stock at a certain price on or before a certain expiration date. Options are among the most versatile financial instruments in the market. They allow traders to define their risk profiles before entering trades and express market views not only on the direction of the underlying but the volatility. While options offer a high degree of flexibility for trading, this feature complicates data collection for research and backtesting.
A single underlying stock can have an array of options contracts with different combinations of strike prices and expiration dates. Collecting and manipulating this data is a challenge. The combination of options contracts for all strikes and expiration dates is commonly referred to as an options chain. There can be thousands of individual options contracts at a given time for a single underlying stock. Not only does the number of individual contracts pose a challenge, but getting price data has historically been expensive. With the introduction of the OpenBB Platform, it is now only a few lines of Python code to download options chains into a pandas DataFrame. This recipe will walk you through acquiring options data using the OpenBB Platform.
Getting ready…
By now, you should have the OpenBB Platform installed in your virtual environment. If not, go back to the beginning of this chapter and get it set up.
How to do it…
Similar to how we used the OpenBB Platform for futures data, we can use it for options data too:
- Import the OpenBB Platform and Matplotlib for visualization:
from openbb import obb obb.user.preferences.output_type = "dataframe"
- Use the
chains
method to download the entire options chain:chains = obb.derivatives.options.chains(symbol="SPY")
- Inspect the resulting DataFrame:
chains.info()
By running the preceding code, we’ll see the details of the options chains data:
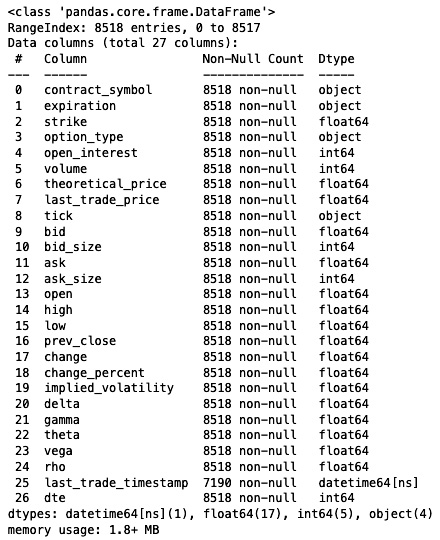
Figure 1.10: Preview of the data downloaded for the SPY options chains
Note that there are 8,518 options contracts for the SPY Exchange Traded Fund (ETF) that can be downloaded from CBOE (for free).
How it works…
The obb.derivatives.options.chains
method downloads the entire options chain and stores it in a pandas DataFrame. The obb.derivatives.options.chains
has an additional optional parameter:
provider
: The source from which the data should be downloaded. The default is CBOE. You can also select Tradier, Intrinio, or TMX. Note that for Tradier, Intrinio, and TMX, you need to provide your API key, which can be configured in the OpenBB Hub.
There’s more…
You can use the OpenBB Platform to download historical options data for a single contract. To do this, you need the option symbol.
We’ll use the obb.equity.price.historical
method to get the historical options data for an SPY call option with a strike price of $550 expiring on December 20, 2024:
data = obb.equity.price.historical( symbol="SPY241220C00550000", provider="yfinance" )[["close", "volume"]]
The result is a pandas DataFrame with the closing price and volume of the options contract.
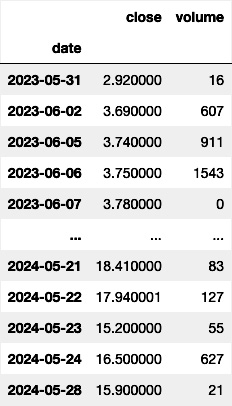
Figure 1.11: Closing prices and volume of the SPY options contract
Options Greeks
Options Greeks measure how options prices change given a change in one of the inputs to an options pricing model. For example, delta measures how an options price changes given a change in the underlying stock price.
Using obb.derivatives.options.chains
, the OpenBB Platform returns the most used Greeks including Delta, Gamma, Theta, Vega, and Rho.
See also
Options are a fascinating and deep topic that is rich with opportunities for trading. You can learn more about options, volatility, and how to analyze both via the OpenBB Platform:
- Free articles, code, and other resources for options trading: https://pyquantnews.com/free-python-resources/options-trading-with-python/
- Learn more about financial options if you’re unfamiliar with them: https://en.wikipedia.org/wiki/Option_(finance)
- Documentation on the OpenBB Platform’s options methods: https://docs.openbb.co/platform/reference/derivatives/options