The pandas library provides a variety of file reading and writing options. In this section, we will learn about reading and writing CSV files. In order to read a CSV file, we will use the read_csv() method. Let's see an example:
# import pandas
import pandas as pd
# Read CSV file
sample_df=pd.read_csv('demo.csv', sep=',' , header=None)
# display initial 5 records
sample_df.head()
This results in the following output:
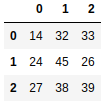
We can now save the dataframe as a CSV file using the following code:
# Save DataFrame to CSV file
sample_df.to_csv('demo_sample_df.csv')
In the preceding sample code, we have read and saved the CSV file using the read_csv() and to_csv(0) methods of the pandas module.
The read_csv() method has the following important arguments:
- filepath_or_buffer: Provides a file path or URL as a string to read a file.
- sep: Provides a separator in the string, for example, comma as ',' and semicolon as &apos...