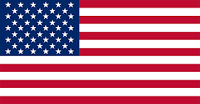

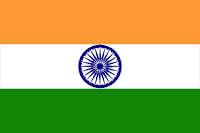
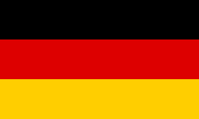
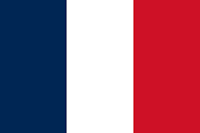

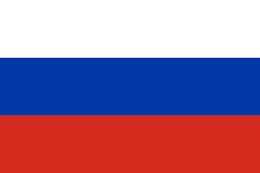
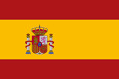



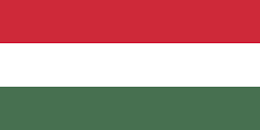
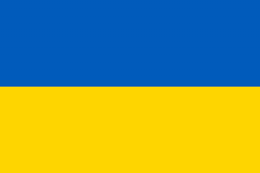
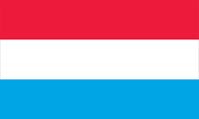

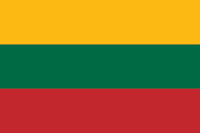

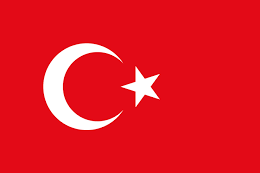


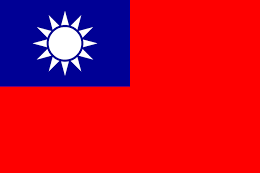
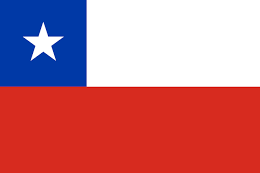

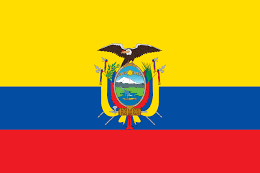
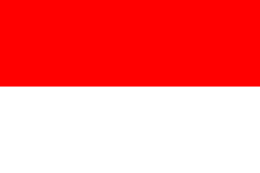
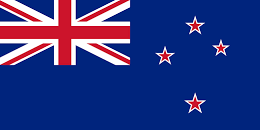
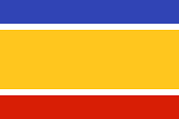
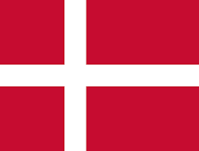
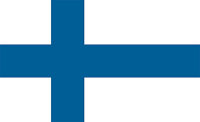


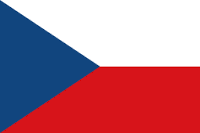
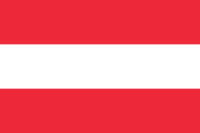
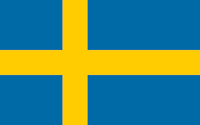
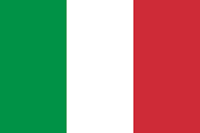
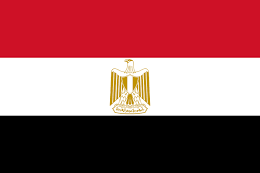

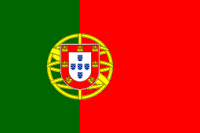
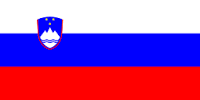

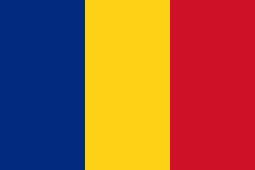
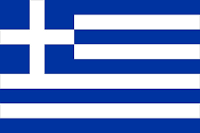


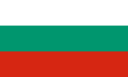
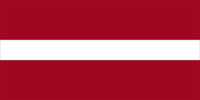
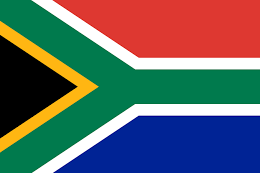
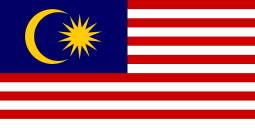



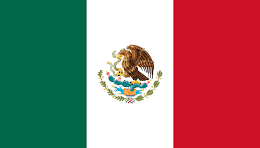
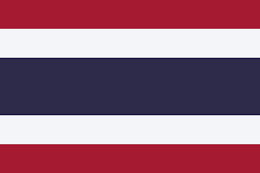
This article is an excerpt from the book, ASP.NET 8 Best Practices, by Jonathan R. Danylko. With the latest version of .NET 8.0 Core in LTS (Long-Term-Support), best practices are becoming harder to find as the technology continues to evolve. This book will guide you through coding practices and various aspects of software development.
In the ever-evolving landscape of web development, .NET 8 has emerged as a game-changer, especially in the realm of Web APIs. With new features and enhancements, .NET 8 prioritizes the ease and efficiency of building Web APIs, supported by robust tools in Visual Studio 2022. This chapter explores the innovations in .NET 8, focusing on creating and testing Web APIs seamlessly. From leveraging minimal APIs to utilizing Visual Studio's new features, developers can now build powerful REST-based services with simplicity and speed. We'll guide you through the process, demonstrating how to create a minimal API and highlighting the benefits of this approach.
In .NET 8, Web APIs take a front seat. Visual Studio has added new features to make Web APIs easier to build and test. For this chapter, we recommend using Visual Studio 2022, but the only requirement to view the GitHub repository is a simple text editor.
The code for Chapter 09 is located in Packt Publishing’s GitHub repository, found at https:// github.com/PacktPublishing/ASP.NET-Core-8-Best-Practices.
With .NET 8, APIs are integrated into the framework, making it easier to create, test, and document. In this section, we’ll learn a quick and easy way to create a minimal API using Visual Studio 2022 and walk through the code it generates. We’ll also learn why minimal APIs are the best approach to building REST-based services.
One of the features of .NET 8 is the ability to create minimal R EST APIs extremely fast. One way is to use the dotnet command-line tool and the other way is to use Visual Studio. To do so, follow these steps:
1. Open Visual Studio 2022 and create an ASP.NET Core Web API project.
2. After selecting the directory for the project, click Next.
3. Under the project options, make the following changes:
Figure 9.1 – Options for a web minimal API project
4. Click Create.
That’s it – we have a simple API! It may not be much of one, but it’s still a complete API with Swagger documentation. Swagger is a tool for creating documentation for APIs and implementing the OpenAPI specification, whereas Swashbuckle is a NuGet package that uses Swagger for implementing Microsoft APIs. If we look at the project, there’s a single file called Program.cs
.
Opening Program.cs
will show the entire application. This is one of the strong points of .NET – the ability to create a scaffolded REST API relatively quickly:
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
// Learn more about configuring Swagger/OpenAPI at
https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
var summaries = new[]
{
"Freezing", "Bracing", "Chilly", "Cool", "Mild",
"Warm", "Balmy", "Hot", "Sweltering", "Scorching"
};
app.MapGet("/weatherforecast", () =>
{
var forecast = Enumerable.Range(1, 5).Select(index
=>
new WeatherForecast
(
DateOnly.FromDateTime(DateTime.Now.AddDays
(index)),
Random.Shared.Next(-20, 55),
summaries[Random.Shared.Next(
summaries.Length)]
))
.ToArray();
return forecast;
})
.WithName("GetWeatherForecast")
.WithOpenApi();
app.Run();
internal record WeatherForecast(DateOnly Date,
int TemperatureC, string? Summary)
{
public int TemperatureF => 32 +
(int)(TemperatureC / 0.5556);
}
In the preceding code, we created our “application” through the .CreateBuilder()
method. We also added the EndpointsAPIExplorer
and SwaggerGen
services. EndpointsAPIExplore
r enables the developer to view all endpoints in Visual Studio, which we’ll cover later. The SwaggerGen service
, on the other hand, creates the documentation for the API when accessed through the browser. The next line creates our application instance using the .Build() method
.
Once we have our app instance and we are in development mode, we can add Swagger and the Swagger UI. .UseHttpsRedirection()
is meant to redirect to HTTPS when the protocol of a web page is HTTP to make the API secure.
The next line creates our GET weatherforecast route using .MapGet()
. We added the .WithName()
and .WithOpenApi()
methods to identify the primary method to call and let .NET know it uses the OpenAPI standard, respectively. Finally, we called app.Run().
If we run the application, we will see the documented API on how to use our API and what’s available. Running the application produces the following output:
Figure 9.2 – Screenshot of our documented Web API
If we call the /weatherforecast API
, we see that we receive JSON back with a 200 HTTP status.
Figure 9.3 – Results of our /weatherforecast API
Think of this small API as middleware with API controllers combined into one compact file (Program. cs).
I consider minimal APIs to be a feature in .NET 8, even though it’s a language concept. If the application is extremely large, adding minimal APIs should be an appealing feature in four ways:
Moving forward, we’ll take these minimal APIs and start looking at Visual Studio’s testing capabilities.
In conclusion, .NET 8 has revolutionized the process of building Web APIs by integrating them more deeply into the framework, making it easier than ever to create, test, and document APIs. By harnessing the power of Visual Studio 2022, developers can quickly set up minimal APIs, offering a streamlined and efficient approach to building REST-based services. The advantages of minimal APIs—being self-contained, performant, cross-platform, and self-documenting—make them an invaluable tool in a developer's arsenal. As we continue to explore the capabilities of .NET 8, the potential for creating robust and scalable web applications is limitless, paving the way for innovative and efficient software solutions.
Jonathan "JD" Danylko is an award-winning, full-stack ASP.NET architect. He's used ASP.NET as his primary way to build websites since 2002 and before that, Classic ASP.
Jonathan contributes to his blog (DanylkoWeb.com) on a weekly basis, has built a custom CMS, is a founder of Tuxboard (an open-source ASP.NET dashboard library), has been on various podcasts, and guest posted on the C# Advent Calendar for 6 years. Jonathan has worked in various industries for small, medium, and Fortune 100 companies, but currently works as an Architect at Insight Enterprise. The best way to contact Jonathan is through GitHub, LinkedIn, Twitter, email, or through the website.