Overview of JavaScript Capabilities
Without JavaScript, the web would be a fairly bland and non-interactive experience. As one of the core technologies used to build the web alongside HTML and CSS, JavaScript is immensely important for anyone working with these technologies today. JavaScript allows us to perform complex interactions, ferry data into your application, and display restructured values within web views. It even has the ability to build, destroy, and otherwise modify an entire HTML document.
Client-Side Form Validation
Forms are everywhere on the web—and the HTML specification includes a wide variety of inputs, checkboxes, radio groups, text areas, and more. Often, even before this data hits the server, you'll want to have some logic that looks for certain formatting peculiarities or other aberrations that are present that have been entered by the user. You can trigger initial client-side validation once the Submit
button has been clicked, or even as each input loses focus:
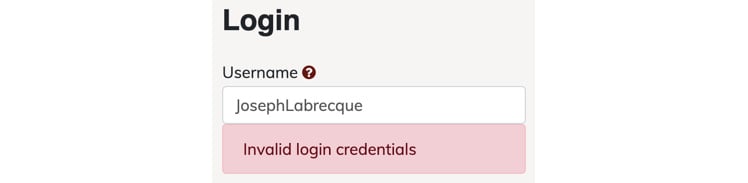
Figure 1.13: Form validation on a login
This is likely one of the most common uses for JavaScript on the web: you can provide basic feedback letting the user know they've done something in error—in this case, invalid login credentials.
JavaScript Widgets or Components
Whether using JavaScript snippets supplied by a component library such as Bootstrap or jQuery UI, or code supplied by specific vendors and services, people have been using JavaScript to include functional widgets and components for over two decades now. It truly is one of the most common uses for JavaScript on the web.
Normally, you are supplied a bit of code that often consists of both HTML and JavaScript. When it is run on the page, there is often either an embedded JavaScript library from which it can call functions, or a remote one, which then transforms the blank slate snippet into a fully functional piece of content for a specific purpose:
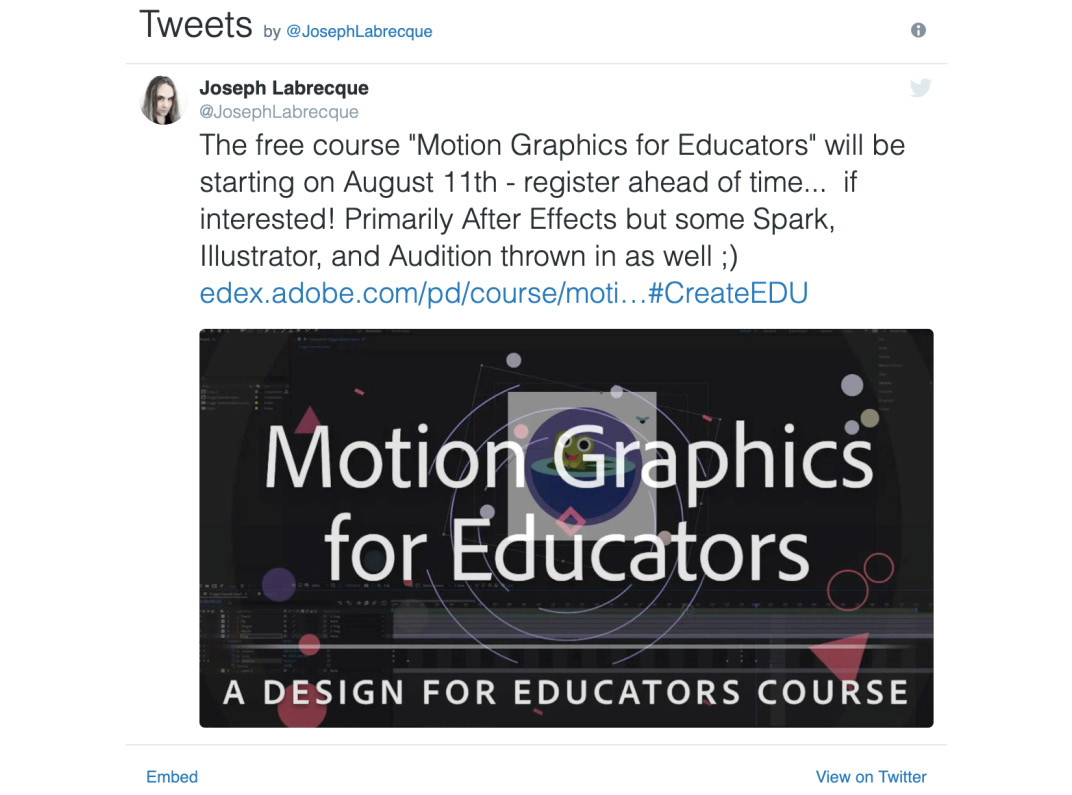
Figure 1.14: Embeddable Twitter widget
One of the best examples of a JavaScript-based component, or widget, is that of the Twitter timeline embed. You'll find similar embed types for Instagram and other social networks. Nearly all of them use JavaScript to insert dynamic content into a document.
Note
This is different from an <iframe>
embed, in that with an <iframe>
element, you are simply pulling in content from a remote resource and not building it on the fly.
XML HTTP Requests (XHR)
This technology was born from the concept of Rich Internet Applications (RIA), which has been dominated by technologies such as Adobe Flash Player and Microsoft Silverlight around the turn of the century. What made RIAs great is that you no longer had to refresh the browser view in its entirety in order to see changes in data presented in the browser DOM. Using something such as Flash Player as a visual interactive layer, ActionScript could be used within the application to perform all the tasks related to retrieving data in the background, with the user interface then changes based on the data retrieved. In this way, the user was presented with a much better experience as the entire document wouldn't have to load and reload with every server interaction.
As developers began searching for ways to accomplish this same thing without the use of additional technologies, XMLHttpRequest(XHR) was introduced as part of Microsoft Internet Explorer in 1999 as XMLHTTP. Other browser makers, recognizing the obvious benefits of this implementation, went on to standardize it across their interpretations as XMLHttpRequest.
Note
Previous to this more modern naming, XHR was commonly referred to as Asynchronous JavaScript and XML, abbreviated as AJAX. When people refer to AJAX, they are referring to the XHR API.
Press F12 and navigate to Network
| Preview
to view the XHR network preview in your browser:
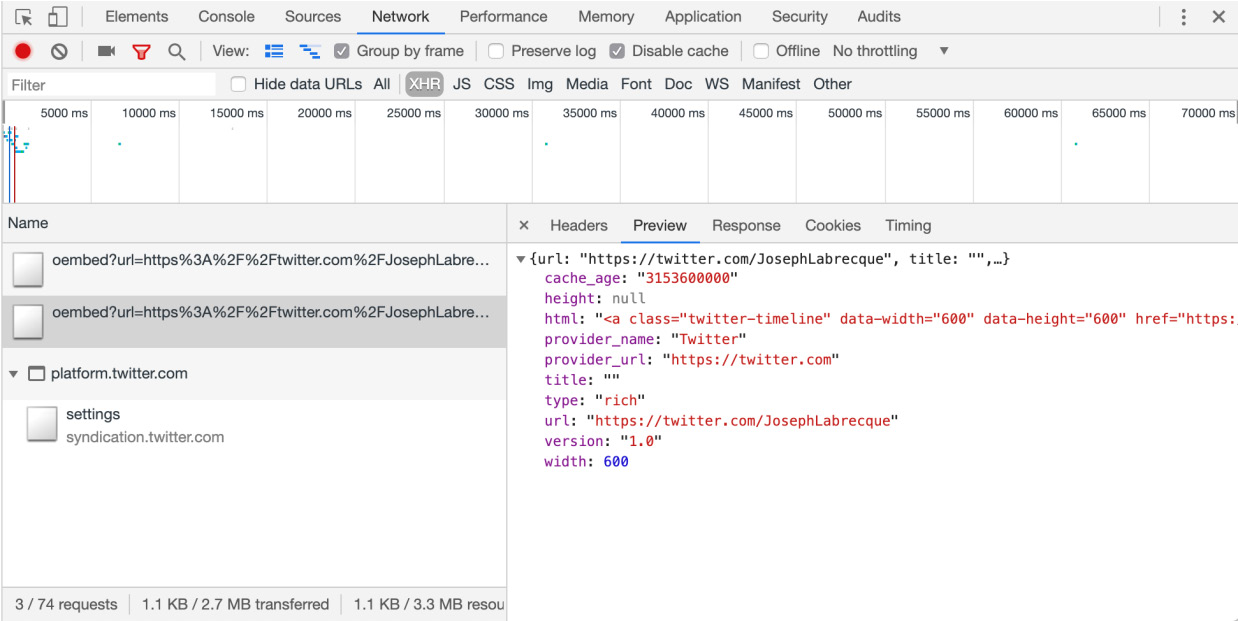
Figure 1.15: XHR network preview in Chrome
Browser developer tools all have a way of inspecting the files and data that are being transferred to and from the browser pertaining to the current website. For XHR, you can view raw header information, a formatted preview, and more.
Storing Local Data
Web browsers have been able to store local data for quite some time in the form of cookies. These are generally simple data:value
pairs that allow some sort of session memory on the client-side of an application. Eventually, the need arose for much more complex ways of storing local data within the browser as applications grew in complexity.
We now have the LocalStorage API, which was introduced with HTML5, as a more performant, more secure, and more expansive way of storing local website data. You can think of LocalStorage
as a better version of cookies all around, yet it still lacks the capabilities of a true database.
If you do need access to a real, client-side database for your web application, you'll want to explore the Indexed Database (IndexedDB) API. IndexedDB is a true client-side database and allows for complex data structures, relationships, and everything you'd expect from a database.
Note
Some web browsers also have access to a Web SQL database as well—but this is no longer considered appropriate by web standards bodies and should generally be avoided.
You can always check the local storage for any website you visit by digging into the developer tools. In Google Chrome, you will find local storage under the Application
 view:
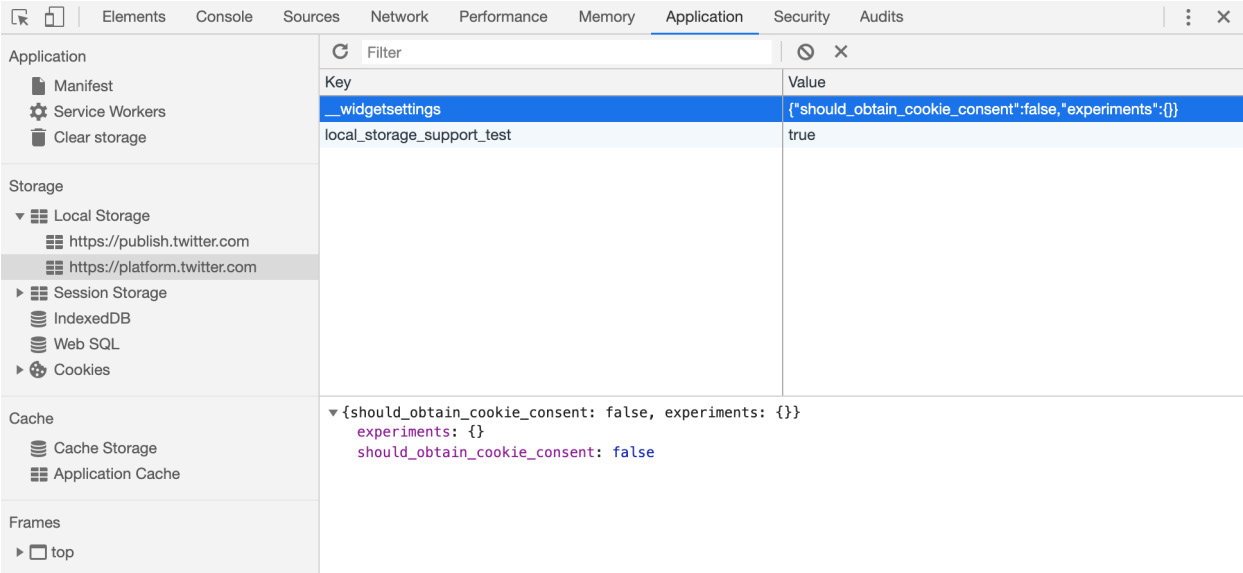
Figure 1.16: Local storage inspection in Chrome
DOM Manipulation
JavaScript can modify, create, and destroy elements and attributes within the Document Object Model (DOM). This is a very powerful aspect of JavaScript and nearly all modern development frameworks leverage this capability in some way. Similar to XHR, the browser's page doesn't need to be refreshed for us to perform these client-side amendments using JavaScript.
We'll see a particular project based on this in the next chapter, where you'll get some first-hand experience with this very task.
Animations and Effects
Looking back at the web in its infancy, everything was a very static experience. Pages were served up in the browser and consisted of text and hyperlinks. Depending on the year, we usually saw black serif type against a white background with the occasional blue/purple hyperlink.
Eventually, images and different visual stylistic attributes were available, but things really changed with the advent of various extensions such as Macromedia Shockwave and Flash Player. All of a sudden, rich experiences such as interactive video, animation, gaming, audio playback, special effects, and more were all available.
The web standards bodies rightly recognized that all of these capabilities should not be locked behind different browser plugins, but rather be part of the native web experience using core web technologies. Of course, primary among these was JavaScript, though JavaScript often relied on a close relationship with HTML and CSS to make things work. The following screenshot shows an interactive animation created using the CreateJS
library:
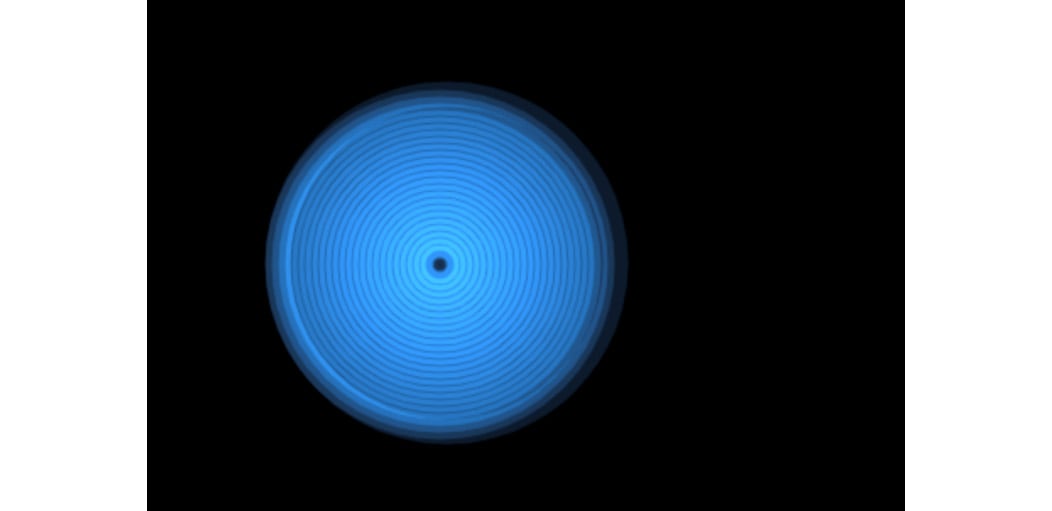
Figure 1.17: Interactive animation using the CreateJS JavaScript library
Today, we have rich implementations of the types of content creation that were previously only available with third-party plugins. Libraries such as CreateJS
allow for a huge variety of effects, gaming applications, interactions, animations, and beyond and use native JavaScript.
Note
For a number of examples of what is possible in this area of development, have a look at the Google Doodles archive at https://packt.live/2WZafHV.
In this section, we looked at some of the common capabilities of JavaScript that are used on the web today. In the chapters that follow, we'll be exploring some of these capabilities in much greater detail.