Creating classes based on adapter pattern
Another important design pattern is the adapter design. As the name suggests, the adapter design is used for conversion of one object into another object belonging to a different class. An adapter
class will have a method that takes as input the object reference that is to be converted and outputs it into the other object reference format.
We have referred to the cl_salv_tree_adapter
standard class while making of this recipe.
Getting ready
In order to demonstrate the adapter, we need two classes (input
class and output
class). The input
class will be the fac_meth_class
created earlier. For the output, we will create another class fac_meth_class2
. This will serve as the class, into the format of which the input object will be converted.
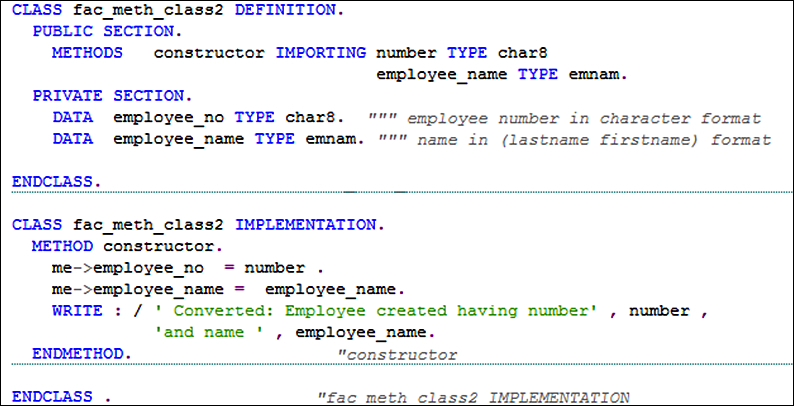
It is without a factory
method for sake of simplicity. It contains employee number and employee name but the format of these two is different from the classes shown in the previous recipes. The employee name of this class is based on data element emnam
, whereas the number is a character without zeros having length as eight. The name is of the form (firstname lastname
), meaning John Reed
will be stored as John Reed
and not Reed John as in the previous recipes. The constructor outputs the message, Converted employee created
.
We will use the same class used previously as the input object for the adapter method.
How to do it...
For creating a singleton
class, follow these steps:
Create a deferred definition of the
adapter
classadapter_meth_class
that we are going to create in the next step.Specify the
adapter_meth_class
as a friend of ourfact_meth_class
class in the definition via theFRIENDS
addition.The
adapter
class is then defined. It contains astatic adapter
method adapter that imports an object based onfac_meth_class
and returns one in thefac_meth_class2 format
.The implementation of the
adapter
class is then created. It contains the code of theadapter
method. Theadapter
method will convert the incoming number of the employee from numeric to character format. In addition, the name of the employee is converted to thefirstname lastname
format. The new object based on the second classfac_meth_class2
is then created and returned as an exporting parameter of the method.While calling the
adapter
method, you first create an object based on thefac_meth_class
class that is afactory
method (for illustrative purpose), similar to the previous recipe for the object referenceEMP
. This is then passed on to thestatic adapter
method of theadapter_meth_class
. Theadapter
class returns the converted object in the second format.
How it works...
When the program calls the static method adapter of the class adapter_meth_class , the code in the adapter method is executed. The adapter method calls the necessary code for converting the number into the character format and any zeros are removed from the number. In addition, the SPLIT statement is called for converting name of the employee in the (first name last name) format such as converting Reed John into John Reed. Finally the CREATE OBJECT is called in order to create the object in the converted class format . This triggers the constructor for the converted class fac_meth_class2 that outputs the message "Converted: Employee Created having number 1234" and name John Reed. Since we called the factory method of the original fac_meth_class before the adapter method call, the original constructor was also called and message printed also.

See also
Design Patterns in Object-Oriented ABAP published by SAP-Press