Our first screen will contain a list of the items we need to buy, so it will contain one list item per item we need to buy, including a button to mark that item as already bought. Moreover, we need a button to navigate to the AddProduct screen, which will allow us to add products to our list. Finally, we will add a button to clear the list of products, in case we want to start a new shopping list:
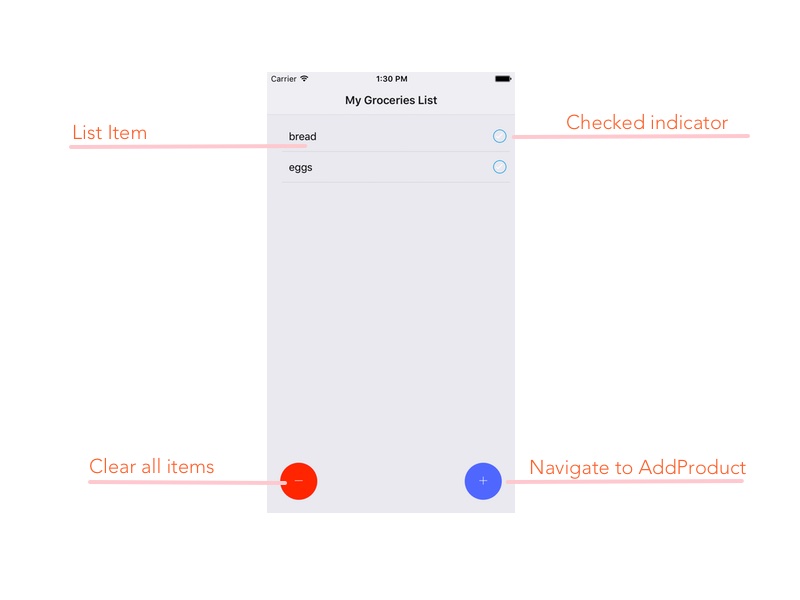
Let's start by creating ShoppingList.js inside the screens folder and importing all the UI components we will need from native-base and react-native (we will use an alert popup to warn the user before clearing all items). The main UI components we will be using are Fab (the blue and red round buttons), List, ListItem, CheckBox, Text, and Icon. To support our layout, we will be using Body, Container, Content, and Right, which are layout containers for the rest of our components.
Having all these components, we can create a simple version of our ShoppingList component:
/*** ShoppingList.js ***/
import React from 'react';
import { Alert } from 'react-native';
import {
Body,
Container,
Content,
Right,
Text,
CheckBox,
List,
ListItem,
Fab,
Icon
} from 'native-base';
export default class ShoppingList extends React.Component {
static navigationOptions = {
title: 'My Groceries List'
};
/*** Render ***/
render() {
return (
<Container>
<Content>
<List>
<ListItem>
<Body>
<Text>'Name of the product'</Text>
</Body>
<Right>
<CheckBox
checked={false}
/>
</Right>
</ListItem>
</List>
</Content>
<Fab
style={{ backgroundColor: '#5067FF' }}
position="bottomRight"
>
<Icon name="add" />
</Fab>
<Fab
style={{ backgroundColor: 'red' }}
position="bottomLeft"
>
<Icon ios="ios-remove" android="md-remove" />
</Fab>
</Container>
);
}
}
This is just a dumb component statically displaying the components we will be using on this screen. Some things to note:
- navigationOptions is a static attribute which will be used by <Navigator> to configure how the navigation would behave. In our case, we want to display My Groceries List as the title for this screen.
- For native-base to do its magic, we need to use <Container> and <Content> to properly form the layout.
- Fab buttons are placed outside <Content>, so they can float over the left and right-bottom corners.
- Each ListItem contains a <Body> (main text) and a <Right> (icons aligned to the right).
Since we enabled Live Reload in our first steps, we should see the app reloading after saving our newly created file. All the UI elements are now in place, but they are not functional since we didn't add any state. This should be our next step.