Motion detection and tracking
We will now build a sophisticated motion detection and tracking system with simple logic to find the difference between subsequent frames from a video feed, such as a webcam stream and plotting contours around the area where the difference is detected.
Let's import the required libraries and initialize the webcam:
import cv2 import numpy as np cap = cv2.VideoCapture(0)
We will need a kernel for the dilation operation that we will create in advance rather than creating it every time in the loop:
k=np.ones((3,3),np.uint8)
The following code will capture and store the subsequent frames:
t0 = cap.read()[1] t1 = cap.read()[1]
Now we initiate the while
loop and calculate the difference between the frames and convert the output to grayscale for further processing:
while(True): d=cv2.absdiff(t1,t0) grey = cv2.cvtColor(d, cv2.COLOR_BGR2GRAY)
The output will be as follows, and it shows difference of pixels between the frames:
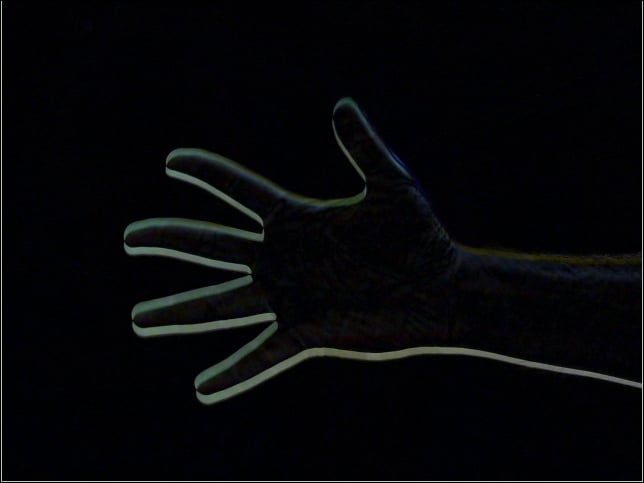
This image might contain some noise...