Writing optimized code
Key 1: Easy optimizations for code.
We should pay close attention to not use loops inside loops, giving us quadratic behavior. We can use built-ins, such as map, ZIP, and reduce, instead of using loops if possible. For example, in the following code, the one with map is faster because the looping is implicit and done at C level. By plotting their run times respectively on graph as test 1
and test 2
, we see that it is nearly constant for PyPy but reduces a lot for CPython, as follows:
def sqrt_1(ll): """simple for loop""" res = [] for i in ll: res.append(math.sqrt(i)) return res def sqrt_2(ll): "builtin map" return list(map(math.sqrt,ll)) The test 1 is for sqrt_1(list(range(1000))) and test 22 sqrt_2(list(range(1000))).
The following image is a graphical representation of the preceding code:
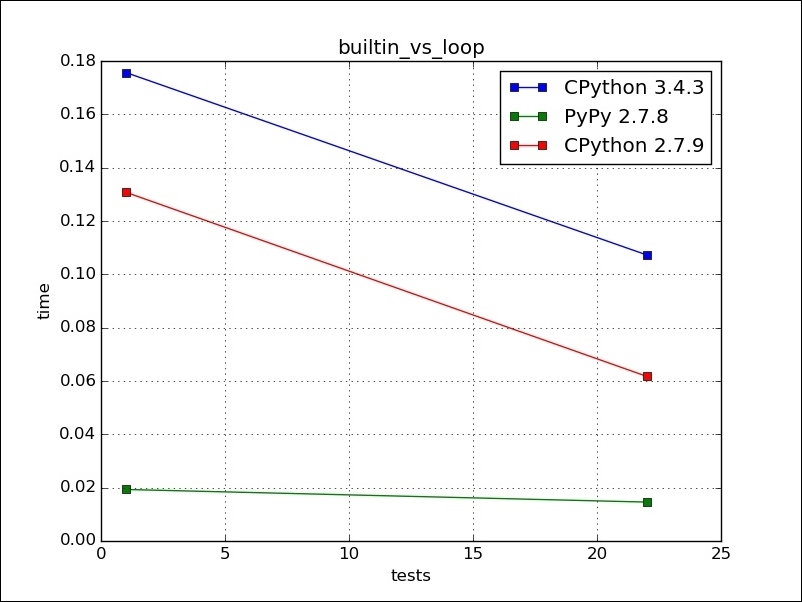
Generators should be used, when the result that is consumed is averagely smaller than the total result consumed. In other words, the result that is...