Creating a list of static items
Lists are similar to scroll views in that they are used to view a collection of items. Lists are used for larger datasets, whereas scroll views are used for smaller datasets; the reason being that list views do not load the whole dataset in memory at once and thus are more efficient at handling large data.
Getting ready
Let's start by creating a new SwiftUI app called StaticList
.
How to do it…
We'll create a struct to hold weather information and an array of weather data. The data will then be used to create a view that provides weather information from several cities. Proceed as follows:
- Before the
ContentView
struct, create a struct calledWeatherInfo
with four properties:id
,image
,temp
, andcity
:struct WeatherInfo: Identifiable { var id = UUID() var image: String var temp: Int var city: String }
- Within the
ContentView
struct, create aweatherData
property as an array ofWeatherInfo
:let weatherData: [WeatherInfo] = [ WeatherInfo(image: "snow", temp: 5, city:"New York"), WeatherInfo(image: "cloud", temp:5, city:"Kansas City"), WeatherInfo(image: "sun.max", temp: 80, city:"San Francisco"), WeatherInfo(image: "snow", temp: 5, city:"Chicago"), WeatherInfo(image: "cloud.rain", temp: 49, city:"Washington DC"), WeatherInfo(image: "cloud.heavyrain", temp: 60, city:"Seattle"), WeatherInfo(image: "sun.min", temp: 75, city:"Baltimore"), WeatherInfo(image: "sun.dust", temp: 65, city:"Austin"), WeatherInfo(image: "sunset", temp: 78, city:"Houston"), WeatherInfo(image: "moon", temp: 80, city:"Boston"), WeatherInfo(image: "moon.circle", temp: 45, city:"denver"), WeatherInfo(image: "cloud.snow", temp: 8, city:"Philadelphia"), WeatherInfo(image: "cloud.hail", temp: 5, city:"Memphis"), WeatherInfo(image: "cloud.sleet", temp:5, city:"Nashville"), WeatherInfo(image: "sun.max", temp: 80, city:"San Francisco"), WeatherInfo(image: "cloud.sun", temp: 5, city:"Atlanta"), WeatherInfo(image: "wind", temp: 88, city:"Las Vegas"), WeatherInfo(image: "cloud.rain", temp: 60, city:"Phoenix"), ]
- Add the
List
view to theContentView
body. The list should display the information contained in ourweatherData
array. Also add thefont(size: 25)
andpadding()
modifiers:List { ForEach(self.weatherData){ weather in HStack { Image(systemName: weather.image) .frame(width: 50, alignment: .leading) Text("\(weather.temp)°F") .frame(width: 80, alignment: .leading) Text(weather.city) } .font(.system(size: 25)) .padding() } }
The resulting preview should be as follows:
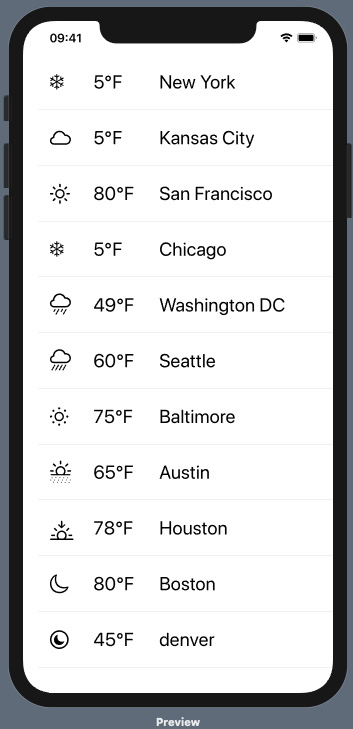
Figure 2.2 – List in the weather app
How it works…
We started the recipe by creating a WeatherInfo
struct to hold and model our weather data. The id = UUID()
property creates a unique identifier for each variable we create from the struct. Adding the id
property to our struct allows us to later use it within a ForEach
loop without providing id: .\self
parameter as seen in the previous recipe.
The ForEach
loop within the List
view iterates through the items in our model data and copies one item at a time to the weather variable declared on the same line.
The ForEach
loop contains HStack
because we would like to display multiple items on the same line – in this case, all the information contained in the weather
variable.
The font(.system(size: 25))
and padding()
modifiers add some padding to the list rows to improve the design and readability of the information.
The images displayed are based on SF Symbols, explained in Chapter 1, Using the Basic SwiftUI Views and Controls.