Code generation
Yii2 provides the powerful module Gii to generate models, controllers, and views, which you can easily modify and customize. It's a really helpful tool for fast and quick development.
In this section we will explore how to use Gii and generate code. For example you have a database with one table named film
and you would like to create an application with CRUD operations for this table. It's easy.
Getting ready
- Create a new application by using composer as described in the official guide at http://www.yiiframework.com/doc-2.0/guide-start-installation.html.
- Download the Sakila database from http://dev.mysql.com/doc/index-other.html.
- Execute the downloaded SQLs: first the schema then the data.
- Configure the database connection in
config/main.php
to use the Sakila database. - Run your web-server by
./yii serve
.
How to do it…
- Go to
http://localhost:8080/index.php?r=gii
and select Model Generator. - Fill out Table Name as
actor
and Model Class asActor
and press button Generate at the bottom of page. - Return tothe main Gii menu by clicking the yii code generator logo on the header and choose CRUD Generator.
- Fill out the Model Class field as
app\models\Actor
and Controller Class asapp\controllers\ActorController
. - Press the Preview button at the bottom of page and then press green button Generate.
- Check the result via
http://localhost:8080/index.php?actor/create
.
How it works…
If you check your project structure you will see autogenerated code:
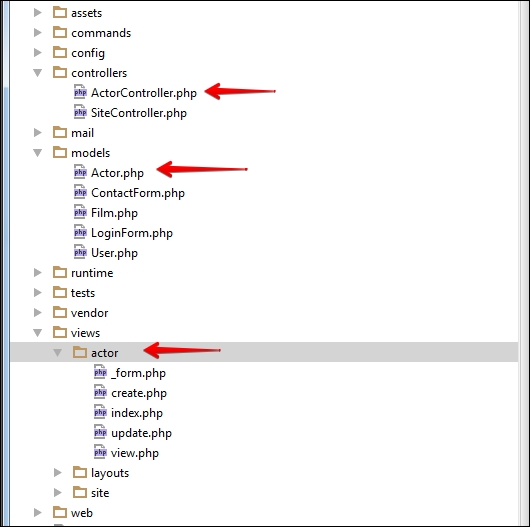
Firstly we've created an Actor
model. Gii automatically creates all model rules which depends on mysql
field types. For example, if in your MySQL actor
table's fields first_name
and last_name
have IS NOT NULL
flag then Yii automatically creates rule for it required
and sets max length 45
symbols because in our database max length of this field is set up as 45
.
public function rules() { return [ [['first_name', 'last_name'], 'required'], [['last_update'], 'safe'], [['first_name', 'last_name'], 'string', 'max' => 45], ]; }
Also Yii creates relationship between models automatically, based on foreign keys you added to your database. In our case two relations were created automatically.
public function getFilmActors() { return $this->hasMany(FilmActor::className(), ['actor_id' => 'actor_id']); } public function getFilms() { return $this->hasMany(Film::className(), ['film_id' => 'film_id'])->viaTable('film_actor', ['actor_id' => 'actor_id']); }
This relationship has been created because we have two foreign keys in our database. The film_actor
table has foreign key fk_film_actor_actor
which points to actor
table fields actor_id
and fk_film_actor_film
which points to film
table field film_id
.
Notice that you haven't generated FilmActor
model yet. So if you would develop full-app versus demo you had to generate Film
, FilmActor
models also. For the rest of the pieces, refer to http://www.yiiframework.com/doc-2.0/guide-start-gii.html.