Basic If statements may look like this:
- If (X == 50) (assuming 0x0001000 represents the X variable):
mov eax, 50
cmp dword ptr [00010000h],eax
- If (X | 00001000b) (| represents the OR logical gate):
mov eax, 000001000b
test dword ptr [00010000h],eax
In order to understand the branching and flow redirection, let's take a look at the following diagram to see how it's manifested in pseudocode:
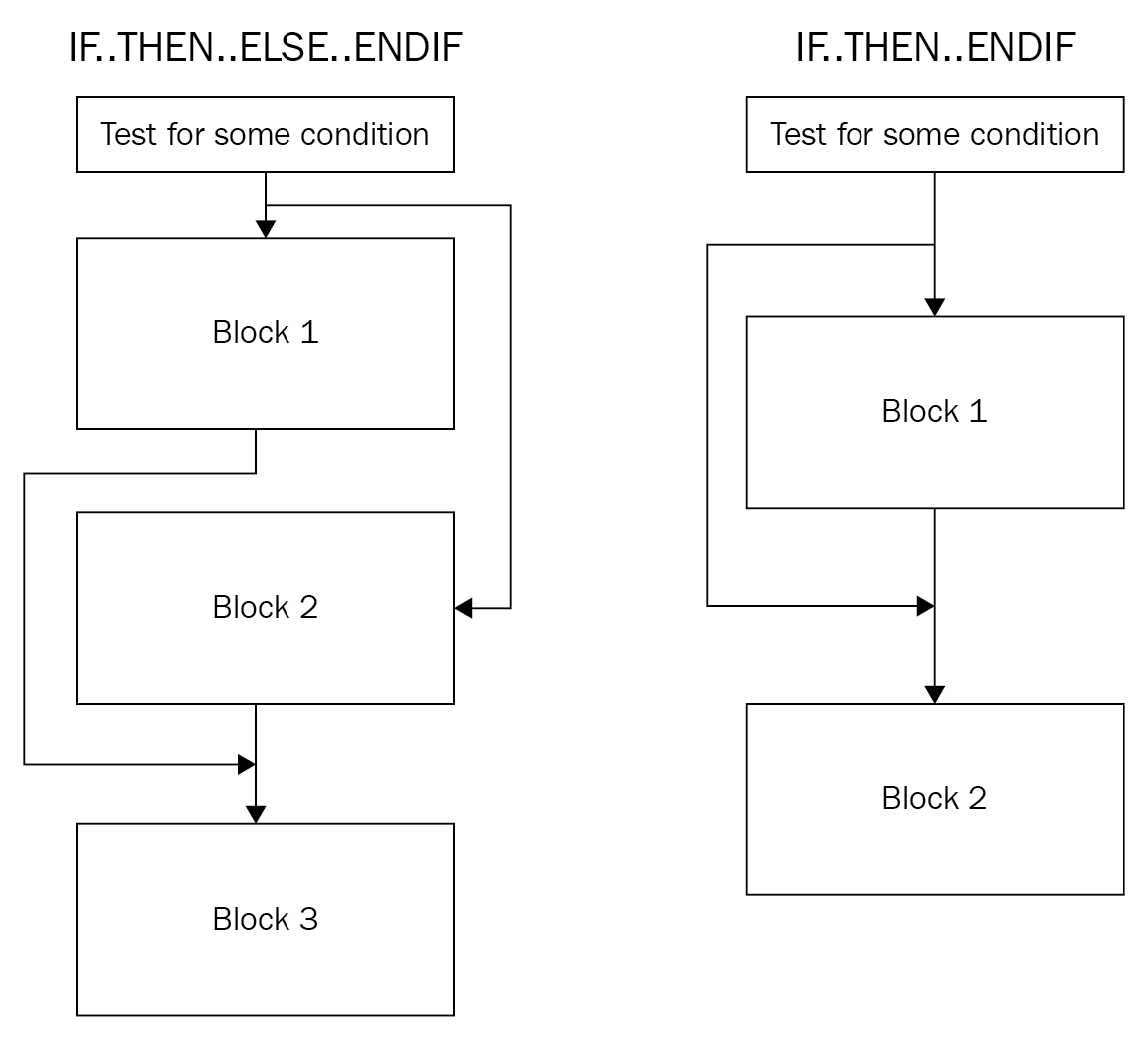
Figure 7: Conditional flow redirection
To apply this branching sequence in assembly, the compiler uses a mix of conditional and unconditional jumps, as follows:
- IF.. THEN.. ENDIF:
cmp dword ptr [00010000h],50
jnz 3rd_Block ; if not true
…
Some Code
…
3rd_Block:
Some code
- IF.. THEN.. ELSE.. ENDIF:
cmp dword ptr [00010000h],50
jnz Else_Block ; if not true
...
Some code
...
jmp 4th_Block ;Jump after Else
Else_Block:
...
Some code
...
4th_Block:
...
Some code