Build a 12-hour clock functor
We'll build a 12-hour clock functor like this one:
Structure | A clock with 12 places for the hours |
Transformation operation | f(x) = x + 12, where x is the hour |
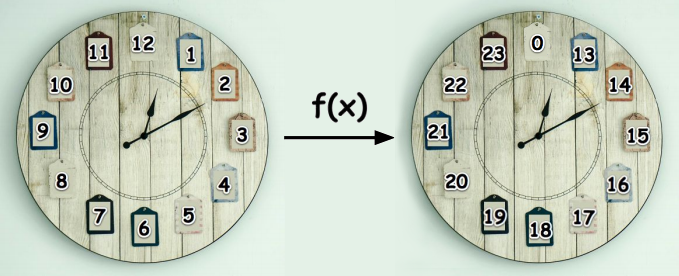
First, let’s examine the functor implementation:
// src/functor/clock.go package functor import ( "fmt" )
Define our ClockFunctor
interface to include a single function (Map
):
type ClockFunctor interface { Map(f func(int) int) ClockFunctor }
Create a container to hold our list of 12 hours:
type hourContainer struct { hours []int }
When called, Map
will be executed/applied to each element in the container:
func (box hourContainer) Map(f func(int) int) ClockFunctor { for i, el := range box.hours { box.hours[i] = f(el) } return box }
It's okay for the implementation of Map
to be impure, as long as the side effects are limited to variables, such as the loop variables, scoped to the Map
function. Notice that return the container, that we call box
, whose elements have been transformed in some way by the mapper...