Building a simple program with OpenCV
There are many program samples that show how to use OpenCV using Python. In our case, we build a simple program to detect a circle in a still image.
Consider we have the following image, which is used for testing. You can find the image file in the source code files, called circle.png
.
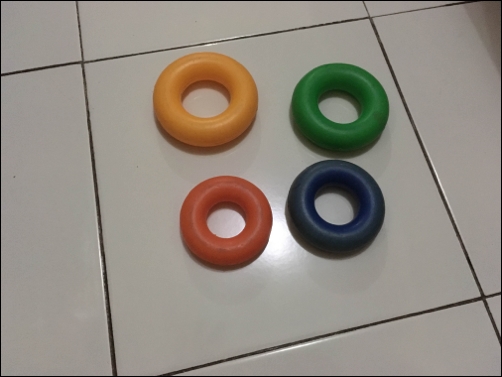
To find a circle in a still image, we use circle Hough Transform (CHT). A circle can be defined as follows:

(a,b) is the center of a circle with radius r. These parameters will be computed using the CHT method.
Let's build a demo!
We will build a program to read an image file. Then, we will detect a circle form in an image using the cv2.HoughCircles()
function.
Let's start to write these scripts:
import cv2 import numpy as np print('load image') orig = cv2.imread('circle.png') processed = cv2.imread('circle.png', 0) processed = cv2.medianBlur(processed, 19) print('processing...') circles = cv2.HoughCircles(processed, cv2.HOUGH_GRADIENT, 1, 70, param1=30, ...