It's the job of the TodoList component to render the todo list items. When the GET_USER query takes place, the TodoList component needs to be able to render all the todo items. Here's a look at the item list again, with several more todos added:
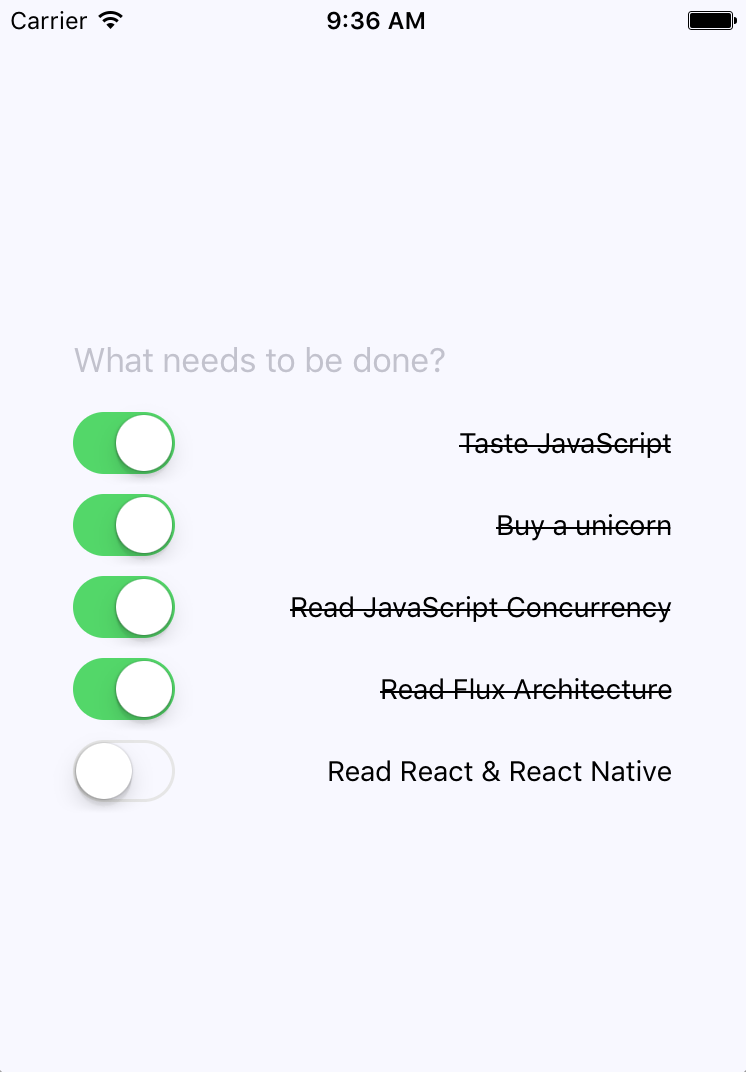
Here's the TodoList component itself:
import React, { Component } from 'react';
import { View } from 'react-native';
import Todo from './Todo';
class TodoList extends Component {
render() {
const { user } = this.props;
return (
<View>
{user.todos.map(todo => (
<Todo key={todo.id} todo={todo} />
))}
</View>
);
}
}
export default TodoList
The relevant GraphQL query to get the data you need for this component is already executed in the App component. This component, therefore, doesn't need to send a query to the GraphQL backend itself and can render the todos that were passed to it. When you render the <Todo...