Scala collections
In Scala, collections are automatically mutable
or immutable
depending on your usage. All collections in scala.collections.immutable
are immutable
. And vice-versa for scala.collections.immutable
. Scala picks immutable
collections by default, so your code will then draw automatically from the mutable
collections, as in:
var List mylist;
Or, you can prefix your variable with immutable
:
var mylist immutable.List;
We can see this in this short example:
var mutableList = List(1, 2, 3); var immutableList = scala.collection.immutable.List(4, 5, 6); mutableList.updated(1,400); immutableList.updated(1,700);
As we can see in this Notebook:
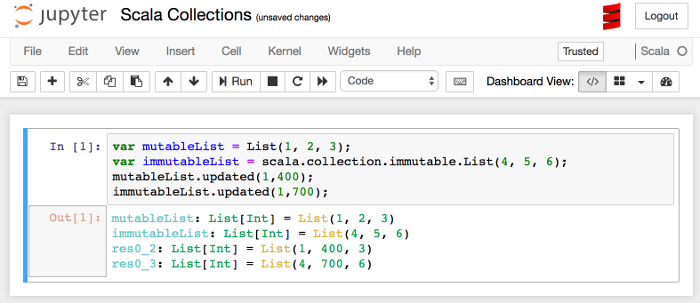
Note that Scala cheated a little here: it created a new collection
when we updated immutableList
, as you can see with the variable name real_3
instead.