Moving the player character
Cool, after that detour to vector math, let’s continue working on our game. The first thing we’ll start out with is player movement.
Changing the current player node
Godot has a physics engine baked into it. To utilize it, we will have to use the physics nodes provided by Godot itself. The player is currently a Node2D, but we actually want it to be a CharacterBody2D.
Luckily, it is very easy to change the type of a node. To do so, follow these steps:
- Right-click on the
Player
node. - Select Change Type as shown in the following figure:
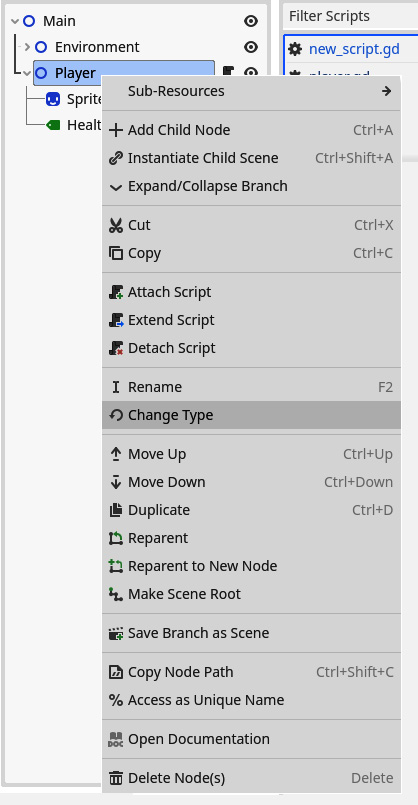
Figure 7.12 – Node type can be changed through the menu that pops up when right-clicking that node
- Search for CharacterBody2D as follows:
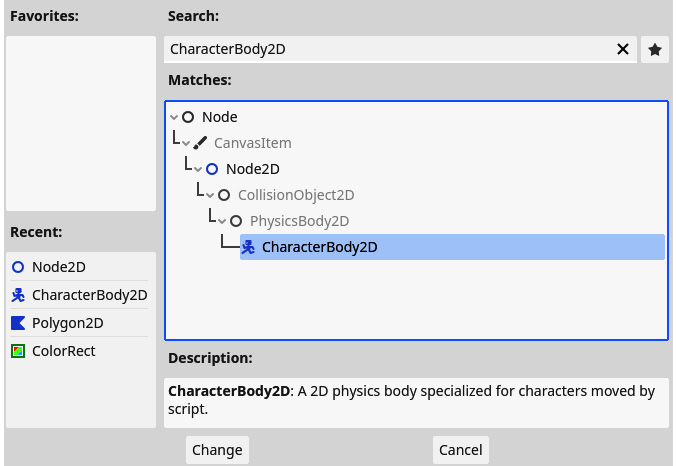
Figure 7.13 – Search for the CharacterBody2D node type
- Select the CharacterBody2D node and you will see the icon for the
Player
node change to reflect our selection...