Strict versus lazy
Consider the operation of zipping up two lists. The zip
method pairs elements from the first list with the elements from the second list. Here is a sample run:
scala> List(1,2,3).zip(List(4,5,6,7)) res8: List[(Int, Int)] = List((1,4), (2,5), (3,6)) scala> List(1,2,3).zip(Nil) res9: List[(Int, Nothing)] = List()
All the elements of both the lists are visited to create a zipped list. The following figure shows the zip
operation in action:
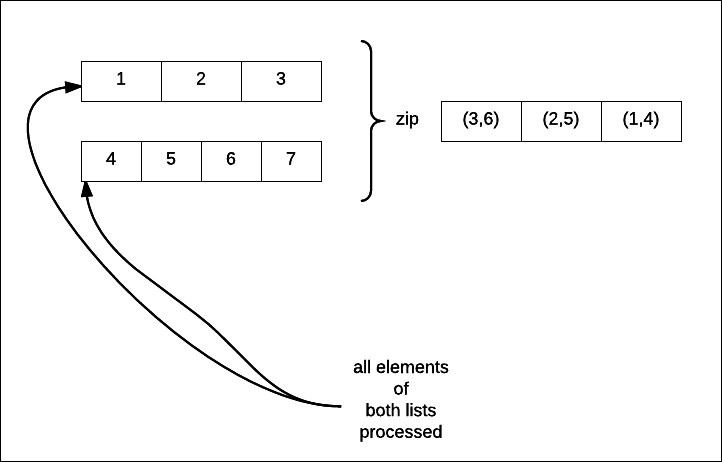
As another example of strict evaluation, consider the reverse
method of List
:
scala> List(1,2,3).reverse res11: List[Int] = List(3, 2, 1)
The reverse
method also visits all the elements of the list. On the other hand, consider the following:
scala> val q = List.range(1, 1000000).view.reverse q: scala.collection.SeqView[Int,List[Int]] = SeqViewR(...) scala> (q take 10) foreach println 999999 999998 999997 ...
Only the first 10 elements of the lazy lists are computed on demand.