Variables in JavaScript
Now that we have built a Hello World program, let us take the next step and perform a few arithmetic operations on two numbers.
Note
The semi colon (;
) is a statement terminator, it tellsthe JavaScript engine that a statement has ended.
Let us take a look at another program,
alert_script.html
:
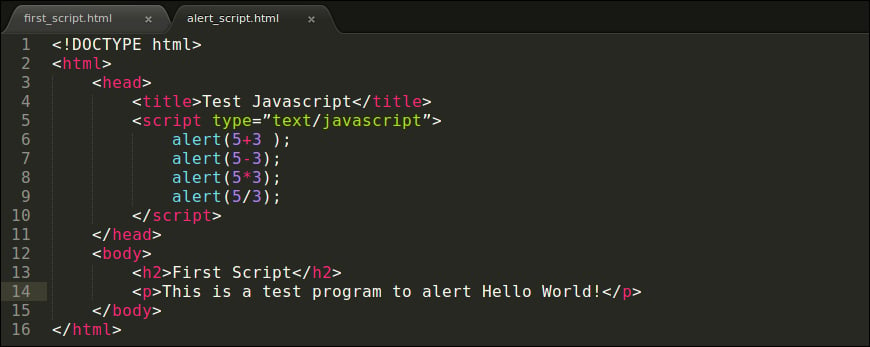
The previous program would run and produce four pop-up windows, one after the other, displaying their respective values. A glaring problem here is that we are repetitively using the same numbers in multiple places. If we had to perform these arithmetic operations on a different set of numbers, we would have had to replace them at multiple locations. To avoid this situation, we would assign those numbers to temporary storage locations; these storage locations are often referred to as variables.
The keyword var
is used to declare a variable in JavaScript, followed by the name of that variable. The name is then implicitly provided with a piece of computer memory, which we will use throughout the program execution. Let us take a quick look at how variables will make the earlier program more flexible:
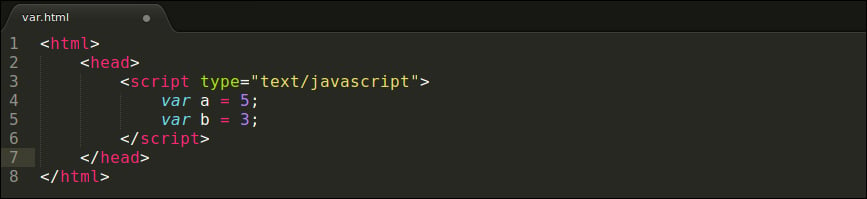
Note
Code commenting can be done in two ways: one is single line, and the other is multiline.
Single line comments:
//This program would alert the sum of 5 and 3; alert(5+3);
Multiline comments:
/* This program would generate two alerts, the first alert would display the sum of 5 and 3, and the second alert would display the difference of 5 and 3 */ alert(5+3); alert(5-3);
Let us continue with the program:
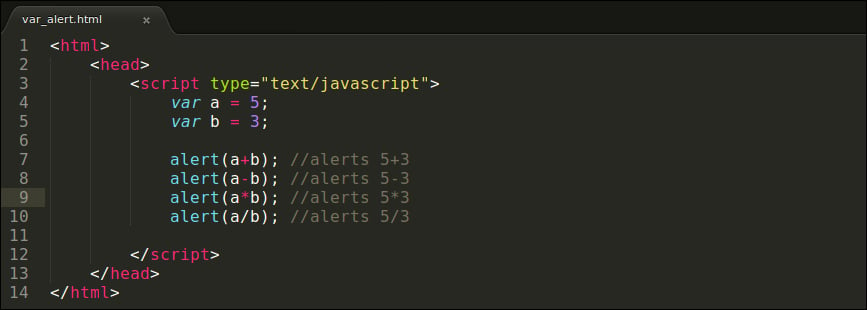
Now let us alter the value from 5
to 6
; the amount of change that we will make here is minimal. We assign the value of 6
to our variable a
, and that takes care of the rest of the process; unlike our earlier script where changes were made in multiple locations. This is shown as follows:
Note
Code commenting is a recurring and an extremely important step in the development life cycle of any application. It has to be used to explain any assumptions and/or any dependencies that our code contains.
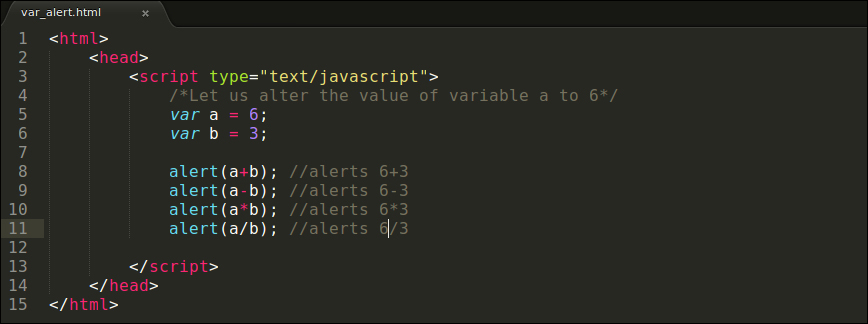
In JavaScript, we declare a variable by using the keyword var
and until a value is assigned to it, the value of the variable will be implicitly set to undefined
; that value is overwritten on variable initialization.