1.7 Loops
If I ask you to close your eyes, count to 10, then open them, the steps look like this:
- Close your eyes
- Set
count
to 1 - While
count
is not equal 10, incrementcount
by 1 - Open your eyes
Steps 2 and 3 together constitute a loop. In this loop, we repeatedly do something while a condition is met. We do not move to step 4 from step 3 while the test returns true.
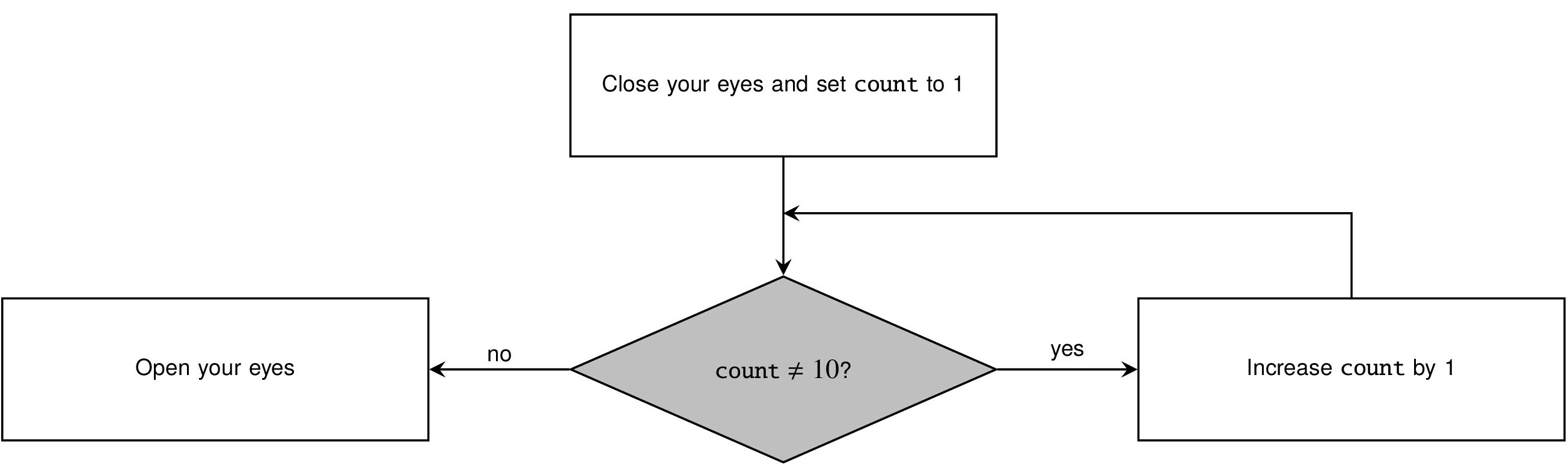
Compare the simplicity of Figure 1.3 to
- Close your eyes
- Set
count
to 1 - Increment
count
to 2 - Increment
count
to 3 - …
- Increment
count
to 10 - Open your eyes
If that doesn’t convince you, imagine if I asked you to count to 200. Here is how we do it in C++:
int n = 1;
while( n < 201 ) {
n++;
}
Exercise 1.6
Create a similar while-loop flowchart for counting backward from 100 to 1.
That loop was a while-loop, but this is an until-loop:
- Close your eyes
- Set
count
to 1 - Increment
count
by 1 untilcount
equals 10 - Open your eyes
Many languages do not have until-loops, but VBA does:
n = 1
Do Until n>200
n = n + 1
Loop
We saw earlier that the code within a function’s definition is its body. The repeated code in a loop is also called its body.
Exercise 1.7
What is different between while-loops and until-loops regarding when you test the condition? Compare this until-loop flowchart with the previous while-loop example.
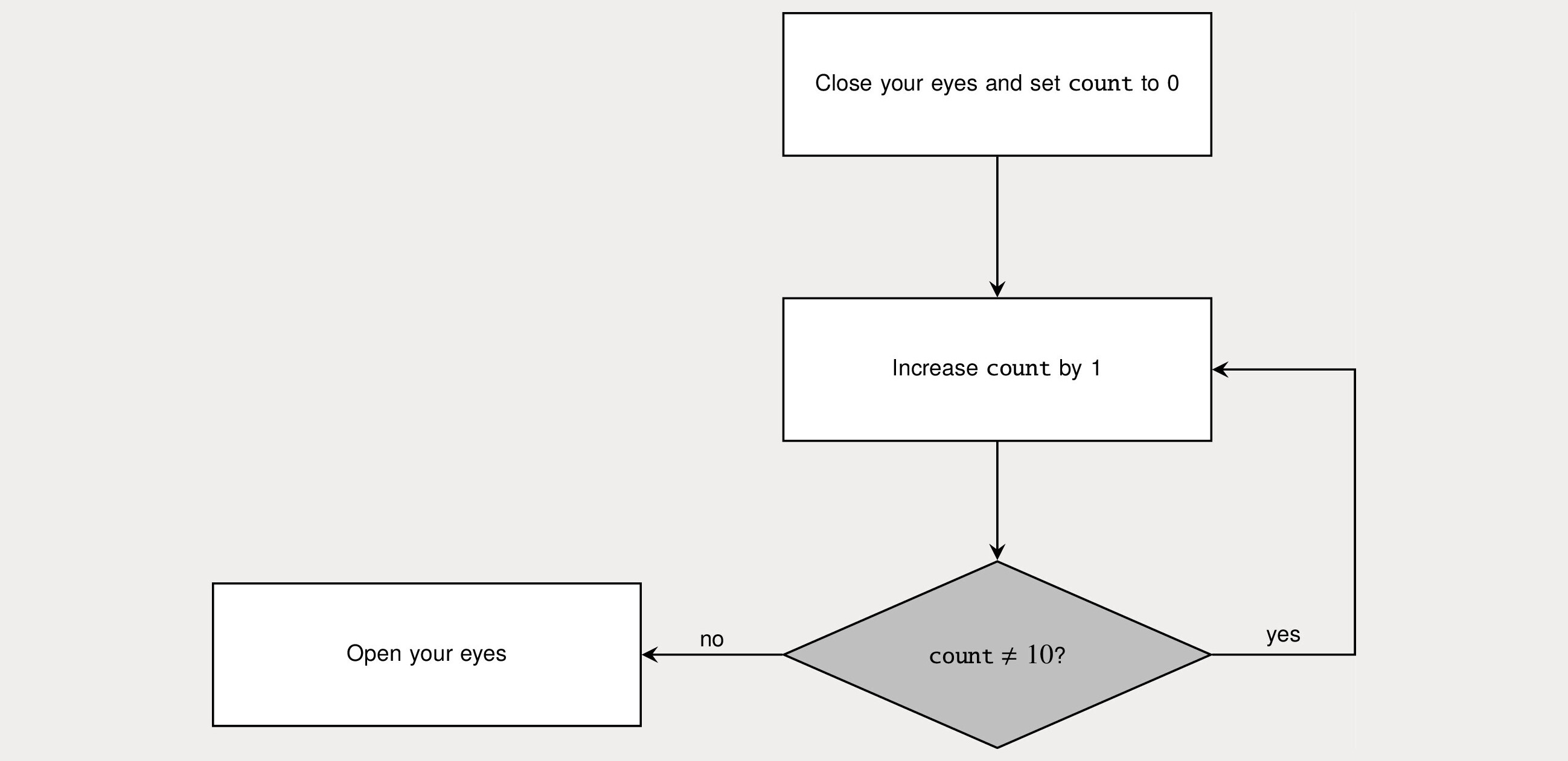
Our next example is a for-loop, so named because of the keyword that many programming languages use. A for-loop is very useful when you want to repeat something a specified number of times. This example uses the Go language:
sum := 0
for n := 1; n <= 50; n++ {
sum += n
}
It adds all the numbers between 1 and 50, storing the result in
sum
. Here, :=
is an assignment, n++
means “replace the value of n
by its previous value plus 1,” and sum +=
n
means “replace the value of sum
by its previous value plus the value of
n
.”
There are four parts to this particular syntax for the for-loop:
- the initialization:
n := 1
- the condition:
n <= 50
- the post-body processing code:
n++
- the body:
sum += n
The sequence is: do the initialization once; test the condition and, if true, execute the body; execute the post-body code; test the condition again and repeat. If the condition is ever false, the loop stops, and we move to whatever code follows the loop.
Exercise 1.8
What are the initialization, condition, post-body processing code, and body of the “count to 10” example rewritten with a for-loop?
Exercise 1.9
Draw a flowchart of a for-loop, including the initialization, condition, post-body processing code, and the body. Use the template in Figure 1.5.
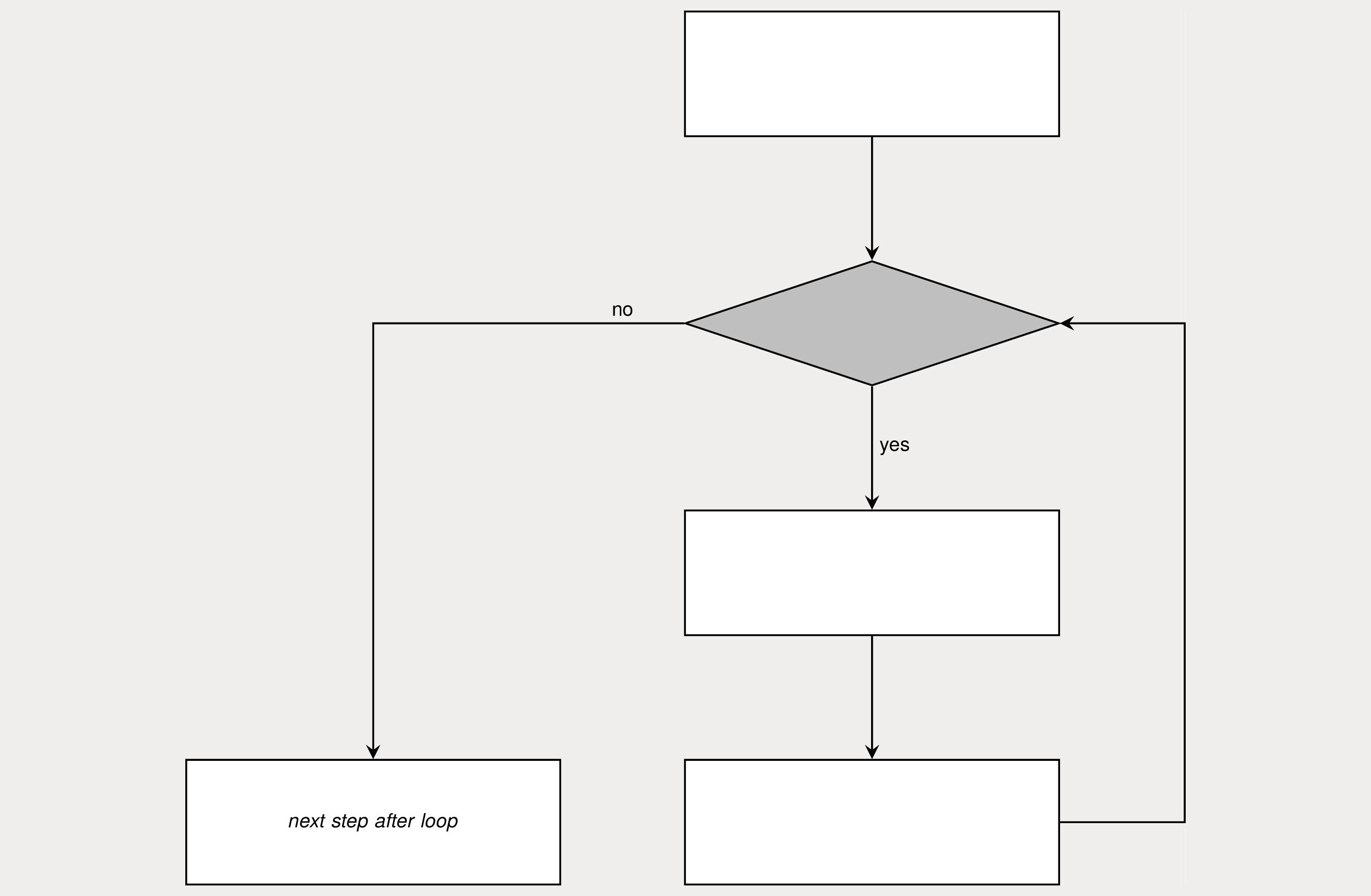