Computing and visualizing histograms
A histogram is a graphical representation of a distribution of data. Basically, it is the graphical depiction of a frequency distribution table. Let me explain this through an example. Suppose we have a dataset such as (1, 2, 1, 3, 4, 1, 2, 3, 4, 4, 2, 3, 4). Here, a frequency distribution looks as follows:
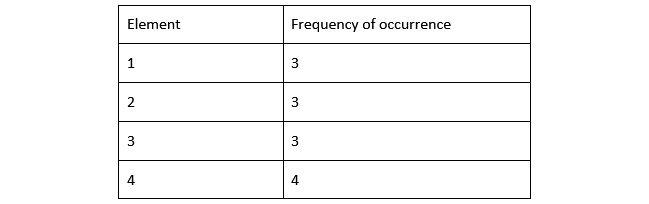
Figure 10.1 – Frequency distribution
If we are to plot a bar graph so that the x axis represents elements and the y axis represents the frequency in which they occur, then this is known as a histogram. We can use np.histogram()
to compute a histogram. plt.hist()
can compute and directly plot it. Let's write some code that will use both functions to interpret the data in the preceding table:
import numpy as np import matplotlib.pyplot as plt a = np.array([1, 2, 1, 3, 4, 1, 2, 3, 4, 4, 2, 3, 4]) hist, bins = np.histogram(a) print(hist) print(bins) plt.hist(a) plt.show()
The output looks as follows...