Scala higher-order functions
A higher-order function either takes other functions as arguments or returns a function as its result.
We can use this example script:
def squared(x: Int): Int = x * x def cubed(x: Int): Int = x * x * x def process(a: Int, processor: Int => Int): Int = {processor(a) } val fiveSquared = process(5, squared) val sevenCubed = process(7, cubed)
We define two functions: one squares the number passed, and the other cubes the number passed.
Next, we define a higher-order function that takes a number to work on and a processor to apply.
Lastly, we call each function. For example, we call process()
with 5
and the squared
function. The process()
function passes 5
to the squared()
function and returns the result:
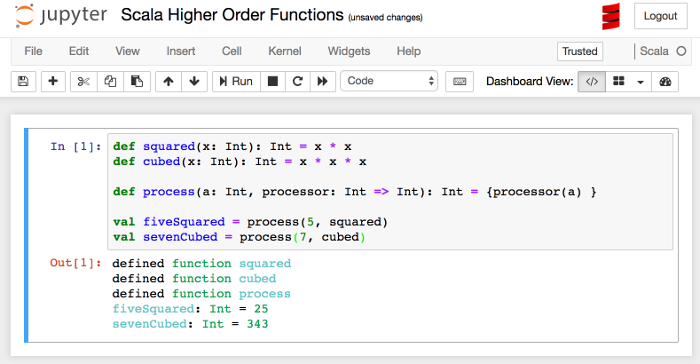
We take advantage of Scala's engine automatically printing out variable values to see the expected result.
These functions are not doing very much. When I ran them, it took a few seconds for the results to display. I think there is a big performance hit using higher...