With authentication and authorization you have already secured all your data, but there are still some things that we need to make sure of security while building your enterprise grade Ionic application. Many things here we will be working around Cordova to secure our application. As security is a deep and complicated topic, the most important thing is to stay updated and try using the latest versions of Cordova and Ionic. Usually, if there are some security vulnerabilities found in Cordova you will soon see a patch for that and it will get fixed.
Securing the Ionic application
Whitelisting
Domain whitelisting is a security model that controls the access to external domains. Cordova provides a configurable security policy to define which sites to access. For Cordova 4.0 and newer, we should use cordova-plugin-whitelist as it provides better security and configuration settings. Currently cordova-whitelist-plugin supports Android higher than 4 and for our platforms we have to configure security in the config.xml file: <access origin="*" />
While creating a project, by default all the domains are whitelisted, which means you will have access to all domains. Also, domain whitelisting is not available on and below Android API 10. So this is another important thing to note that you set your min-target-sdk above 10. Also, Ionic itself supports above Android version 4.4 . It will work on old devices and sdk, but it may have performance and other issues. Also, we don't have a big market share of devices with less than Android 4.4. So for building applications for the future we can ignore some share of market to make your application secure and good in performance. With new technologies coming, such as the BLE support application, even many native applications are only supporting latest versions.
We have already covered the whitelist plugin in Chapter 3, Ionic Native and Plugins so you already have an idea of how we can easily configure various setting and whitelist resources. The new content security policy in the plugin gives you control over which network requests (images, XHRs, and so on) are allowed to be made (via web view directly):
<!-- Allow everything but only from the same origin
and foo.com -->
<meta http-equiv="Content-Security-Policy" con-
tent="default-src 'self' foo.com">
<!-- Allows XHRs only over HTTPS on the same domain. -->
<meta http-equiv="Content-Security-Policy" con-
tent="default-src 'self' https:">
<!-- Allow iframe to https://cordova.apache.org/ -->
<meta http-equiv="Content-Security-Policy" con-
tent="default-src 'self'; frame-src 'self'
https://cordova.apache.org">
You can find various other examples of configurations such as the previous ones in the official documentation of the whitelist plugin: https://cordova.apache.org/docs/en/latest/reference/cordova-plugin-whitelist/.
Enabling SSL
This is one of the most important points if you are transferring secure data over networks to servers, before uploading your application to various stores you should make it production ready and enable SSL for all the secure requests. As most of the Ionic applications use REST APIs to get or post data to servers, we should make sure that all of our secure requests are HTTPS. At the same time, if your application doesn't have any critical data then you can use HTTP also for this case.
Using self-signed certificates for production applications is not recommended. If you want to introduce an SSL, make sure that your server has a certificate properly signed by a well-known certificate authority. The reason is that accepting self-signed certificates bypasses the certificate chain validation, which allows any server certificate to be considered valid by the device. This opens up the communication to man-in-the-middle attacks. The data will be encrypted, but man-in-middle can decrypt it by the key by middleman. You can look at it as a normal web application where you always make sure that all the secure information is sent in encrypted form to the server so that no one can hack it in the middle. To make sure of this it is very important that you buy an SSL certificate from a well-known certificate authority.
Sensitive data outside the app
In our application, there might be some external APIs that we are using for fetching third- party content into our application. For this, you will get some kind of secret_keys specific to your account, which will be used when you hit their servers. Here we have to make sure that we don't place any of these keys inside your Ionic application as someone can easily extract that from your code base.
Here, the best way is to make a proxy request on your server or a custom request on your server-side code that will add the keys to the APIs calls. So you will be calling your server and then your server will pass a request to a third-party service with secret_key attached to the new request. In response the same response can be passed back to the client via a server from the third-party service:
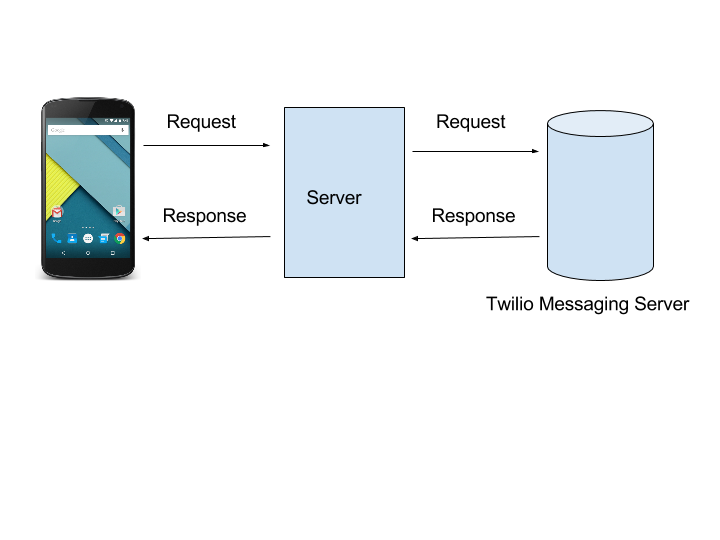
If, for example you have user specific keys then you have to ask the user to first login inside you application, which will then validate the user and the next API call from your server can get the respective user_keys, which can then be used for further communication with their third-party server. Also, make sure that you don't have any business logic inside you application, which you can easily handle on the server side. Don't assume that your source is secure as Ionic applications that are Cordova-based are built from HTML and JavaScript assets packaged in a native container. Anyone can reverse engineer to get into your code, so that is why we don't ever place any sensitive information inside code.
Similarly, for BAAS services such as Ionic Cloud and Firebase, you have to place the keys on the client side for directly calling these platform APIs. Although, if we have proper access control and have protected the application with auth and login protected, then only authenticated users can make request to the APIs. This will secure it from hackers to exhaust your number of API calls for your account.
Secure storage
In Ionic application, many developers heavily depends upon localStorage or SQLite via Cordova plugin. Many times we have to store secret such as usernames, passwords, tokens, certificates, or any other sensitive information, In this case we can use secure storage Native plugin which uses keychain to encrypt data in iOS and in Android this plugin encrypts data to store it in SharedPreference. Inside an Ionic application, localStorage sandboxes the data only for your app. No, other application can access the data from outside, In case you are using SQLite as you application database, you can take advantage of SQLcipher plugin for encrypting the data inside SQLite:
$ ionic cordova:plugin add cordova-plugin-secure-storage
$ npm install --save @ionic-native/secure-storage
Let’s see one such example can be storing user’s mobile number:
import { SecureStorage, SecureStorageObject } from '@ionic-native/secure-storage';
constructor(private secureStorage: SecureStorage) { }
this.secureStorage.create('user_data')
.then((storage: SecureStorageObject) => {
storage.get('mobile_number')
.then(
data => console.log(data),
error => console.log(error)
);
storage.set('mobile_number', '+917813123123')
.then(
data => console.log(data),
error => console.log(error)
);
storage.remove('mobile_number')
.then(
data => console.log(data),
error => console.log(error)
);
});
Again, for all you are doing for encrypting the data we have to take are that we should never save passwords and other critical information inside application storage. Even in native applications it is not recommended because if someone is dedicated enough, they can still decrypt your data.
General security points
Most mobile operating systems provide hardware level encryption. This gives the devices extra protection when the device is locked. So we have to make sure that we always use the latest sdks and Ionic 2 only supports Android 4.4 and higher versions. Other than this there are some secure storage plugins for iOS and Android, which use keychain in case of iOS and for Android AES encryption. Another plugin is PrivacyScreenPlugin, which can be used for hiding sensitive information to be shown in app switchers in case of Android and iOS. Users will see splash screen in task switcher and if it is not detected it will show a black screen.
Reverse engineering is another concern of many developers who think that their application code can easily be extracted and repackaged in the application with some malicious code and uploaded back onto the Play Store. Although this same practice can be done in the case of Native applications, we can bypass this security concern in case of Ionic applications by downloading JavaScript at runtime and deleting it when the app closes. Although we need to think if we really require this as this will come with performance issues also. On the other hand, it's very difficult to do this in Java or objective-C and store your code base dynamically.
Obfuscation, minification, and compression are some of the ways you can secure your code within our Ionic application. We can use many open-source tools to minify and uglify your source before you package them to various app stores. Some popular tools are YUI compressor and UglifyJS, which can be used to uglify your JavaScript resources. Also, you should always minify all your JavaScript and CSS resources, which will improve the performance of your Ionic application and lower your APK file size. Some enterprise tools are also available such as Jscrambler, which does all kinds of minification and obfuscation using its proprietary solutions.
The following are some quick points that you can look into before you release your application for security context:
- Any sensitive business logic should be executed on the server
- Obfuscation and minification your JavaScript assets with uglify or use Jscrambler
- Do not use Android OS less than 4.0
- Use InAppBrowser for external links
- Validate user inputs for HTML forms
- Don't cache sensitive data
- Don't use eval() unless you know what you are doing
- Don't assume your source code is always secure
- Try to use keychain plugin for iOS
It's important to note that security is a critical topic and one should always be updated with new improvements in Cordova so we can improve our application in our next app release to app stores. Also, make sure that you don't lose any performance.