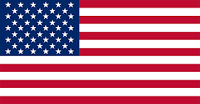

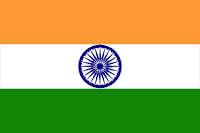
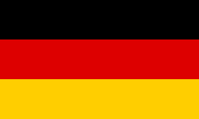
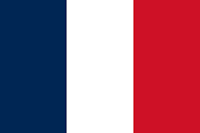

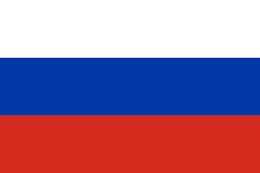
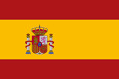



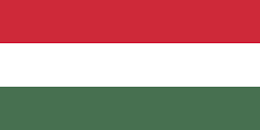
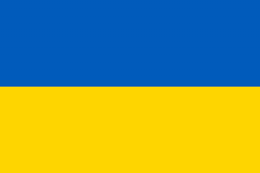
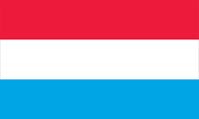

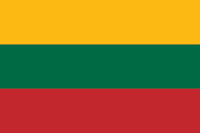

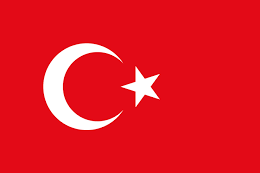


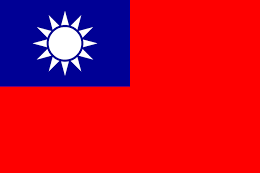
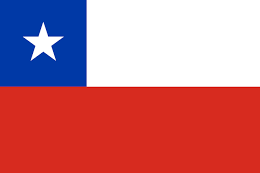

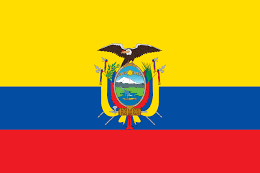
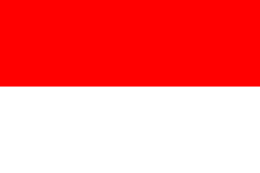
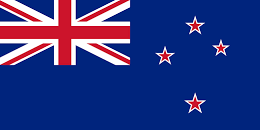
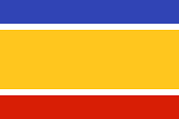
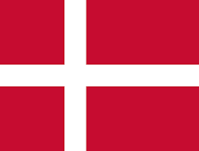
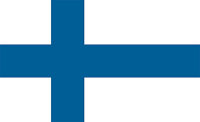


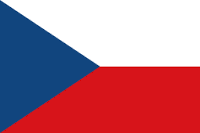
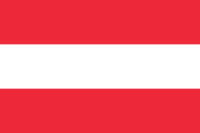
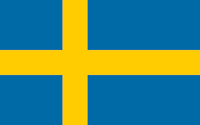
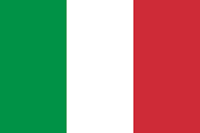
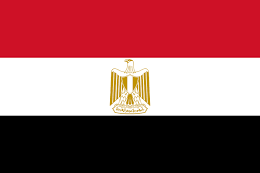

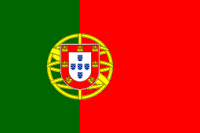
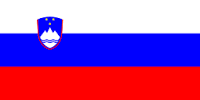

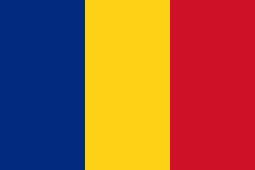
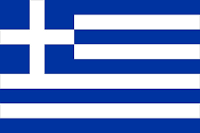


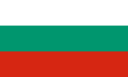
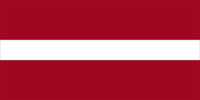
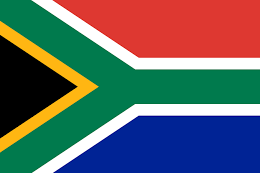
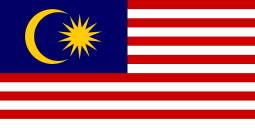



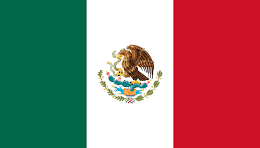
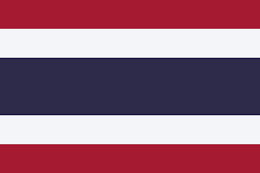
Hi ,
Welcome to this week’s edition of ProgrammingPro!
In today’sExpert Insight, we bring you an excerpt from the recently published book, Functional Programming with C#, which discusses the implementation of Nullable Reference Types (NRTs) in C#, as a tool for developers to handle null references more safely and transparently
News Highlights: OSI releases latest draft of Open Source AI Definition; Microsoft releases Prompty, a new VS Code extension for integrating LLMs into .NET development; JetBrains Workspaces offers monorepo benefits exclusively in IntelliJ IDEA; and Survey shows game engines like Godot gaining traction in non-game projects.
My top 5 picks from today’s learning resources:
But there’s more, so dive right in.
Stay Awesome!
Divya Anne Selvaraj
Editor-in-Chief
PS:We have finished covering all requests made by you so far through the August survey. We did receive some specific and comprehensive requests around Java and are therefore planning to take out a Collector's Edition on Java sometime next month. If you have any more Java specific requests you would like covered in the special issue, reply to this email and let me know.
pdb
, faulthandler
, cProfile
, and tracemalloc
.Result
, dynamic errors like Box<dyn Error>
, and structured errors. Read to also learn about downcasting errors, using the anyhow
crate.Here’s an excerpt from “Chapter 4: Honest Functions, Null, and Option” in the book, Functional Programming with C# by Alex Yagur, published in July 2024.
Handling null in C# has always
been quite a challenge. Many software developers (me included) advocate doing a check for NullReferenceException
s as a mandatory task in a code review checklist. In most cases, it is really easy to check for possible null values just by looking at the pull request, even without an IDE. Recently, we received help when Microsoft introduced nullable reference types. So, now, the compiler will join us in the search of possible disasters causedby null.
What are nullable reference types?
In the simplest terms,Nullable Reference Types(orNRTsfor short) are a feature in C# that allows developers to clearly indicate whether a reference type can be null or not. With this, C# gives us a tool to make our intentions clear right from the start. Think of it as a signpost, guiding other developers (and even our future selves) about what to expect fromour code.
Without NRTs, every reference type in C# could potentially benull
. This would create a guessing game. Is this variable going to have a value or is it going to benull
? Now, with NRTs, we don’t have to guess anymore. The code itself tellsthe story.
Let’s look at a basic example to graspthe concept:
string notNullable = "Hello, World!";
string? nullable = null;
In the preceding snippet, thenotNullable
variable is a regular string that can’t be assignednull
(if you try, the compiler will warn you). On the other hand, since C# 8.0, nullable is explicitly marked with?
, indicating that it canbenull
.
In some cases, you might want to assignnull
to a variable that is not marked as nullable. In this case, to suppress warnings, you can use the!
sign to let the compiler know that you are aware of what you are doing and everything is going according to plan:
string notNullable = "Hello, World!";
notNullable = null!;
One of the biggest advantages of NRTs is that the C# compiler will warn you if you’re potentially doing something risky with null values. It’s like having a friendly guide always looking over your shoulder, ensuring you don’t fall into the common traps ofnull misuse.
For instance, if you try to access properties or methods on a nullable reference without checking fornull
, the compiler will give you a heads-up.
For those with existing C# projects, you might be wondering:Will my project be littered with warnings if I enable NRTs?The answer is no. By default, NRTs are turned off. You can opt into this feature, file by file, allowing for asmooth transition.
NRTs are a good answer to the long-standing challenge posed by null references. By making the potential presence of null explicit in our code, we take a giant leap toward clarity, safety, and functional honesty. In the end, embracing NRTs not only makes ourcode more resilient but also ensures that our intentions, as developers,are transparent.
To enable NRTs, we need to tell the C# compiler that we’re ready for its guidance. This is done using a simple directive:#
nullable enable
.
Place this at the start of your.
cs
file:
#nullable enable
From this point onward in the file, the compiler creates a specific nullable context and assumes that all reference types are non-nullable by default. If you want a type to be nullable, you’ll have to mark it explicitlywith?
.
With NRTs enabled, the C# compiler becomes your safety net, pointing out potential issues with nulls in your code. Whenever you try to assign null to a reference type without the?
marker or when you attempt to access a potentially null variable without checking it, the compiler willwarn you.
Here’san example:
string name = null; // This will trigger a warning
string? maybeName = null; // This is okay
While transitioning a project to use NRTs, there may be sections of your code where you’d prefer to delay the transition. You can turn off NRTs for those specific sections using the #nullable
disable
directive:
#nullable disable
This tells the compiler to revert to the old behavior, treating all reference types aspotentially nullable.
You might wonder why C# chose to use directives for this feature. The reason is flexibility. By using directives, developers can gradually introduce NRTs into their projects, one file or even one section of code at a time. This phased approach makes it easier to adaptexisting projects.
Speaking of a phased approach, there are two more options to set our nullable contexts:warnings
andannotations
. You can use them by writingthe following:
#nullable enable warnings
Or, you canwrite this:
#nullable enable annotations
The main purpose of these options is to ease the migration of your existing code from a fully disabled null context to a fully enabled one. In short, we want to start with thewarnings
option in order to get dereference warnings. When all warnings are fixed, we can switch toannotations
. This option will not give us any warnings, but it will start to treat our variables as non-nullable unless declared with the?
mark.
To get more information about these options and nullable context in generated files, and to find out more about three nullabilities – oblivious, nullable, and non-nullable, I recommend you read the article "Nullable reference types". You might also want to read the article “Update a codebase with nullable reference types to improve null diagnostic warnings”.
Functional Programming with C# was published in July 2024. Packt library subscribers can continue readingthe entire book for free or you can buy the bookhere!
That’s all for today.
We have an entire range of newsletters with focused content for tech pros. Subscribe to the ones you find the most usefulhere. Complete ProgrammingPro archives can be foundhere. Complete PythonPro archives arehere.
If your company is interested in reaching an audience of developers, software engineers, and tech decision makers, you may want toadvertise with us.
If you have any comments or feedback, just respond to this email.