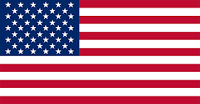

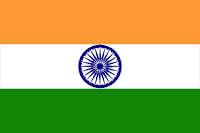
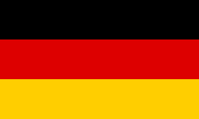
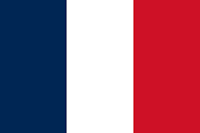

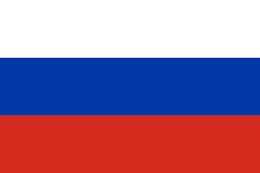
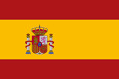



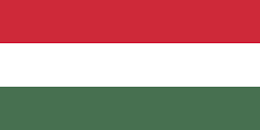

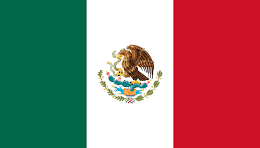
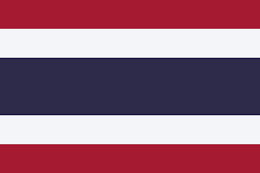
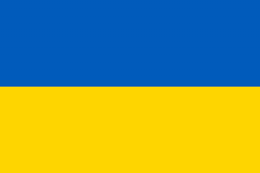
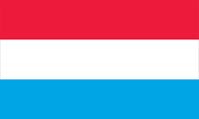

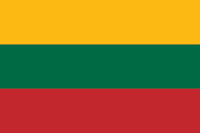

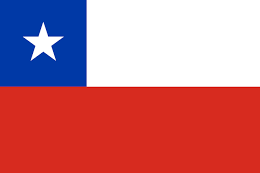

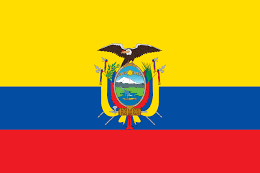

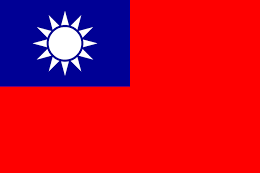

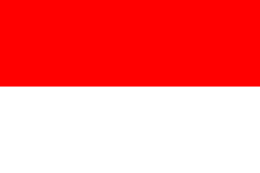
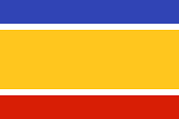
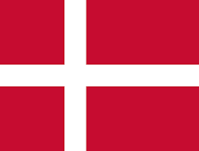
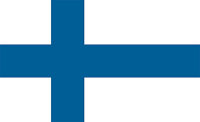


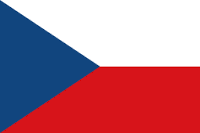
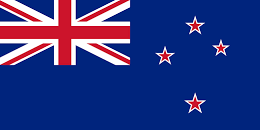
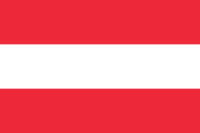
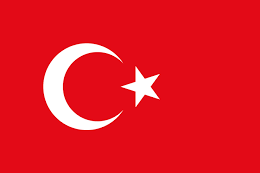
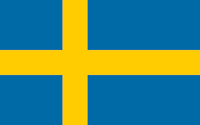
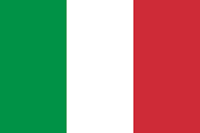
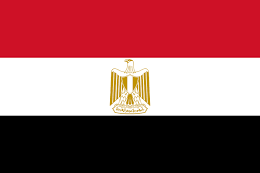

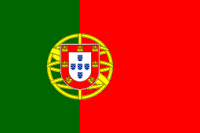
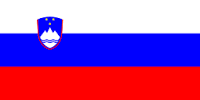

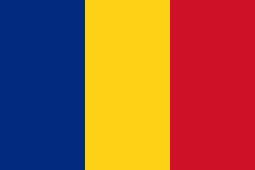
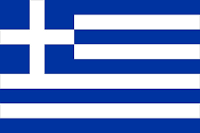

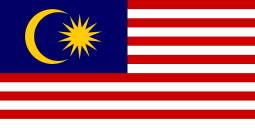
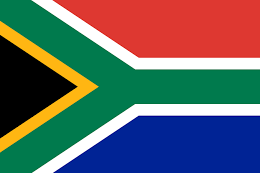

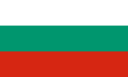
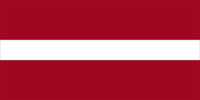


This article is the second part of a series of articles, please refer to Part 1 for learning how to create a simple portfolio website using ChatGPT in a few steps
In my last blog, we created a basic personal portfolio app for Olivia with ChatGPT. We’ll use this as the starting point for our next adventure. We’re going to be adding a simple chatbot to our website and configuring it to deal with certain questions. Of course, we could do this ourselves, but let’s see how we can utilize ChatGPT to accomplish this task faster. We’re going to do so with just four prompts!
Let’s start with the end result, so you know what we’re working towards:
Image 1: End result of the project
In the right bottom corner, you see a pop-up that says Ask me anything. Well, you can’t ask it anything, but it can answer the following common questions:
Of course, we can elaborate and do even more with it. We actually wanted to use a free API for this, and we were stuck with CDN options due to the nature of our project. Luckily, we have a digital friend who has some options for us. Let’s see how this started.
It’s best to be quite descriptive when prompting. This is the first prompt that was used to start implementing the chatbot:
I have an existing portfolio site, using HTML/CSS/JS. I'm not using any package managers, so any requirements will have to use a CDN. I want to add a chatbot to my site using a Natural Language AI. If it is possible I'd want the API I'll use to be free. Can you give me some options as to how I can achieve this?
ChatGPT had some ideas:
Certainly! There are several options available for adding a chatbot with Natural Language AI to your existing portfolio site using HTML/CSS/JS and integrating it through a CDN. Here are a few options that offer free plans:
1. IBM Watson Assistant: IBM Watson Assistant is a powerful NLP service that allows you to build chat bots. You can integrate it into your website using the Watson Assistant API. IBM offers a free tier that provides a limited number of API requests per month. You can find more information and documentation on the IBM Watson Assistant website.
2. Dialogflow (formerly API.AI): Dialogflow, now part of Google Cloud, offers a free tier that allows you to create conversational agents and integrate them into your website. Dialogflow provides a JavaScript library that you can use to communicate with the API. You can find documentation and examples on the Dialogflow website.
3. Wit.ai: Wit.ai, which is also owned by Facebook, provides a free NLP service for building chatbots. You can integrate it into your website using the Wit.ai API. Wit.ai offers a JavaScript SDK that you can use to interact with the API. You can find more information on the Wit.ai website.
4. Microsoft Azure Bot Service: Microsoft Azure Bot Service offers a free tier that allows you to create and deploy chatbots. You can use the Bot Service REST API to communicate with your bot from your website. Microsoft provides documentation and examples on its Azure Bot Service website.
Remember to review the terms and limitations of the free plans for each service to ensure they meet your requirements. Additionally, be aware that some services may have usage limitations or require you to upgrade to a paid plan for higher usage levels or advanced features.
Choose the option that best suits your needs, and refer to the respective documentation for implementation details specific to each service.
After this, it was research time. This actually took a little while. IBM wasn’t properly explored, because the sign-up failed due to the Dutch valid phone number not passing validation. Azure seemed great, but a bit too advanced for what we needed right now. The chatbot service chosen was Wit.ai. On there we can sign up, get an access token and specify how to deal with certain inputs.
After figuring that out and making a little code start ourselves. It was time to start adjusting the code with ChatGPT.
Enter the following prompt, to begin with:
Alright, I set up a wit.ai project, and it seems to work. Now I have the following snippet:
<section id="chat-bot">
<h2>Ask me anything!</h2>
<input id="chat-prompt" type="text">
</section>
I'd like to create a chatbot with this. I can write the responses myself, but can you for now create some JavaScript code that sends an HTTP request to the wit.ai server and parses the result? For now, just print it to the console.
It gave us a great place to start and some additional implementation guidelines. We’ve only included the code snippet here:
// Function to send the user's message to Wit.ai and parse the response
async function sendMessage(message) {
// Replace 'YOUR_WITAI_ACCESS_TOKEN' with your actual Wit.ai access token
const accessToken = 'YOUR_WITAI_ACCESS_TOKEN';
const apiUrl = `https://api.wit.ai/message?v=20210525&q=${encodeURIComponent(message)}`;
try {
const response = await fetch(apiUrl, {
headers: {
Authorization: `Bearer ${accessToken}`
}
});
if (response.ok) {
const data = await response.json();
console.log('Wit.ai Response:', data);
// Process the data and handle the response as needed
// For example, extract intents, entities, or generate appropriate responses
} else {
console.log('Request to Wit.ai failed with status:', response.status);
}
} catch (error) {
console.log('An error occurred while communicating with Wit.ai:', error);
}
}
// Event listener for handling user input
const inputElement = document.getElementById('chat-prompt');
inputElement.addEventListener('keydown', async (event) => {
if (event.key === 'Enter') {
const message = event.target.value.trim();
if (message) {
console.log('User Input:', message);
await sendMessage(message);
event.target.value = ''; // Clear the input field
}
}
});
It also instructed us to take the following steps:
YOUR_WITAI_ACCESS_TOKEN
with our actual access token<script>
tagThis is what it looked like:
Image 2: practical output after the first few prompts
This was already quite good, but of course, we don’t want the response in the console, but displayed in the chat window. And the same goes for our previous questions, it should become a chat. Let’s see if we can move toward that.
It’s always a good idea to be specific in your prompt. You can see the following prompt does quite a bit of the heavy lifting and explains clearly what type of work is needed from ChatGPT. This helps to achieve the result:
I've modified the HTML a little bit:
<section id="chat-bot">
<ol id="chat-history"></ol>
<h2>Ask me anything!</h2>
<input id="chat-prompt" type="text">
</section>
Can you write some logic which can populate the `chat-history` element? I need 3 things:
- A history as a JavaScript object
- A function which can generate the content from the history
- A function which can add the question, and the response, to the history in JavaScript
For now just print the top intent and all the entities.
This did quite a bit of the work already. You can see the result below, the history and the answers of the two questions asked appear on top of the input box.
Image 3: Answers and history appearing on top of the Input box
It’s no secret that I’m not a UX or design expert, but even I can see that this is not ideal. So let’s see if we can improve the design.
Let’s see if we can improve the design. Normally chatbots are not on the top left, but the bottom right. Also, it would be nice if the history could go inside the box. Of course, we want to use the same orange as for the rest of our portfolio. Here’s the prompt:
Can you generate some CSS to style the element? I'd like the following:
- The chat should be at the bottom right of the page.
- The chat should not impact the rest of the page.
- Each question and response should have a chat bubble.
- The theme color is #f37041.
This gave us the CSS and some context as output. Here’s the CSS:
#chat-bot {
position: fixed;
bottom: 20px;
right: 20px;
z-index: 9999;
background-color: #f37041;
color: #fff;
font-family: Arial, sans-serif;
border-radius: 5px;
padding: 10px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.3);
}
#chat-history {
list-style: none;
margin: 0;
padding: 0;
max-height: 200px;
overflow-y: auto;
}
.question,
.response {
display: inline-block;
max-width: 80%;
padding: 8px;
border-radius: 10px;
margin-bottom: 5px;
}
.question {
background-color: #fff;
color: #333;
text-align: right;
}
.response {
background-color: #f37041;
color: #fff;
text-align: left;
}
#chat-prompt {
width: 100%;
padding: 8px;
margin-top: 10px;
border: none;
border-radius: 5px;
}
#chat-prompt:focus {
outline: none;
}
#chat-prompt::placeholder {
color: #ccc;
}
And this did quite the trick. Here’s what it looks like now:
Image4: The final output
If you’d like to see all the responses and the versions of the personal portfolio after each step, you can check out this GitHub repo: https://github.com/BrightBoost/portfolio-with-ai-prompt-chatgpt
In conclusion, integrating a basic chatbot into your personal portfolio website can significantly enhance user engagement and provide a unique interactive experience. By leveraging GPT prompts, you can effortlessly develop a conversational AI agent that understands and responds to user queries. Adding this functionality not only showcases your technical skills but also demonstrates your ability to create dynamic and user-centric web experiences. So go ahead, implement a chatbot and elevate your portfolio website to the next level.
Happy prompting!
Maaike van Putten is an experienced software developer and Pluralsight, LinkedIn Learning, Udemy, and Bright Boost instructor. She has a passion for software development and helping others get to the next level in their career.