OpenCV offers a handy function called Canny (after the algorithm's inventor, John F. Canny), which is very popular not only because of its effectiveness, but also because of the simplicity of its implementation in an OpenCV program since it is a one-liner:
import cv2
import numpy as np
img = cv2.imread("../images/statue_small.jpg", 0)
cv2.imwrite("canny.jpg", cv2.Canny(img, 200, 300)) # Canny in one line!
cv2.imshow("canny", cv2.imread("canny.jpg"))
cv2.waitKey()
cv2.destroyAllWindows()
The result is a very clear identification of the edges:
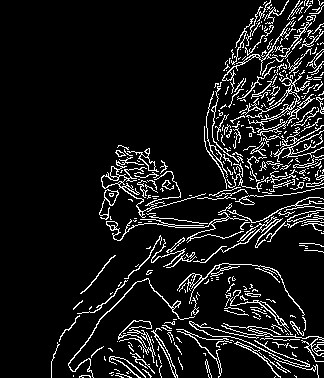
The Canny edge detection algorithm is complex but also quite interesting. It is a five-step process:
- Denoise the image with a Gaussian filter.
- Calculate the gradients.
- Apply non-maximum suppression (NMS) on the edges. Basically, this means that the algorithm selects the...