Binary tree
A binary tree is a tree that has a maximum of two children per node. The two children can be called the left and the right child of a node. The following figure shows an example of a binary tree:
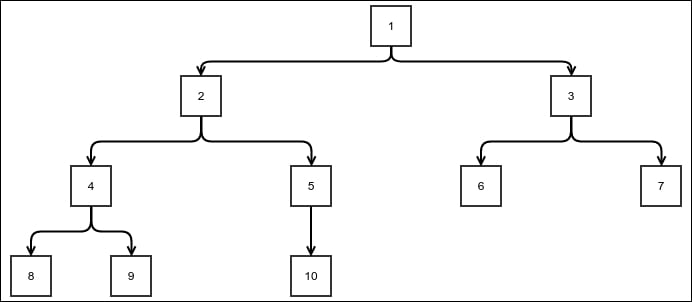
Example binary tree
This particular tree is important mostly because of its simplicity. We can create a BinaryTree
class by inheriting the general tree class. However, it will be difficult to stop someone from adding more than two nodes and will take a lot of code just to perform the checks. So, instead, we will create a BinaryTree
class from scratch:
public class BinaryTree<E> {
The Node
has a very obvious implementation just like the generic tree:
public static class Node<E>{ private E value; private Node<E> left; private Node<E> right; private Node<E> parent; private BinaryTree<E> containerTree; protected Node(Node<E> parent, BinaryTree<E> containerTree, E value) { ...