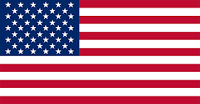

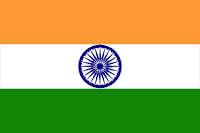
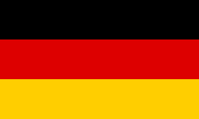
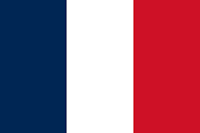

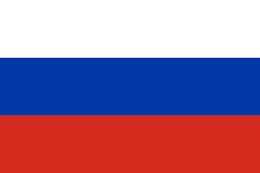
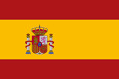



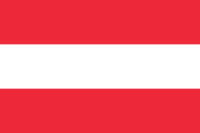

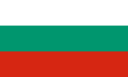
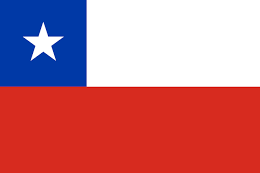

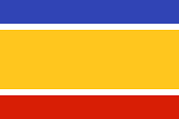
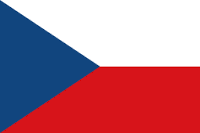
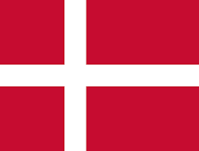
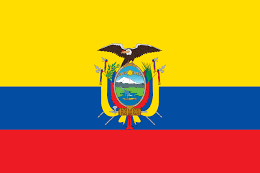
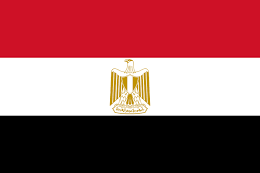

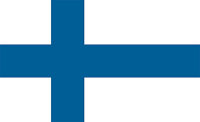
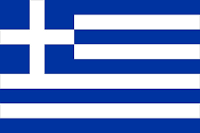
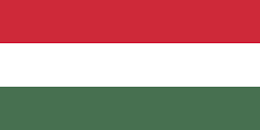
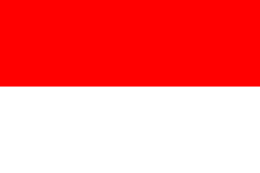

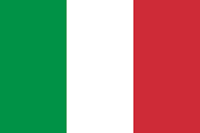

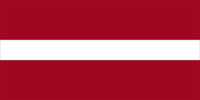
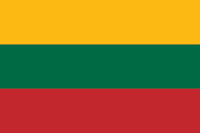
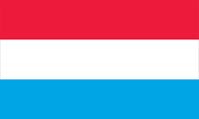
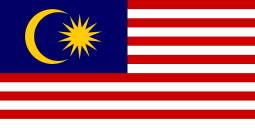

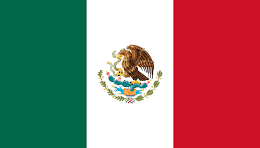

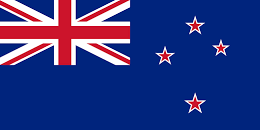



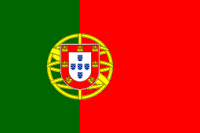
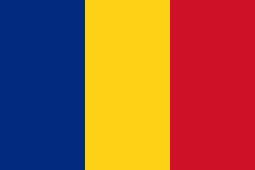


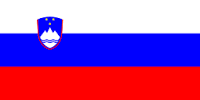
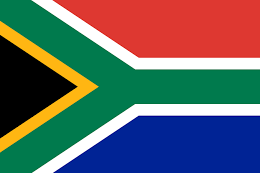

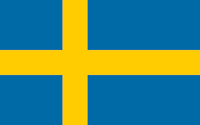

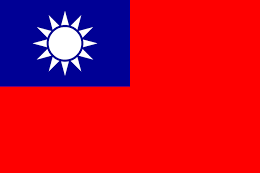
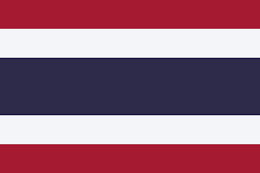
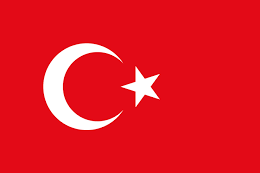
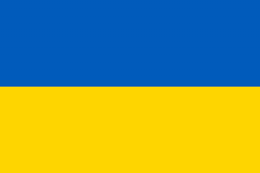
In this tutorial, we will get started with using the Entity Framework and create a simple console application to perform CRUD operations. The intent is to get started with EF Core and understand how to use it. Before we dive into coding, let us see the two development approaches that EF Core supports:
These two paradigms have been supported for a very long time and therefore we will just look at them at a very high level. EF Core mainly targets the code-first approach and has limited support for the database-first approach, as there is no support for the visual designer or wizard for the database model out of the box. However, there are third-party tools and extensions that support this. The list of third-party tools and extensions can be seen at https://docs.microsoft.com/en-us/ef/core/extensions/.
This tutorial has been extracted from the book .NET Core 2.0 By Example, by Rishabh Verma and Neha Shrivastava.
In the code-first approach, we first write the code; that is, we first create the domain model classes and then, using these classes, EF Core APIs create the database and tables, using migration based on the convention and configuration provided. We will look at conventions and configurations a little later in this section. The following diagram illustrates the code-first approach:
In the database-first approach, as the name suggests, we have an existing database or we create a database first and then use EF Core APIs to create the domain and context classes. As mentioned, currently EF Core has limited support for it due to a lack of tooling. So, our preference will be for the code-first approach throughout our examples. The reader can discover the third-party tools mentioned previously to learn more about the EF Core database-first approach as well. The following image illustrates the database-first approach:
Now that we understand the approaches and know that we will be using the code-first approach, let's dive into coding our getting started with EF Core console app. Before we do so, we need to have SQL Express installed in our development machine. If SQL Express is not installed, download the SQL Express 2017 edition from https://www.microsoft.com/en-IN/sql-server/sql-server-downloads and run the setup wizard. We will do the Basic installation of SQL Express 2017 for our learning purposes, as shown in the following screenshot:
Our objective is to learn how to use EF Core and so we will not do anything fancy in our console app. We will just do simple Create Read Update Delete (CRUD) operations of a simple class called Person, as defined here:
public class Person { public int Id { get; set; } public string Name { get; set; } public bool Gender { get; set; } public DateTime DateOfBirth { get; set; } public int Age { get { var age = DateTime.Now.Year - this.DateOfBirth.Year;
if (DateTime.Now.DayOfYear <
this.DateOfBirth.DayOfYear)
{
age = age - 1;
}
return age;
}
}
}
As we can see in the preceding code, the class has simple properties. To perform the CRUD operations on this class, let's create a console app by performing the following steps:
The complete and comprehensive list can be seen at https://docs.microsoft.com/en-us/ef/core/providers/. We will be working with SQL Server on Windows for our learning purposes, so let's install the SQL Server package for Entity Framework Core. To do so, let's install the Microsoft.EntityFrameworkCore.SqlServer package from the NuGet Package Manager in Visual Studio 2017. Right-click on the project. Select Manage Nuget Packages and then search for Microsoft.EntityFrameworkCore.SqlServer. Select the matching result and click Install:
public class Context : DbContext { public DbSet<Person> Persons { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder
optionsBuilder)
{
//// Get the connection string from configuration
optionsBuilder.UseSqlServer(@"Server=.\SQLEXPRESS
;Database=PersonDatabase;Trusted_Connection=True;");
}
protected override void OnModelCreating(ModelBuilder
modelBuilder)
{
modelBuilder.Entity<Person>
().Property(nameof(Person.Name)).IsRequired();
}
}
The class looks quite simple, but it has the following subtle and important things to make note of:
[Required] public string Name { get; set; }
C# data type
|
SQL server data type |
int | int |
string | nvarchar(Max) |
decimal | decimal(18,2) |
float | real |
byte[] | varbinary(Max) |
datetime | datetime |
bool | bit |
byte | tinyint |
short | smallint |
long | bigint |
double | float |
There are many other conventions, and we can define custom conventions as well. For more details, please read the official Microsoft documentation at https://docs.microsoft.com/en-us/ef/core/modeling/.
This will open the Package Manager Console window. Select the Default Project as GettingStartedWithEFCore and type the following command:
add-migration CreatePersonDatabase
If you are not using Visual Studio 2017 and you are dependent on .NET Core CLI tooling, you can use the following command:
dotnet ef migrations add CreatePersonDatabase
Your startup project 'GettingStartedWithEFCore' doesn't reference Microsoft.EntityFrameworkCore.Design. This package is required for the Entity Framework Core Tools to work. Ensure your startup project is correct, install the package, and try again.
So let's first go to the NuGet Package Manager and install this package. After successful installation of this package, if we run the preceding command again, we should be able to run the migrations successfully. It will also tell us the command to undo the migration by displaying the message To undo this action, use Remove-Migration. We should see the new files added in the Solution Explorer in the Migrations folder, as shown in the following screenshot:
8. Although we have migrations applied, we have still not created a database. To create the database, we need to run the following commands.
In Visual Studio 2017:
update-database –verbose
In .NET Core CLI:
dotnet ef database update
If all goes well, we should have the database created with the Persons table (property of type DbSet<Person>) in the database. Let's validate the table and database by using SQL Server Management Studio (SSMS). If SSMS is not installed in your machine, you can also use Visual Studio 2017 to view the database and table.
This is the first migration to create a database. Whenever we add or update the model classes or configurations, we need to sync the database with the model using the add-migration and update-database commands. With this, we have our model class ready and the corresponding database created. The following image summarizes how the properties have been mapped from the C# class to the database table columns:
Now, we will use the Context class to perform CRUD operations.
class Program { static void Main(string[] args) { Console.WriteLine("Getting started with EF Core"); Console.WriteLine("We will do CRUD operations on Person class."); //// Lets create an instance of Person class.
Person person = new Person()
{
Name = "Rishabh Verma",
Gender = true, //// For demo true= Male, false =
Female. Prefer enum in real cases.
DateOfBirth = new DateTime(2000, 10, 23)
};
using (var context = new Context())
{
//// Context has strongly typed property named Persons
which referes to Persons table.
//// It has methods Add, Find, Update, Remove to
perform CRUD among many others.
//// Use AddRange to add multiple persons in once.
//// Complete set of APIs can be seen by using F12 on
the Persons property below in Visual Studio IDE.
var personData = context.Persons.Add(person);
//// Though we have done Add, nothing has actually
happened in database. All changes are in context
only.
//// We need to call save changes, to persist these
changes in the database.
context.SaveChanges();
//// Notice above that Id is Primary Key (PK) and hence
has not been specified in the person object passed
to context.
//// So, to know the created Id, we can use the below
Id
int createdId = personData.Entity.Id;
//// If all goes well, person data should be persisted
in the database.
//// Use proper exception handling to discover
unhandled exception if any. Not showing here for
simplicity and brevity. createdId variable would
now hold the id of created person.
//// READ BEGINS
Person readData = context.Persons.Where(j => j.Id ==
createdId).FirstOrDefault();
//// We have the data of person where Id == createdId,
i.e. details of Rishabh Verma.
//// Lets update the person data all together just for
demonstarting update functionality.
//// UPDATE BEGINS
person.Name = "Neha Shrivastava";
person.Gender = false;
person.DateOfBirth = new DateTime(2000, 6, 15);
person.Id = createdId; //// For update cases, we need
this to be specified.
//// Update the person in context.
context.Persons.Update(person);
//// Save the updates.
context.SaveChanges();
//// DELETE the person object.
context.Remove(readData);
context.SaveChanges();
}
Console.WriteLine("All done. Please press Enter key to
exit...");
Console.ReadLine();
}
}
With this, we have completed our sample app to get started with EF Core. I hope this simple example will set you up to start using EF Core with confidence and encourage you to start exploring it further. The detailed features of EF Core can be learned from the official Microsoft documentation available at https://docs.microsoft.com/en-us/ef/core/.
If you're interested in learning more, head over to this book, .NET Core 2.0 By Example, by Rishabh Verma and Neha Shrivastava.
How to build a chatbot with Microsoft Bot framework
Working with Entity Client and Entity SQL