Resizing an image means changing the dimension or scaling of a given image. Scaling or resizing is done either from the width, height, or both. One of the applications of resizing images is training deep learning models where reduced image sizes can speed up the training. Training a deep learning model is out of the scope of this book. If you are interested, then you can refer to any deep learning book from Packt Publishing. Let's see an example of resizing an image:
# Import cv2 module
import cv2
# Import matplotlib for showing the image
import matplotlib.pyplot as plt
# magic function to render the figure in a notebook
%matplotlib inline
# read image
image = cv2.imread('tajmahal.jpg')
# Convert image color space BGR to RGB
rgb_image=cv2.cvtColor(image,cv2.COLOR_BGR2RGB)
# Display the image
plt.imshow(rgb_image)
This results in the following output:
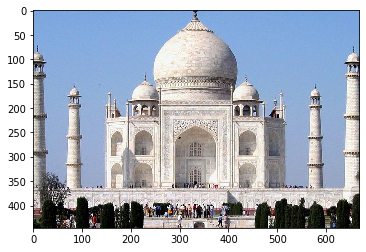
In the preceding code, we read the image and converted it from BGR into the RGB space. Let's resize it now using...