.NET Framework is a software development framework on which we can write a number of languages such as C#, ASP.NET, C++, Python, Visual Basic, and F#.
.NET Framework provides language interoperability across different programming languages. Applications written in .NET Framework execute in an environment or a virtual machine component known as CLR.
The following diagram illustrates the different components in .NET Framework:
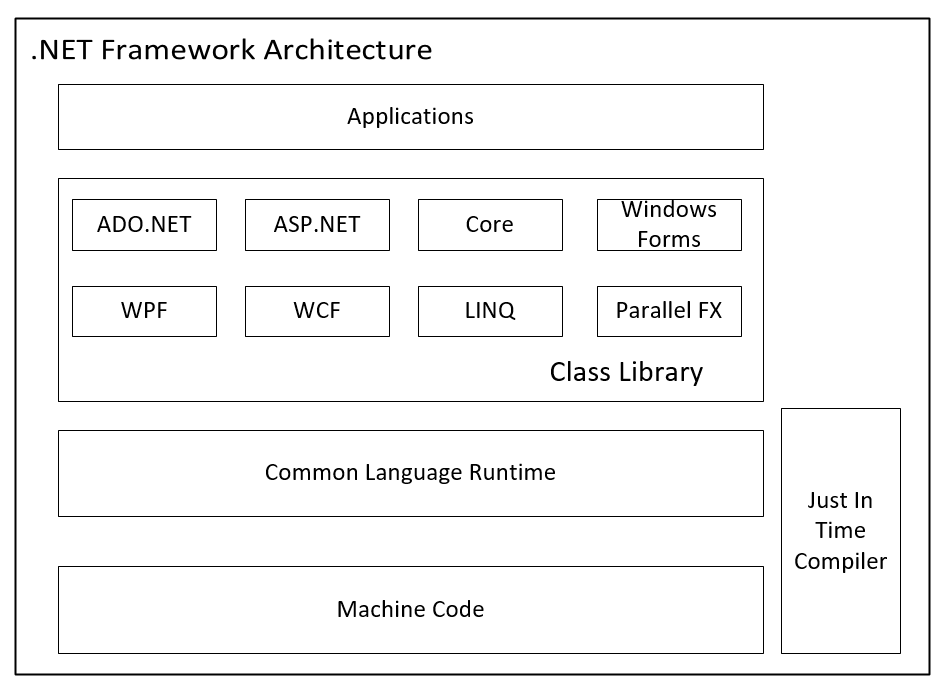
In the previous diagram, note the following:
- At the top of the hierarchy, we have applications or the program code that we write in .NET. It could be as simple as a Hello World console application program, which we will create in this chapter, or as complex as writing multi-threaded applications.
- The applications are based upon a set of classes or design templates, which constitutes a class library.
- The code written in these applications is then acted upon by CLR, which makes use of the Just in Time (JIT) compiler to convert the application code into machine code.
- The machine code is specific to the underlying platform properties. So, for different systems, such as Linux or Windows, it will be different.
In the next section, we will the .NET Framework in detail learn how interact with each other.