The perceptron learning algorithm (PLA) is the following:
Input: Binary class dataset
- Initialize
to zeros, and iteration counter
- While there are any incorrectly classified examples:
- Pick an incorrectly classified example, call it
, whose true label is
- Update
as follows:
- Increase iteration counter,
, and repeat
Return: 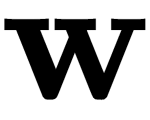
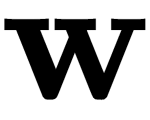
Now, let's see how this takes form in Python.
PLA in Python
Here is an implementation in Python that we will discuss part by part, while some of it has already been discussed:
N = 100 # number of samples to generate
random.seed(a = 7) # add this to achieve for reproducibility
X, y = make_classification(n_samples=N, n_features=2, n_classes=2,
n_informative=2, n_redundant=0, n_repeated=0,
n_clusters_per_class=1, class_sep=1.2,
random_state=5)
y[y==0] = -1
X_train = np.append(np.ones((N,1)), X, 1) # add a column of ones
# initialize the weights to zeros
w...