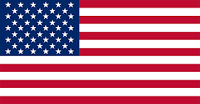

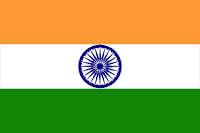
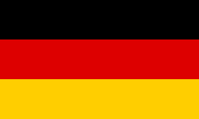
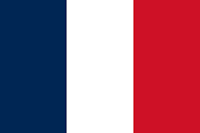

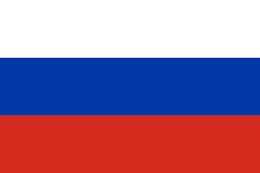
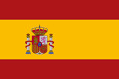



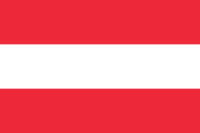

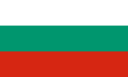
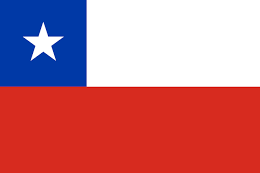

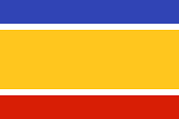
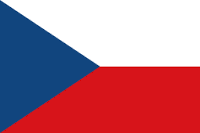
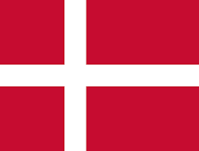
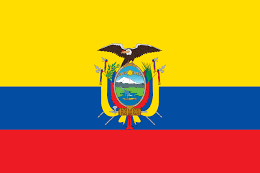
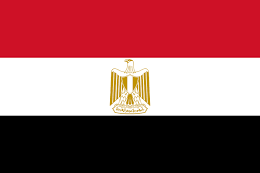

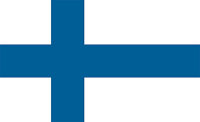
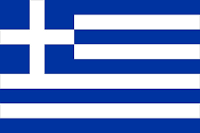
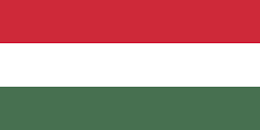
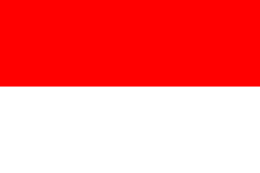

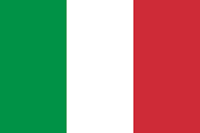

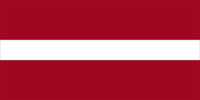
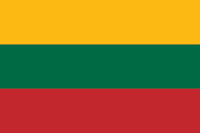
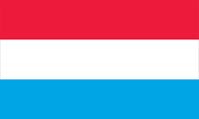
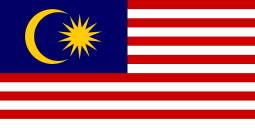

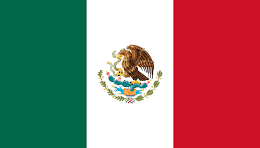

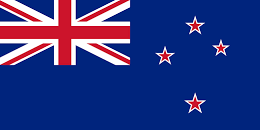



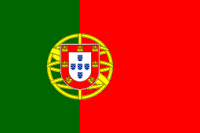
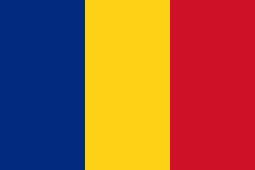


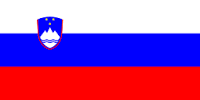
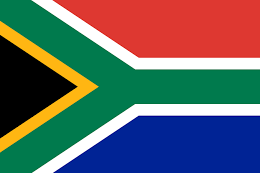

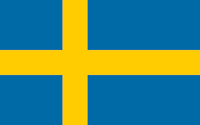

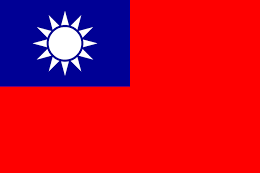
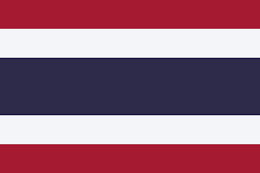
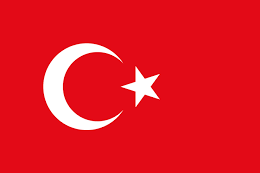
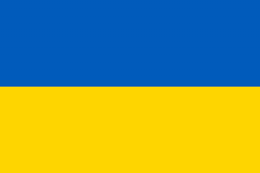
TensorFlow can be integrated into mobile apps for many use cases that involve one or more of the following machine learning tasks:
To run TensorFlow on mobile apps, we need two major ingredients:
The high-level architecture looks like the following figure:
The mobile application code sends the inputs to the TensorFlow binary, which uses the trained model to compute predictions and send the predictions back.
The TensorFlow ecosystem enables it to be used in Android apps through the interface class TensorFlowInferenceInterface, and the TensorFlow Java API in the jar file libandroid_tensorflow_inference_java.jar. You can either use the jar file from the JCenter, download a precompiled jar from ci.tensorflow.org, or build it yourself.
The inference interface has been made available as a JCenter package and can be included in the Android project by adding the following code to the build.gradle file:
allprojects {
repositories {
jcenter()
}
}
dependencies {
compile 'org.tensorflow:tensorflow-android:+'
}
Note : Instead of using the pre-built binaries from the JCenter, you can also build them yourself using Bazel or Cmake by following the instructions at this link: https://github.com/tensorflow/tensorflow/blob/r1.4/ tensorflow/contrib/android/README.md
Once the TF library is configured in your Android project, you can call the TF model with the following four steps:
TensorFlowInferenceInterface inferenceInterface =
new TensorFlowInferenceInterface(assetManager, modelFilename);
inferenceInterface.feed(inputName, floatValues, 1, inputSize, inputSize, 3);
inferenceInterface.run(outputNames, logStats);
inferenceInterface.fetch(outputName, outputs);
In this section, we shall learn about recreating the Android demo app provided by the TensorFlow team in their official repo. The Android demo will install the following four apps on your Android device:
Note: The sample demo only works for Android devices with an API level greater than 21 and the device must have a modern camera that supports FOCUS_MODE_CONTINUOUS_PICTURE. If your device camera does not have this feature supported, then you have to add the path submitted to TensorFlow by the author: https://github.com/ tensorflow/tensorflow/pull/15489/files.
The easiest way to build and deploy the demo app on your device is using Android Studio. To build it this way, follow these steps:
Your screen will look similar to this:
def nativeBuildSystem = 'none'
7. You can also build the apk and install the apk file on the virtual or actual connected device. Once the app installs on the device, you will see the four apps we discussed earlier:
You can also build the whole demo app from the source using Bazel or Cmake by following the instructions at this link: https://github.com/tensorflow/tensorflow/tree/r1.4/tensorflow/examples/android
TensorFlow enables support for iOS apps by following these steps:
target 'Name-Of-Your-Project'
pod 'TensorFlow-experimental'
Note: To create your own TensorFlow binaries for iOS projects, follow the instructions at this link: https://github.com/tensorflow/tensorflow/ tree/master/tensorflow/examples/ios
Once the TF library is configured in your iOS project, you can call the TF model with the following four steps:
PortableReadFileToProto(file_path, &tensorflow_graph);
tensorflow::Status s = session->Create(tensorflow_graph);
std::string input_layer = "input"; std::string output_layer = "output"; std::vector<tensorflow::Tensor> outputs; tensorflow::Status run_status = session->Run(
{{input_layer, image_tensor}},
{output_layer}, {}, &outputs);
tensorflow::Tensor* output = &outputs[0];
In order to build the demo on iOS, you need Xcode 7.3 or later. Follow these steps to build the iOS demo apps:
$ mkdir -p ~/Downloads
$ curl -o ~/Downloads/inception5h.zip https://storage.googleapis.com/download.tensorflow.org/models/incep tion5h.zip
&& unzip ~/Downloads/inception5h.zip -d ~/Downloads/inception5h
$ cp ~/Downloads/inception5h/*
~/tensorflow/tensorflow/examples/ios/benchmark/data/
$ cp ~/Downloads/inception5h/*
~/tensorflow/tensorflow/examples/ios/camera/data/
$ cp ~/Downloads/inception5h/*
~/tensorflow/tensorflow/examples/ios/simple/data/
$ cd ~/tensorflow/tensorflow/examples/ios/camera
$ pod install
$ open tf_simple_example.xcworkspace
TF Lite is the new kid on the block and still in the developer view at the time of writing this book. TF Lite is a very small subset of TensorFlow Mobile and TensorFlow, so the binaries compiled with TF Lite are very small in size and deliver superior performance. Apart from reducing the size of binaries, TensorFlow employs various other techniques, such as:
The workflow for using the models in TF Lite is as follows:
$ freeze_graph
--input_graph=mymodel.pb
--input_checkpoint=mycheckpoint.ckpt
--input_binary=true
--output_graph=frozen_model.pb
--output_node_name=mymodel_nodes
$ toco
--input_file=frozen_model.pb
--input_format=TENSORFLOW_GRAPHDEF
--output_format=TFLITE
--input_type=FLOAT
--input_arrays=input_nodes
--output_arrays=mymodel_nodes
--input_shapes=n,h,w,c
Generally, you would use the graph_transforms:summarize_graph tool to prune the model obtained in step 1. The pruned model will only have the paths that lead from input to output at the time of inference or prediction. Any other nodes and paths that are required only for training or for debugging purposes, such as saving checkpoints, are removed, thus making the size of the final model very small.
The official TensorFlow repository comes with a TF Lite demo that uses a pre-trained mobilenet to classify the input from the device camera in the 1001 categories. The demo app displays the probabilities of the top three categories.
TF Lite Demo on Android
To build a TF Lite demo on Android, follow these steps:
We received the following warnings, but the build succeeded. You may want to resolve the warnings if the build fails:
Warning:The Jack toolchain is deprecated and will not run. To enable support for Java 8 language features built into the plugin, remove 'jackOptions { ... }' from your build.gradle file, and add android.compileOptions.sourceCompatibility 1.8 android.compileOptions.targetCompatibility 1.8
Note: Future versions of the plugin will not support usage 'jackOptions' in build.gradle. To learn more, go to https://d.android.com/r/tools/java-8-support-message.html
Warning:The specified Android SDK Build Tools version (26.0.1) is ignored, as it is below the minimum supported version (26.0.2) for Android Gradle Plugin 3.0.1. Android SDK Build Tools 26.0.2 will be used.
To suppress this warning, remove "buildToolsVersion '26.0.1'" from your build.gradle file, as each version of the Android Gradle Plugin now has a default version of the build tools.
In order to build the demo on iOS, you need Xcode 7.3 or later. Follow these steps to build the iOS demo apps:
$ cd ~/tensorflow/tensorflow/contrib/lite/examples/ios/camera
$ pod install
$ open tflite_camera_example.xcworkspace
We learned about using TensorFlow models on mobile applications and devices. TensorFlow provides two ways to run on mobile devices: TF Mobile and TF Lite. We learned how to build TF Mobile and TF Lite apps for iOs and Android. We used TensorFlow demo apps as an example.
If you found this post useful, do check out the book Mastering TensorFlow 1.x to skill up for building smarter, faster, and efficient machine learning and deep learning systems.
The 5 biggest announcements from TensorFlow Developer Summit 2018
Getting started with Q-learning using TensorFlow
Implement Long-short Term Memory (LSTM) with TensorFlow