In this recipe, the application uses an SDK to access the APIs that permit queries and updates to the ledger. Now we will perform the following steps:
- Enroll an admin user with the enrollAdmin.js script:
$ sudo node enrollAdmin.js
When we launch the network, an admin user needs to be registered with certificate authority. We send an enrollment call to the CA server and retrieve the enrollment certificate (eCert) for this user. We then use this admin user to subsequently register and enroll other users:
- Register and enroll a user called user1 using the registerUser.js script:
$ sudo node registerUser.js
- With the newly-generated eCert for the admin user, let's communicate with the CA server once more to register and enroll user1. We can use the ID of user1 to query and update the ledger:
- Let's run a query against the ledger:
$ sudo node query.js
- It returns the following screenshot. You will find that there are 10 cars on the network, from CAR0 to CAR9. Each has a color, doctype, make, model, and owner:
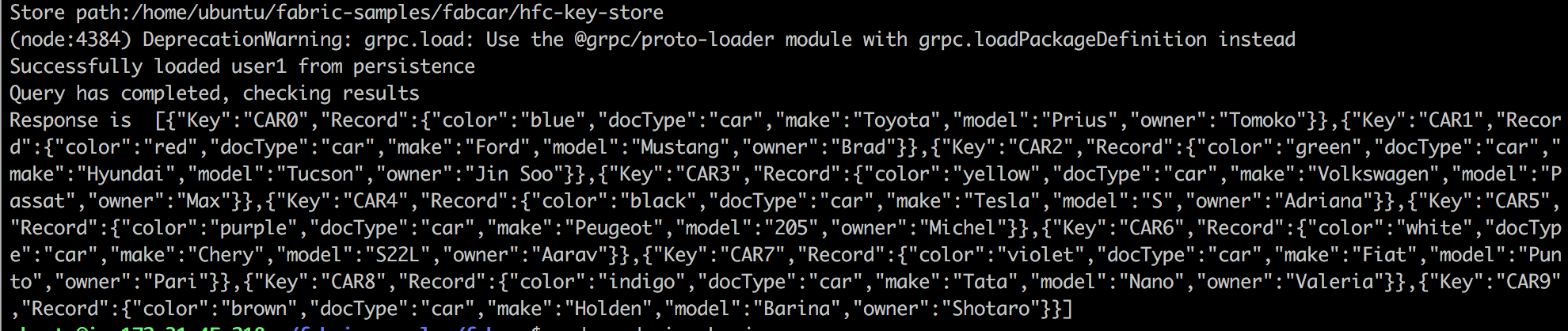
- The following chaincode constructs the query using the queryAllCars function to query all cars:
// queryCar chaincode function - requires 1 argument,
ex: args: ['CAR4'],
// queryAllCars chaincode function - requires no arguments,
ex: args: [''],
const request = {
//targets : --- letting this default to the
peers assigned to the channel
chaincodeId: 'fabcar',
fcn: 'queryAllCars',
args: ['']
}
- Update the ledger. To do this, we will update the invoke.js script. This time, the fabcar chaincode uses the createCar function to insert a new car, CAR10, into the ledger:
var request = {
//targets: let default to the peer assigned to the client
chaincodeId: 'fabcar',
fcn: 'createCar',
args: ['CAR10', 'Chevy', 'Volt', 'Red', 'Nick'],
chainId: 'mychannel',
txId: tx_id
};
sudo node invoke.js
Here we will complete the transaction when CAR10 is created.
- Execute a query to verify the changes made. Change query.js using the queryCar function to query CAR10:
var request = {
//targets: let default to the peer assigned to the client
chaincodeId: 'fabcar',
fcn: 'queryCar',
args: ['CAR10'],
chainId: 'mychannel',
txId: tx_id
};
- Run query.js again. We can now extract CAR10 from the ledger with the response as {"color":"Red","docType":"car","make":"Chevy","model":"Volt","owner":"Nick"}:
sudo node query.js
This will result in the following query:
- Shut down the Fabric network:
sudo docker stop $(sudo docker ps -a -q)
sudo docker rm $(sudo docker ps -a -q)
sudo docker ps
We have gone through the steps to query and update the transaction using smart contract chaincode. Now, let's see how it works under the hood.