The cross-platform programming
Qt is an application programming framework that is used to develop cross-platform applications. What this means is that software written for one platform can be ported and executed on another platform with little or no effort. This is obtained by limiting the application source code to a set of calls to routines and libraries available to all the supported platforms, and by delegating all tasks that may differ between platforms (such as drawing on the screen and accessing system data or hardware) to Qt. This effectively creates a layered environment (as shown in the following figure), where Qt hides all platform-dependent aspects from the application code:
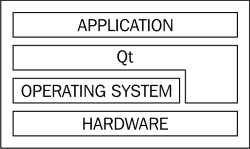
Of course, at times we need to use some functionality that Qt doesn't provide. In such situations, it is important to use conditional compilation like the one used in the following code:
#ifdef Q_OS_WIN32 // Windows specific code #elif defined(Q_OS_LINUX) || defined(Q_OS_MAC) // Mac and Linux specific code #endif
Tip
Downloading the example code
You can download the example code files from your account at http://www.packtpub.com for all the Packt Publishing books you have purchased. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register there to have the files e-mailed directly to you.
What just happened?
Before the code is compiled, it is first fed to a preprocessor that may change the final text that is going to be sent to a compiler. When it encounters a #ifdef
directive, it checks for the existence of a label that will follow (such as Q_OS_WIN32
), and only includes a block of code in compilation if the label is defined. Qt makes sure to provide proper definitions for each system and compiler so that we can use them in such situations.
Tip
You can find a list of all such macros in the Qt reference manual under the term "QtGlobal".
Qt Platform Abstraction
Qt itself is separated into two layers. One is the core Qt functionality that is implemented in a standard C++ language, which is essentially platform-independent. The other is a set of small plugins that implement a so-called Qt Platform Abstraction (QPA) that contains all the platform-specific code related to creating windows, drawing on surfaces, using fonts, and so on. Therefore, porting Qt to a new platform in practice boils down to implementing the QPA plugin for it, provided the platform uses one of the supported standard C++ compilers. Because of this, providing basic support for a new platform is work that can possibly be done in a matter of hours.
Supported platforms
The framework is available for a number of platforms, ranging from classical desktop environments through embedded systems to mobile phones. The following table lists down all the platforms and compiler families that Qt supports at the time of writing. It is possible that when you are reading this, a couple more rows could have been added to this table:
Platform |
QPA plugins |
Supported compilers |
---|---|---|
Linux |
XCB (X11) and Wayland |
GCC, LLVM (clang), and ICC |
Windows XP, Vista, 7, 8, and 10 |
Windows |
MinGW, MSVC, and ICC |
Mac OS X |
Cocoa |
LLVM (clang) and GCC |
Linux Embedded |
DirectFB, EGLFS, KMS, and Wayland |
GCC |
Windows Embedded |
Windows |
MSVC |
Android |
Android |
GCC |
iOS |
iOS |
LLVM (clang) and GCC |
Unix |
XCB (X11) |
GCC |
RTOS (QNX, VxWorks, and INTEGRITY) |
qnx |
qcc, dcc, and GCC |
BlackBerry 10 |
qnx |
qcc |
Windows 8 (WinRT) |
winrt |
MSVC |
Maemo, MeeGo, and Sailfish OS |
XCB (X11) |
GCC |
Google Native Client (unsupported) |
pepper |
GCC |