REPL up!
REPL is the interpreter of Clojure, and it is an acronym of Read Evaluate Print Loop. Unlike other interpreter languages, such as Python or Ruby, Clojure REPL automatically compiles into Java's byte code after the reading expression. Then, REPL evaluates the expression and returns the result of the expression. This dynamic compilation feature of REPL makes Clojure code execution as fast as executing pre-compiled code.
Getting ready
Before you set up your Clojure environment, the Java Development Kit (JDK) is necessary. The JDK version should be 1.6 or later. Throughout the book, we will use JDK 1.8 to develop and test the code.
This is how the command-line result will look, once you type java -version
:
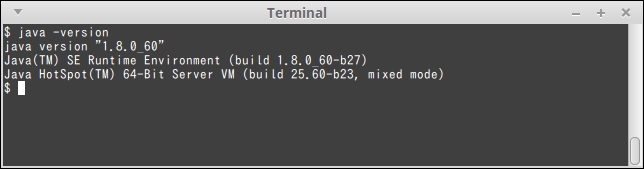
How to do it...
Leiningen is a standard build tool for Clojure. It simplifies the Clojure development, including setting up your project, compiling and testing code, and creating libraries for deployment.
It's easy to set up a Clojure environment using Leiningen. There are only a few steps before you can enjoy Clojure in REPL!
Here are the steps we need to perform to run Clojure REPL:
- Download and set up Leiningen from http://leiningen.org/.
- Download the
lein
script (or on Windows,lein.bat
). - Place it on your
$PATH
where your shell can find it (for example,~/bin
):$ mv lein ~/bin
- Set it to be executable:
$ chmod a+x ~/bin/lein
- Run
lein
, and it will download the self-install package: - Create a new project and go there. Using Leiningen, you can create a project from a project template. This example creates a project called
living-clojure
:$ lein new living-clojure
- Run REPL and put Clojure code into it:
How it works...
Here is a very simple code to demonstrate how REPL works. This code simply loops forever with read
, eval
, and println
functions:
user=> (defn simple-repl [] #_=> (try #_=> (while true #_=> (println (eval (read))) #_=> ) #_=> (catch Exception e (println "exited..")) #_=> ) #_=> ) #'user/simple-repl user=> (simple-repl) (+ 1 1) 2 (defn hello [s] (println "Hello world " s)) #'user/hello (hello "Makoto") Hello world Makoto nil exited.. nil user=>
You can exit simple-repl
by entering ^D
(Ctrl + D).
There's more...
Leiningen is a very powerful tool for Clojure developers. The lein new living-clojure
command generates the following directory structure:
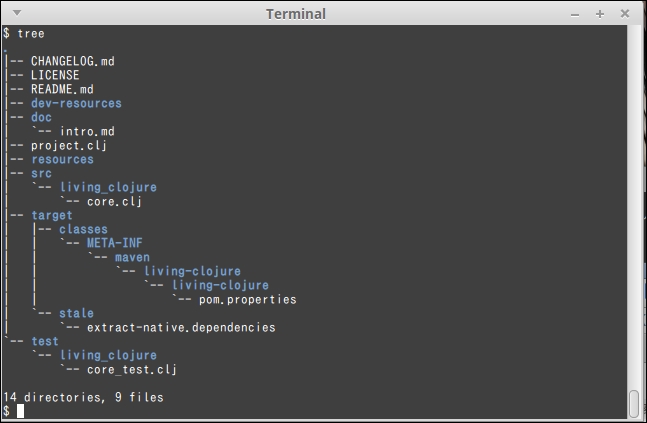
Let's pick up project.clj
, which defines the project:
(defproject living-clojure "0.1.0-SNAPSHOT" :description "FIXME: write description" :url "http://example.com/FIXME" :license {:name "Eclipse Public License" :url "http://www.eclipse.org/legal/epl-v10.html"} :dependencies [[org.clojure/clojure "1.8.0"]])
In project.clj
, the :dependencies
section declares the libraries used by your project.
Leiningen internally uses Maven to solve the dependencies of libraries. However, declaring libraries in project.clj
is much simpler than doing it in the pom.xml
file of Maven.
To use other libraries for your project, add them to the dependency section. We will review how to do this in a later recipe. In the preceding project.clj
file, the Clojure library named org.clojure/clojure
is declared and automatically downloads in the maven
directory. This is the reason why you don't need to download and set up the Clojure library explicitly.
Without Leiningen, you have to do it in a more native way. Here are the steps:
- Download
clojure-1.8.0.jar
. - Download
clojure-1.8.0.jar
usingwget
:$ wget http://repo1.maven.org/maven2/org/clojure/clojure/1.8.0/ clojure-1.8.0.jar
- Run Clojure and test the Clojure code:
$ java -jar clojure-1.8.0.jar Clojure 1.8.0 user=> (+ 1 1) 2
REPL supports the command-line editing feature, like Linux bash shell does, but the preceding way does not. Another difference is that REPL solves library dependency problems in project.clj
, but using the native way, you can solve them by yourself.
See also
Please see related web sites as follows:
- TryClojure is a browser-based Clojure tool that provides a REPL environment. You don't need to set up your own environment: http://www.tryclj.com/
- The REPL and main entry points explain more details about REPL: http://clojure.org/repl_and_main
- Please see the Leiningen web page: http://leiningen.org/
- If you are an emacian, use CIDER and Clojure-mode. You might be very comfortable with CIDER: http://clojure-doc.org/articles/tutorials/emacs.html
- If you are a
vi
user, try the fireplace plugin: https://github.com/tpope/vim-fireplace - If you love IntelliJ/Idea, try to use cursive: https://cursiveclojure.com/
- If you are a traditional Eclipse user, use Counterclockwise: http://doc.ccw-ide.org/documentation.html
- Light table is very cool. It's worth trying: http://lighttable.com/