Keeping sensitive variables safe
In the Manipulating variables recipe in this chapter, we learned how to use variables to make our Terraform configuration more dynamic. By default, all variables’ values used in the configuration will be stored in clear text in the Terraform state file, and this value will also be in clear text in the console output execution.
In this recipe, we will learn how to keep Terraform variable information safe from prying eyes by not displaying their values in clear text in the console output.
Getting ready
To start with, we will use the Terraform configuration available at https://github.com/PacktPublishing/Terraform-Cookbook-Second-Edition/tree/main/CHAP02/sample-app, which provisions an Azure Web App with custom app settings.
To illustrate this, we will add a custom application API key, which has a sensitive value, in the key-value app setting.
The source code of this recipe is available at https://github.com/PacktPublishing/Terraform-Cookbook-Second-Edition/tree/main/CHAP02/sample-app.
How to do it…
Perform the following steps:
- In the
main.tf
file, which contains our Terraform configuration, inazurerm_linux_web_app
we will add anapp_settings
property that is set with theapi_key
variable:resource "azurerm_linux_web_app" "app" { name = "${var.app_name}-${var.environment} -${random_string.random.result}” location = azurerm_resource_group.rg-app.location resource_group_name = azurerm_resource_group.rg-app.name service_plan_id = azurerm_service_plan.plan-app.id site_config {} app_settings = { API_KEY = var.api_key } }
- Then, in
variable.tf
, declare thecustom_app_settings
variable:variable "api_key " { description = "Custom application api key" sensitive = true }
- In
terraform.tfvars
, we instantiate this variable with these values (as an example for this book):api_key = "xxxxxxxxxxxxxxxxx"
- Finally, we run the
terraform plan
command. The following screenshot shows a part of this execution:
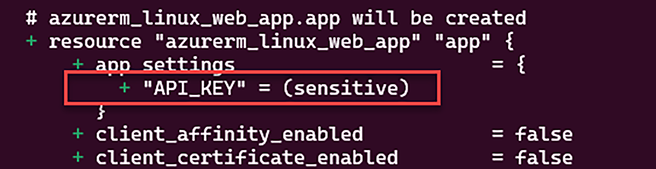
Figure 2.8: Terraform doesn’t display the sensitive variable value
- We can see that the value of the
api_key
property (which is in uppercase in the Terraform plan output) inapp_settings
isn’t displayed in the console output.
How it works…
In the recipe, we add the sensitive
flag to the api_key
variable, which enables the protection of the variable. By enabling this flag, the terraform
plan
command (and the apply
command) doesn’t display the value of the variable in the console output in clear text.
There’s more…
Be careful! The sensitive
variable property protects the value of this variable from being displayed in the console output, but the value of this is still written in clear text in the Terraform state file.
Then, if this Terraform configuration is stored in a source control such as Git, the default value of this variable or the tfvars
file is also readable as clear text in the source code.
So, to protect the value of this variable in source control, we can set the value of this variable by using Terraform’s environment variable technique with the format TF_VAR_<variable name
> as we learned in the previous recipe, Manipulating variables.
In our scenario, we can set the TF_VAR_api_key = "xxxxxxxxxxx"
environment variable just before the execution of the terraform plan
command.
One scenario in which the sensitive variable is effective is when Terraform is executed in a CI/CD pipeline; then, unauthorized users can’t read the value of the variable.
Finally, one best practice is to use an external secret management solution such as Azure Key Vault or HashiCorp Vault (https://www.vaultproject.io/) to store your secrets and use Terraform providers to get these secrets’ values.
See also
- Documentation for
sensitive
variables is available at https://www.terraform.io/language/values/variables#suppressing-values-in-cli-output. - For more information on
sensitive
variables, read the Terraform tutorial at https://learn.hashicorp.com/tutorials/terraform/sensitive-variables.