When coding in UE4, you often want to construct a string from variables. This is pretty easy using the FString::Printf or FString::Format function.
UE4 – making an FString from FStrings and other variables
Getting ready
For this, you should have an existing project into which you can enter some UE4 C++ code. Putting variables into a string is possible via printing. It may be counter intuitive to print into a string, but you can't just concatenate variables together and hope that they will automatically convert into strings, as in some languages, such as JavaScript.
How to do it...
In this recipe, we will see how to print in two different ways. First, we will be using FString::Printf():
- Consider the variables you'd like to be printed into your string. Note what each variable type is.
- Open and take a look at a reference page of the printf format specifiers, such as http://en.cppreference.com/w/cpp/io/c/fprintf. For each variable you want to print, node what the specifier is. For example, %s for a formatted string.
- Try code such as the following:
FString name = "Tim"; int32 mana = 450; FString string = FString::Printf( TEXT( "Name = %s Mana =
%d" ), *name, mana );
Notice how the preceding code block uses the format specifiers precisely like the traditional printf function does. In the preceding example, we used %s to place a string in the formatted string, and %d to place an integer in the formatted string. Different format specifiers exist for different types of variables, and you should look them up on a site such as cppreference.com.
It is also possible to print a string using FString::Format().
- Write code in the following form:
FString name = "Tim"; int32 mana = 450; TArray< FStringFormatArg > args; args.Add( FStringFormatArg( name ) ); args.Add( FStringFormatArg( mana ) );
FString string = FString::Format( TEXT( "Name = {0} Mana =
{1}" ), args );
UE_LOG( LogTemp, Warning, TEXT( "Your string: %s" ),
*string );
With FString::Format(), instead of using correct format specifiers, we use simple integers and a TArray of FStringFormatArg instead. FstringFormatArg helps FString::Format() deduce the type of variable to put in the string. Refer to the following screenshot:
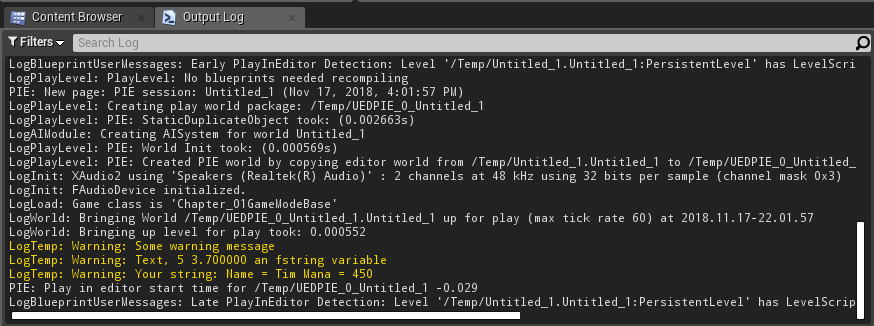
No matter which method you use, upon calling UE_LOG and you will get the same output.