Setting up the working environment
This section briefly discusses the requirements needed to effectively follow the narrative, implement, and run Kivy applications. Personal computer running a modern operating system—a Mac, Linux, or Windows box—is implied.
A note on Python
Python is the primary programming language used in the book; good knowledge of it, while not strictly necessary, may help.
At the time of writing, there are two incompatible versions of Python in wide use. Python 2.7 is monumentally stable but no longer actively developed, and Python 3 is a newer and slightly more controversial version bringing many improvements to the language, but occasionally breaking compatibility along the way.
The code in this book should largely work in both Python versions, but it may need minor adjustments to be fully compatible with Python 3; for best results, it's recommended that you use Python 2.7, or the latest Python 2 version available for your system.
Note
Installing Python separately for Kivy development is not necessary on most platforms: it either comes preinstalled (Mac OS X), bundled with Kivy (MS Windows), or included as a dependency (Linux, Ubuntu in particular).
Installing and running Kivy
Kivy can be downloaded from the official site (http://kivy.org/); just choose an appropriate version and follow the instructions. This whole procedure should be pretty straightforward and simple.
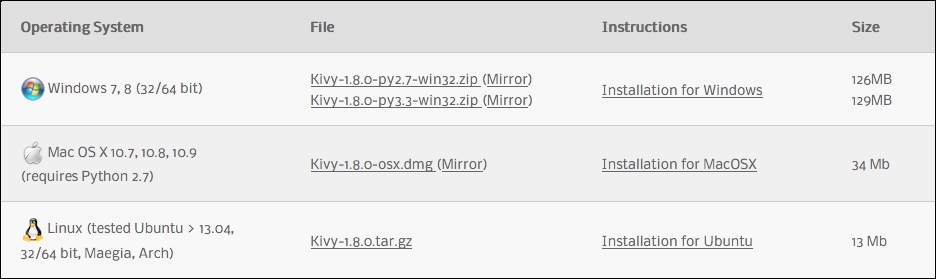
Kivy downloads
To check whether the installation is working, follow these instructions:
- On a Mac:
- Open Terminal.app.
- Run
kivy
. - The Python prompt,
>>>
, should appear. Typeimport kivy
. - The command should complete with no errors, printing a message along the lines of
[INFO] Kivy v1.8.0
.
- On a Linux machine:
- Open a terminal.
- Run
python
. - The Python prompt,
>>>
, should appear. Typeimport kivy
. - The command should print a message similar to
[INFO] Kivy v1.8.0
.
- On a Windows box:
- Double-click kivy.bat inside the Kivy package directory.
- Type
python
at the command prompt. - Type
import kivy
. - The command should print a message similar to
[INFO] Kivy v1.8.0
.
A terminal session
Running a Kivy application (basically, a Python program) is achieved similarly:
- On a Mac, use
kivy main.py
- On Linux, use
python main.py
- On Windows, use
kivy.bat main.py
(or drag-and-drop the main.py file on top of kivy.bat).
Note on coding
Programming typically amounts to working with text a lot; hence, it's important to choose a good text editor. This is why I profoundly recommend trying Vim before you consider other options.
Vim is one of the better text editors largely available; it's highly configurable and built specifically for effective text editing (way more so than a typical alternative). Vim has a vibrant community, is actively maintained, and comes preinstalled with many Unix-like operating systems—including Mac OS X and Linux. It is known that (at least some) developers of the Kivy framework also prefer Vim.
Here are some quick Kivy-related tips for Vim users out there:
- Python-mode (https://github.com/klen/python-mode) is great for writing Python code. It throws in a lot of extra functionality, such as stylistic and static checker, smart completion, and support for refactoring.
- Source code of GLSL shaders can be properly highlighted using the
vim-glsl
syntax (https://github.com/tikhomirov/vim-glsl). - Kivy texture maps (the
.atlas
files, covered in Chapter 8, Introducing Shaders) are basically JSON, so you can use, for example, vim-json (https://github.com/elzr/vim-json), and add a file association to your.vimrc
file like this:au BufNewFile,BufRead *.atlas set filetype=json
- Kivy layout files,
.kv
, are slightly more complicated to handle as they're similar to Python, but don't really parse as Python. There is an incomplete Vim plugin in the Kivy repository, but at the time of writing, Vim's built-in YAML support highlights these files better (this obviously might change in future). To load.kv
files as YAML, add the following line to your.vimrc
file:au BufNewFile,BufRead *.kv set filetype=yaml
Clearly, you are not obliged to use Vim to follow examples of this book—this is but a mere suggestion. Now let's write a bit of code, shall we?