Implementing the main body
In this section, we will complete the third project in this book by implementing the main body of our application. Our first step will be to code the Highlight banner, followed by the app icons.
Coding the highlight banner
Firstly, we will add the code for a Highlight banner. The banner is simply going to be an image that spans the width of the body; we will give it some spacing for aesthetic purposes. It is common to add multiple banners throughout the page to highlight different applications and have carousel banners, which provide the ability to showcase multiple banners in a single section through a transition such as sliding. We will implement a single banner; however, adding more is simple. Follow these steps:
- Let’s start by adding a banner image. My image is 728x90 pixels. Feel free to modify this to suit your needs. Select Assets from the Project Navigator:
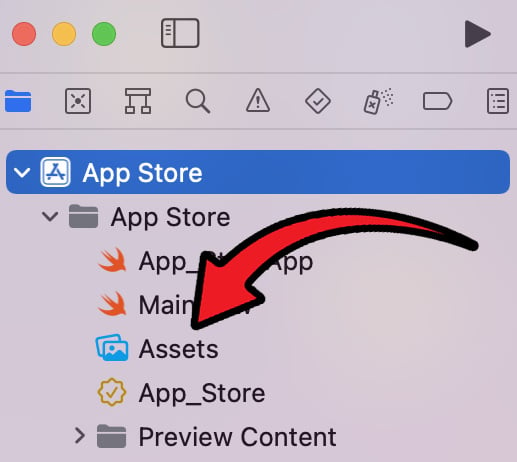
Figure 7.5 – Assets location in Project Navigator
- Now, the Assets view will appear. Importing an image into Assets can be done in one of two ways
- Dragging and dropping the files into the Assets section:
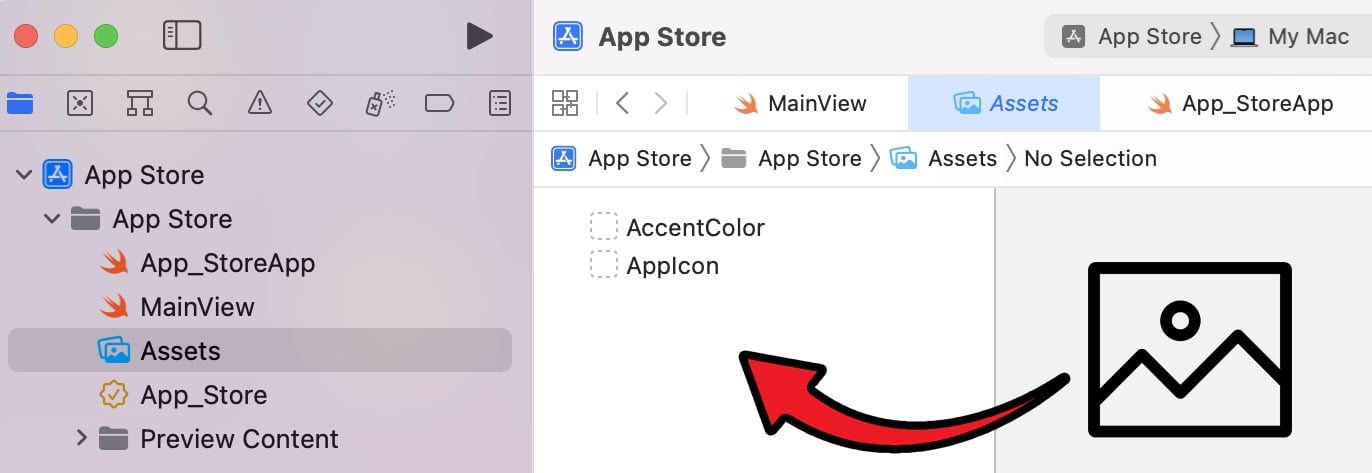
Figure 7.6 – Dragging and dropping asset
- Right-clicking the Assets section and selecting Import…:
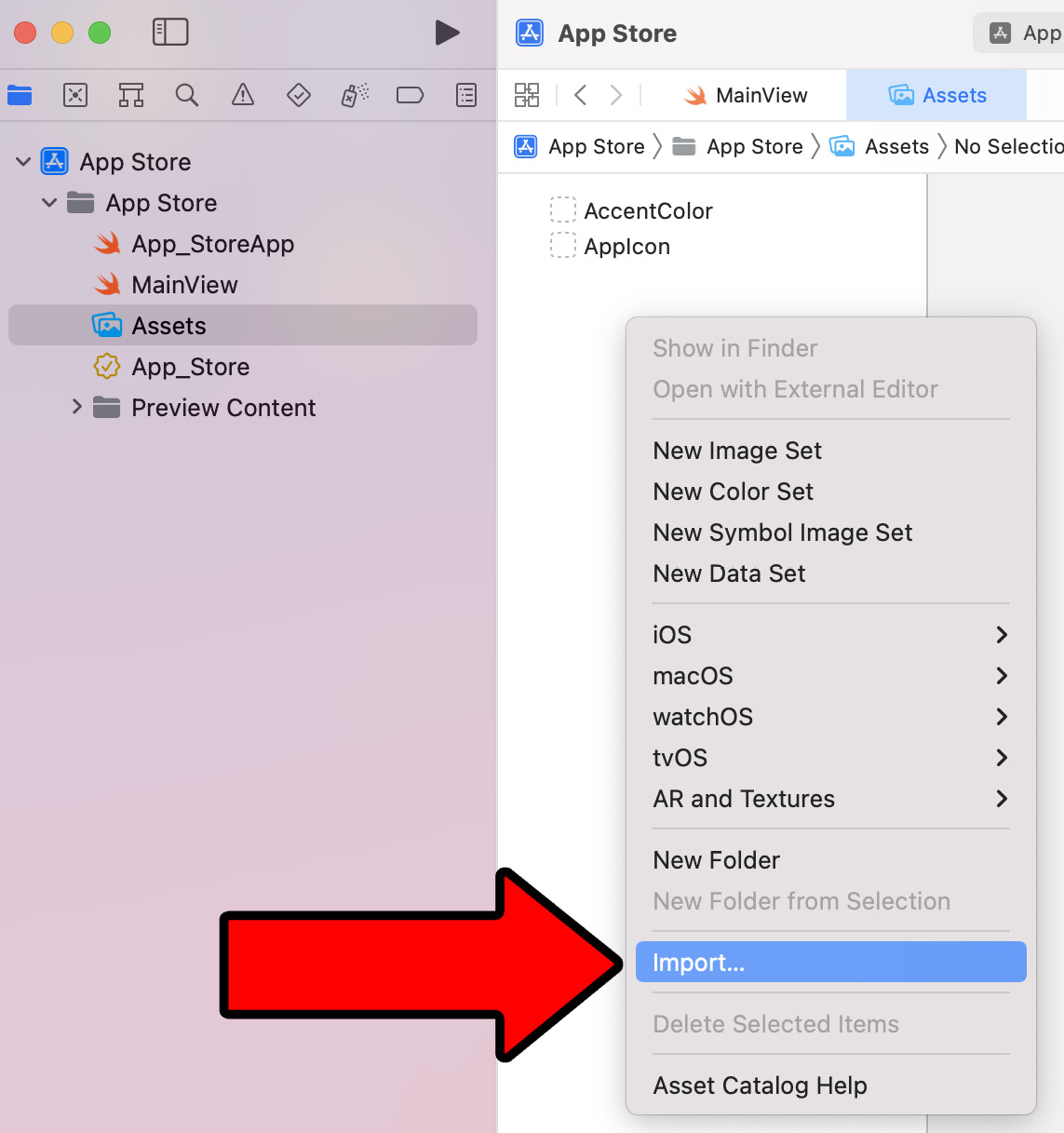
Figure 7.7 – Import… button
Once the asset(s) have been imported, the Assets view will look as follows:
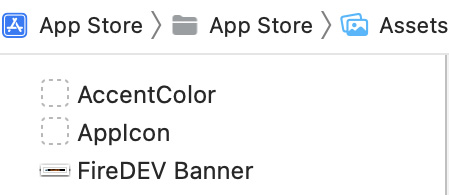
Figure 7.8 – Asset(s) imported
Note
I am using the banner for my developer-centric podcast FireDEV. Feel free to use it and tune in to my podcast every Thursday at the following links:
- Spotify: https://open.spotify.com/show/387RiHksQE33KYHTitFXhg
- Apple Podcasts: https://podcasts.apple.com/us/podcast/firedev-fireside-chat-with-industry-professionals/id1602599831
- Google Podcasts: https://podcasts.google.com/feed/aHR0cHM6Ly9hbmNob3IuZm0vcy83Yjg2YTNiNC9wb2RjYXN0L3Jzcw
- We will add an
Image
component after theList
code:}.searchable( text: $searchText ) .onSubmit( of: .search ) { print( searchText ) } Image( "FireDEV Banner" )
This will result in the following:
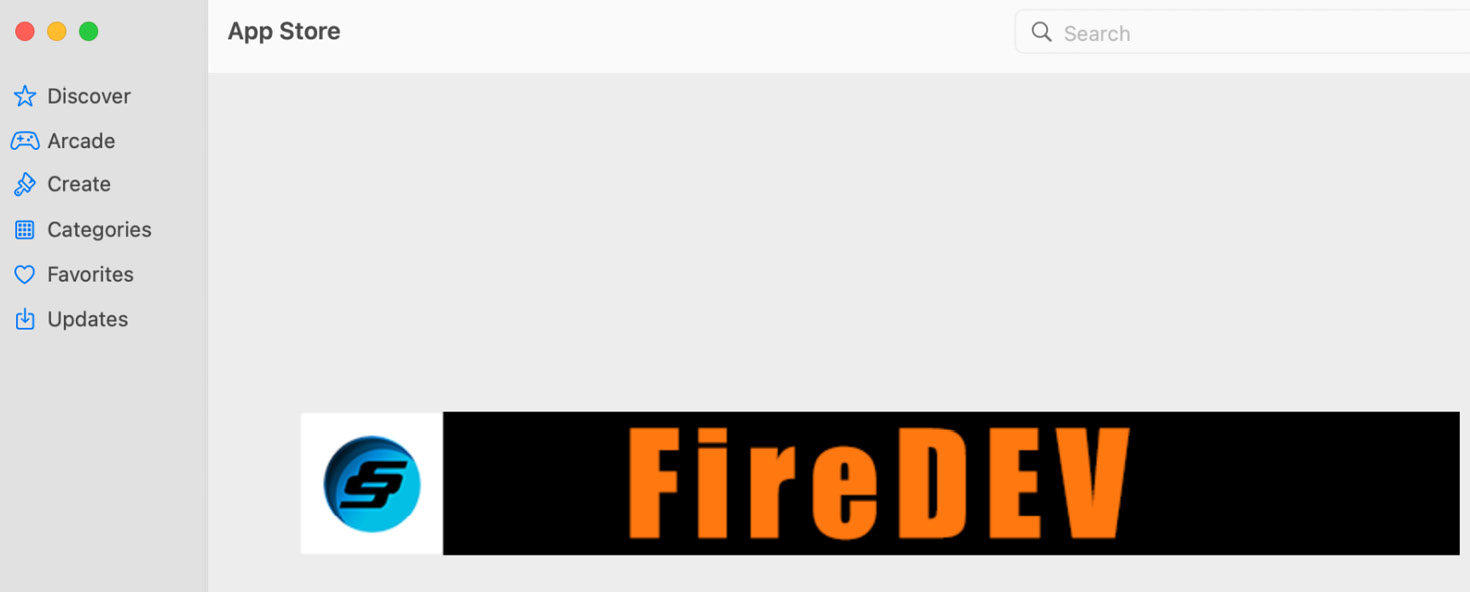
Figure 7.9 – Banner added
- If you try and resize the window, there are restrictions. We need to make the banner resizable and maintain its original aspect ratio. Update the image code as follows:
Image( "FireDEV Banner" ) .resizable( ) .padding( .horizontal ) .scaledToFit( )
We made the image resizable, which allows it to change size based on the size of the window. This is very useful as the user will run the App Store on different screen sizes and may not always have it fullscreen. We then added horizontal padding to make sure it doesn’t touch the left or right edges. This can be omitted if you like, or you can specify a set amount of padding. Finally, we set it to
scaledToFit
, which maintains the original aspect ratio. Distortion is never a good idea. All of this results in the following:
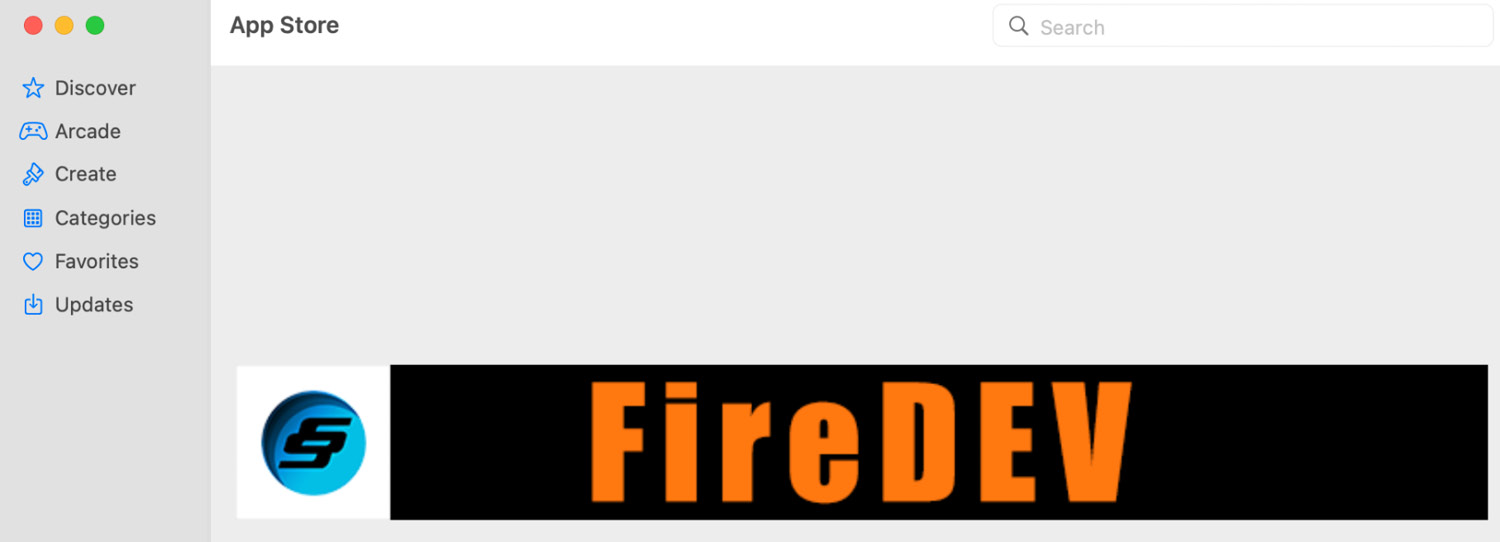
Figure 7.10 – Banner updated
- As of now, the banner is always in the center. We want it at the top of the view. To achieve this result, we will enclose the image code we added previously within a
ScrollView
with an alignment oftopLeading
. Update the code like so:ScrollView { Color.clear Image( "FireDEV Banner" ) .resizable( ) .padding( .horizontal ) .scaledToFit( ) }
We also add a
Color.clear
instruction to make sure there is no background color, all of which results in the following awesome banner:
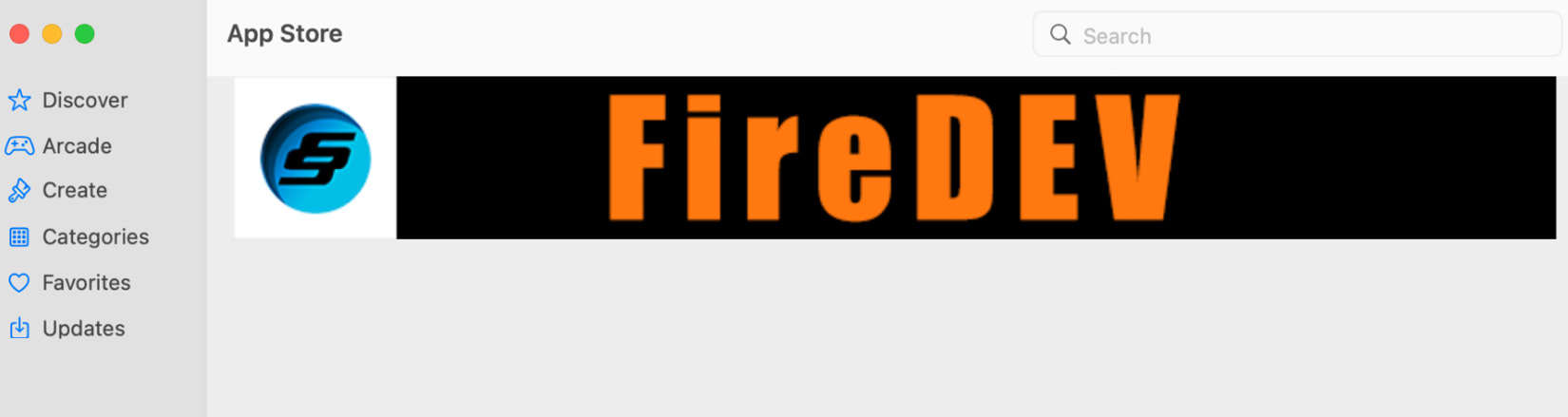
Figure 7.11 – Banner positioned at the top
The highlight banner has been finished, which can be converted into a carousel. Moving forward, the app groups will be coded to showcase a list of application icons.