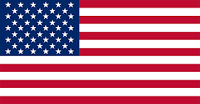

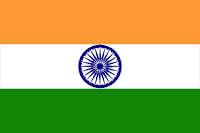
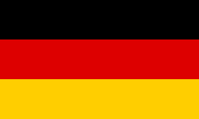
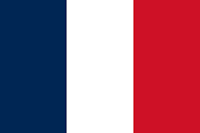

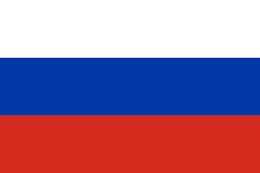
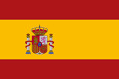



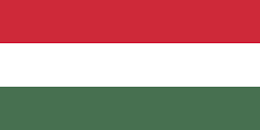

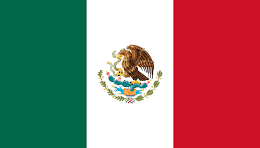
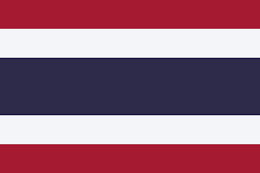
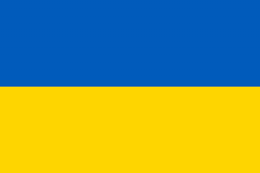
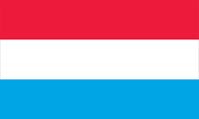

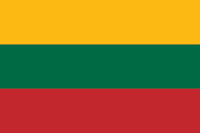

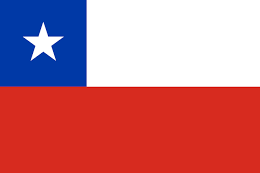

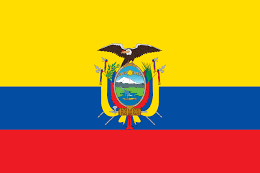

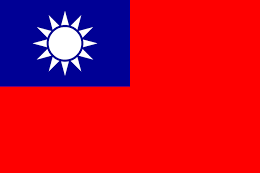

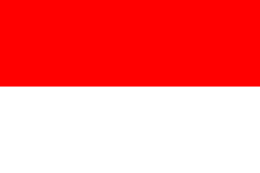
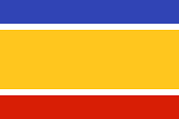
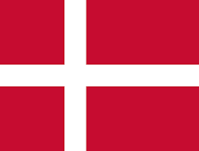
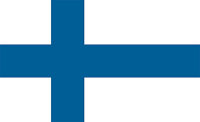


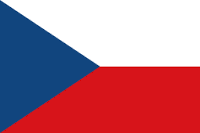
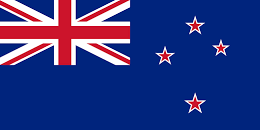
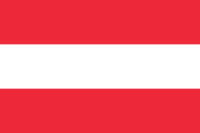
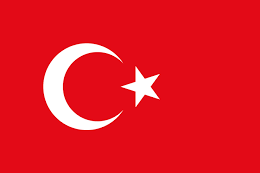
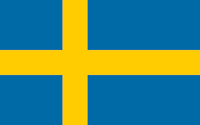
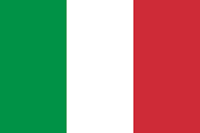
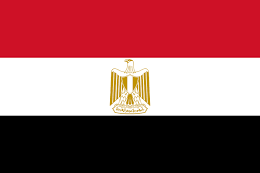

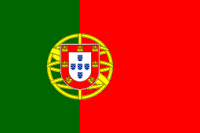
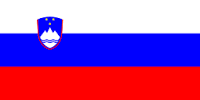

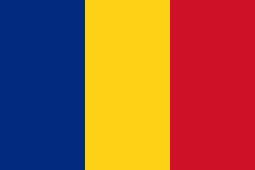
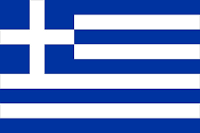

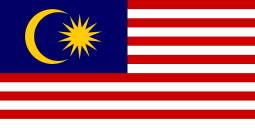
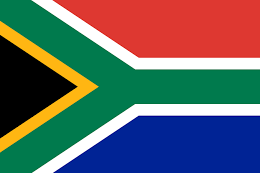

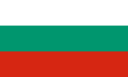
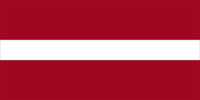


Dive deeper into the world of AI innovation and stay ahead of the AI curve! Subscribe to our AI_Distilled newsletter for the latest insights. Don't miss out – sign up today!
This article is an excerpt from the book, Building AI Applications with ChatGPT APIs, by Martin Yanev. Master core data architecture design concepts and Azure Data & AI services to gain a cloud data and AI architect’s perspective to developing end-to-end solutions
Before we start writing our first code, it’s important to create an environment to work in and install any necessary dependencies. Fortunately, Python has an excellent tooling system for managing virtual environments. Virtual environments in Python are a complex topic, but for the purposes of this book, it’s enough to know that they are isolated Python environments that are separate from your global Python installation. This isolation allows developers to work with different Python versions, install packages within the environment, and manage project dependencies without interfering with Python’s global installation.
In order to utilize the ChatGPT API in your NLP projects, you will need to set up your Python development environment. This section will guide you through the necessary steps to get started, including the following:
A properly configured development environment will allow you to make API requests to ChatGPT and process the resulting responses in your Python code.
Python is a popular programming language that is widely used for various purposes, including machine learning and data analysis. You can download and install the latest version of Python from the official website, https://www.python.org/downloads/. Once you have downloaded the Python installer, simply follow the instructions to install Python on your computer. The next step is to choose an Integrated Development Environment (IDE) to work with (see Figure 1.7).
Figure 1.7: Python Installation
One popular choice among Python developers is PyCharm, a powerful and user-friendly IDE developed by JetBrains. PyCharm provides a wide range of features that make it easy to develop Python applications, including code completion, debugging tools, and project management capabilities.
To install PyCharm, you can download the Community Edition for free from the JetBrains website, https://www.jetbrains.com/pycharm/download/ Once you have downloaded the installer, simply follow the instructions to install PyCharm on your computer.
Setting up a Python virtual environment is a crucial step in creating an isolated development environment for your project. By creating a virtual environment, you can install specific versions of Python packages and dependencies without interfering with other projects on your system.
Creating a Python virtual environment specific to your ChatGPT application project is a recommended best practice. By doing so, you can ensure that all the packages and dependencies are saved inside your project folder rather than cluttering up your computer’s global Python installation. This approach provides a more organized and isolated environment for your project’s development and execution.
PyCharm allows you to set up the Python virtual environment directly during the project creation process. Once installed, you can launch PyCharm and start working with Python. Upon launching PyCharm, you will see the Welcome Window, and from there, you can create a new project. By doing so, you will be directed to the New Project window, where you can specify your desired project name and, more importantly, set up your Python virtual environment. To do this, you need to ensure that New environment using is selected. This option will create a copy of the Python version installed on your device and save it to your local project.
As you can see from Figure 1.8, the Location field displays the directory path of your local Python virtual environment situated within your project directory. Beneath it, the Base interpreter displays the installed Python version on your system. Clicking the Create button will initiate the creation of your new project.
Figure 1.8: PyCharm Project Setup
Figure 1.9 displays the two main indicators showing that the Python virtual environment is correctly installed and activated. One of these indications is the presence of a venv folder within your PyCharm project, which proves that the environment is installed. Additionally, you should observe Python 3.11 (ChatGPTResponse) in the lower-right corner, confirming that your virtual environment has been activated successfully.
Figure 1.9: Python Virtual Environment Indications
A key component needed to install any package in Python is pip. Lets’s see how to check whether pip is already installed on your system, and how to install it if necessary.
pip is a package installer for Python. It allows you to easily install and manage third-party Python libraries and packages such as openai. If you are using a recent version of Python, pip should already be installed. You can check whether pip is installed on your system by opening a command prompt or terminal and typing pip followed by the Enter key. If pip is installed, you should see some output describing its usage and commands.
If pip is not installed on your system, you can install it by following these steps:
1. First, download the get-pip.py script from the official Python website: https:// bootstrap.pypa.io/get-pip.py.
2. Save the file to a location on your computer that you can easily access, such as your desktop or downloads folder.
3. Open a command prompt or terminal and navigate to the directory where you saved the get-pip.py file.
4. Run the following command to install pip: python get-pip.py
5. Once the installation is complete, you can verify that pip is installed by typing pip into the command prompt or terminal and pressing Enter.
You should now have pip installed on your system and be able to use it to install packages and libraries for Python.
Alternatively, to create a Python virtual environment, you can use the built-in venv module that comes with Python. Once you create your project in PyCharm, click on the Terminal tab located at the bottom of the screen. If you don’t see the Terminal tab, you can open it by going to View | Tool Windows | Terminal in the menu bar. Then, run this command:
$ python3 -m venv myenv
This will create a new directory named myenv that contains the virtual environment. You can replace myenv with any name you want.
To activate the virtual environment, run the following command:
$ myenv\Scripts\activate.bat
$ source myenv/bin/activate
Once activated, you should see the name of the virtual environment in the command prompt or terminal. From here, you can install any packages or dependencies you need for your project without interfering with other Python installations on your system.
This was a complete guide on how to set up a Python development environment for using the ChatGPT API in NLP projects. The steps included installing Python, the PyCharm IDE, and pip, and setting up a virtual environment. Setting up a virtual environment was a crucial step in creating an isolated development environment for your project. You are now ready to complete your first practice exercise on using the ChatGPT API with Python to interact with the OpenAI library.
Using the ChatGPT API with Python is a relatively simple process. You’ll first need to make sure you create a new PyCharm project called ChatGPTResponse (see Figure 1.8). Once you have that set up, you can use the OpenAI Python library to interact with the ChatGPT API. Open a new Terminal in PyCharm, make sure that you are in your project folder, and install the openai package:
$ pip install openai
Next, you need to create a new Python file in your PyCharm project. In the top-left corner, right-click on ChatGPTResponse | New | Python File. Name the file app.py and hit Enter. You should now have a new Python file in your project directory.
Figure 1.10: Create a Python File
To get started, you’ll need to import the openai library into your Python file. Also, you’ll need to provide your OpenAI API key. You can obtain an API key from the OpenAI website by following the steps outlined in the previous sections of this book. Then you’ll need to set it as a parameter in your Python code. Once your API key is set up, you can start interacting with the ChatGPT API:
import openai
openai.api_key = "YOUR_API_KEY"
Replace YOUR_API_KEY with the API key you obtained from the OpenAI platform page. Now, you can ask the user for a question using the input() function:
question = input("What would you like to ask ChatGPT? ")
The input() function is used to prompt the user to input a question they would like to ask the ChatGPT API. The function takes a string as an argument, which is displayed to the user when the program is run. In this case, the question string is "What would you like to ask ChatGPT?". When the user types their question and presses Enter, the input() function will return the string that the user typed. This string is then assigned to the question variable.
To pass the user question from your Python script to ChatGPT, you will need to use the ChatGPT API Completion function:
response = openai.Completion.create(
engine="text-davinci-003",
prompt=question,
max_tokens=1024,
n=1,
stop=None,
temperature=0.8,
)
The openai.Completion.create() function in the code is used to send a request to the ChatGPT API to generate the completion of the user’s input prompt. The engine parameter allows us to specify the specific variant or version of the GPT model we want to utilize for the request, and in this case, it is set to "text-davinci-003". The prompt parameter specifies the text prompt for the API to complete, which is the user’s input question in this case.
The max_tokens parameter specifies the maximum number of tokens the request and the response should contain together. The n parameter specifies the number of completions to generate for the prompt. The stop parameter specifies the sequence where the API should stop generating the response.
The temperature parameter controls the creativity of the generated response. It ranges from 0 to 1. Higher values will result in more creative but potentially less coherent responses, while lower values will result in more predictable but potentially less interesting responses. Later in the book, we will delve into how these parameters impact the responses received from ChatGPT.
The function returns a JSON object containing the generated response from the ChatGPT API, which then can be accessed and printed to the console in the next line of code:
print(response)
In the project pane on the left-hand side of the screen, locate the Python file you want to run. Right-click on the app.py file and select Run app.py from the context menu. You should receive a message in the Run window that asks you to write a question to ChatGPT (see Figure 1.11).
Figure 1.11: Asking ChatGPT a Question
Once you have entered your question, press the Enter key to submit your request to the ChatGPT API. The response generated by the ChatGPT API model will be displayed in the Run window as a complete JSON object:
{
"choices": [
{
"finish_reason": "stop",
"index": 0,
"logprobs": null,
"text": "\n\n1. Start by getting in the water. If you're swimming in a pool, you can enter the water from the side, ………….
}
],
"created": 1681010983,
"id": "cmpl-73G2JJCyBTfwCdIyZ7v5CTjxMiS6W",
"model": "text-davinci-003",
"object": "text_completion",
"usage": {
"completion_tokens": 415,
"prompt_tokens": 4,
"total_tokens": 419
}
}
This JSON response produced by the OpenAI API contains information about the response generated by the GPT-3 model. This response consists of the following fields:
The most important parameter from the response is the text field, which contains the answer to the question asked to the ChatGPT API. This is why most API users would like to access only that parameter from the JSON object. You can easily separate the text from the main body as follows:
answer = response["choices"][0]["text"]
print(answer)
By following this approach, you can guarantee that the answer variable will hold the complete ChatGPT API text response, which you can then print to verify. Keep in mind that ChatGPT responses can significantly differ depending on the input, making each response unique.
OpenAI:
1. Start by getting in the water. If you're swimming in a pool, you can enter the water from the side, ladder, or diving board. If you are swimming in the ocean or lake, you can enter the water from the shore or a dock.
2. Take a deep breath in and then exhale slowly. This will help you relax and prepare for swimming.
ChatGPT can be employed for a multitude of NLP tasks across a wide array of topics accessible in the vast expanse of available data. We can utilize our script to inquire about diverse areas of expertise and receive advanced responses from the ChatGPT API, as demonstrated here:
1. Mathematics:
* User: What is the square root of 256?
* ChatGPT: The square root of 256 is 16.
2. Sentiment analysis:
* User: Analyze the sentiment of the sentence “I had a great day today!”
* ChatGPT: The sentiment of the sentence “I had a great day today!” is positive.
3. Programming:
* User: How do you declare a variable in Python?
* ChatGPT: To declare a variable in Python, you can use the following syntax: variable_ name = value.
4. Science:
* User: What is the process of photosynthesis in plants?
* ChatGPT: The process of photosynthesis in plants is the fundamental mechanism by which they convert light energy into chemical energy.
5. Literature:
* User: What are the major themes in Shakespeare’s play “Hamlet”?
* ChatGPT: “Hamlet,” one of Shakespeare’s most renowned tragedies, explores several major themes that continue to captivate audiences and provoke thought.
In this section, you learned how to use the OpenAI Python library to interact with the ChatGPT API by sending a request to generate the completion of a user’s input prompt/question. You also learned how to set up your API key and how to prompt the user to input a question, and finally, how to access the generated response from ChatGPT in the form of a JSON object containing information about the response. You are now ready to build more complex projects and integrate the ChatGPT API with other frameworks.
In this article, we covered how to set up a Python development environment, specifically using the PyCharm IDE, and creating a virtual environment. To help you get started with using the ChatGPT API, we walked through a simple example of obtaining a ChatGPT API response.
Martin Yanev is an experienced Software Engineer who has worked in the aerospace and industries for over 8 years. He specializes in developing and integrating software solutions for air traffic control and chromatography systems. Martin is a well-respected instructor with over 280,000 students worldwide, and he is skilled in using frameworks like Flask, Django, Pytest, and TensorFlow. He is an expert in building, training, and fine-tuning AI systems with the full range of OpenAI APIs. Martin has dual master's degrees in Aerospace Systems and Software Engineering, which demonstrates his commitment to both practical and theoretical aspects of the industry.