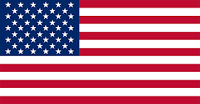

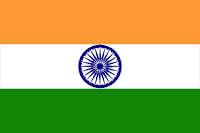
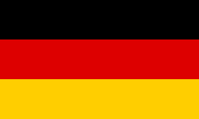
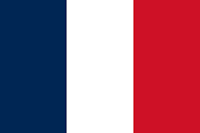

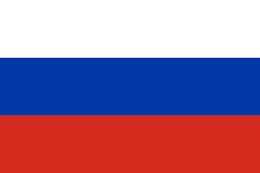
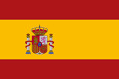



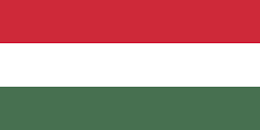

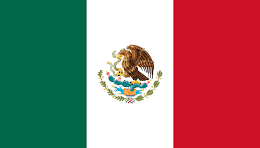
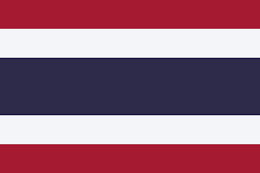
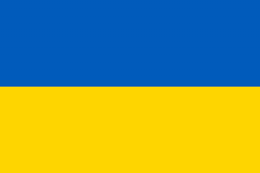
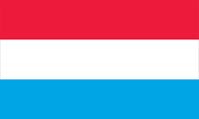

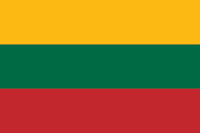

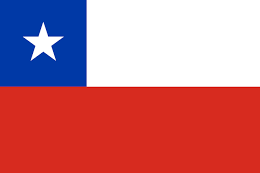

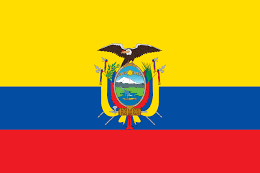

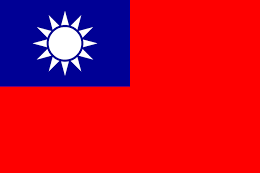

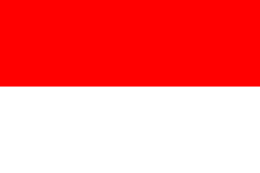
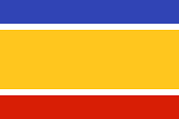
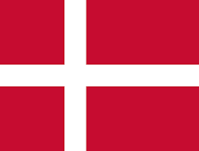
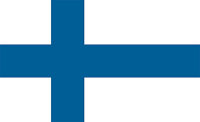


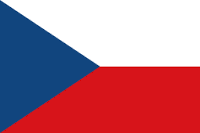
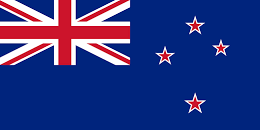
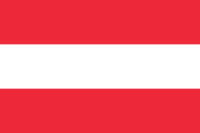
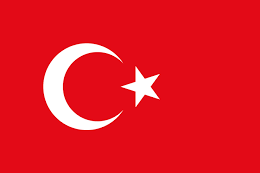
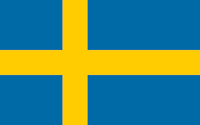
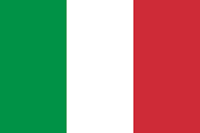
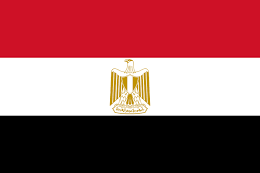

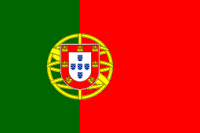
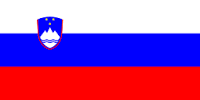

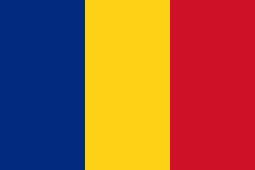
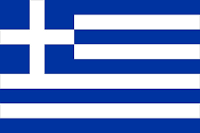

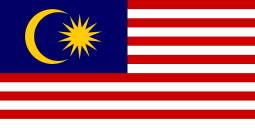
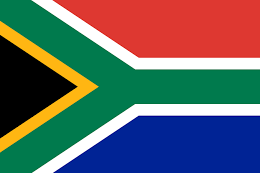

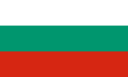
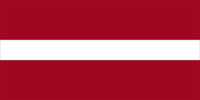


ChatGPT has been making the rounds recently in the world of programming. In short, there is a lot of hoopla about whether or not it will replace programmers, how well it writes code, how well it troubleshoots issues, and more importantly how programmers can leverage it to automate code production. One area where ChatGPT can be of particular use is in the field of automation. As all automation programmers know, the automation industry is very fast-paced. This means that being able to quickly produce quality code is vital to the success of not only the programmer but the company as a whole. However, it is often the case that some of the simplest code that a developer will need to produce is often the most time-consuming to write. Two areas that come to mind are large case statements and sorting algorithms. As such, the following article is going to explore how ChatGPT can be used to produce code that would otherwise be monotonous or time-consuming to write. More specifically, the following is going to explore how ChatGPT can be used to generate the necessary code for a case statement that displays a motor’s health and how it can be used to select and generate the code for a sorting algorithm to sort part sizes.
A very daunting and time-consuming task that all automation programmers will perform regularly is creating large case statements. It is not uncommon for automation programmers to have to build things like state machines that have 10s or even 100s of different cases. As anyone who has built these before knows, building structures like these is time-consuming and monotonous at best. For example, suppose a developer is working on a function block that will display a custom message about a motor error in an HMI. Assume in this example the developer needs a code block that can handle the input/output exchange for the following table,
Input value | String Message |
---|---|
1 | Cable Error |
2 | Motor Overheated |
3 | Under Current |
Depending on the experience level of the developer a simple case statement program that can handle the requirements would take about five minutes. However, when there are more case statements, for example, 30 or 40 inputs, the timeframe for completion could drastically increase. Simply put, since there are more cases there will be more code to write. In turn, this means that it will take the developer more time to type out the code and there will be a higher probability of introducing a bug or bugs in the code due to human error.
On the other hand, to quickly generate a quality code block that can meet these requirements all one would need to do is enter the following into ChatGPT:
write an IEC 61131-3 code block that will set a message variable to "cable error" when the input is 1, "motor overheated" when the input is 2, and "under current" when the input is 3
This statement will output the following:
FUNCTION_BLOCK SetMessage
VAR_INPUT
Input: INT; // Input value
END_VAR
VAR_OUTPUT
Message: STRING(50); // Output message
END_VAR
METHOD SetMessage
VAR
TempMessage: STRING(50); // Temporary message variable
END_VAR
CASE Input OF
1:
TempMessage := 'Cable error';
2:
TempMessage := 'Motor overheated';
3:
TempMessage := 'Under current';
ELSE
TempMessage := 'Invalid input'; // Optional: default message for other input values
END_CASE
Message := TempMessage; // Assign the temporary message to the output message
END_METHOD
From the time it took to input and run the query on ChatGPT to the final code output was about one minute. The code that was generated is quality code that looks like it was produced by an experienced programmer, it is documented, and above all else works. The code even has an optional else statement to catch invalid inputs. Overall, the autogenerated code is no less than what would be expected from a human developer. With that, let’s explore how ChatGPT can be used to generate a more complex piece of software.
It is not uncommon for developers to be tasked with finding the smallest/largest or lightest/heaviest part produced on a production run. For a task like this, it is common to store all the sizes, weights, or whatever it may be in an array and at one point or another sort the array to find the greatest and least size. Depending on the size of the array, or in the case of this scenario, the number of part sizes to sort, having an efficient array is vital. For this task, an efficient sorting algorithm will need to be picked and the code will need to be implemented.
Assuming the PLC programmer is knowledgeable of sorting algorithms and Big O notations selecting an algorithm may only take a few minutes. However, for a PLC programmer that is not versed in these topics, such as a person that is perhaps and mechanical engineer or a technician of some type, this task will probably require a Google search which ideally will take about five minutes. Regardless, of whether the programmer is experienced or not with the algorithm it will take roughly 10 to 20 minutes for the person to type in the code. As such, on a good day, it would take roughly 10 to 25 minutes for someone to implement a sorting algorithm.
On the other hand, if a person used ChatGPT all they would have to do is type in the following query:
write an IEC 61131-3 Structured Text sorting algorithm that can sort the elements in an array with 100 entries with the best possible Big O time
Which will generate the following:
FUNCTION_BLOCK QuickSort
VAR_INPUT
arr: ARRAY[1..100] OF INT;
END_VAR
METHOD SortArray : BOOL
SortPartition(arr, 1, 100);
SortArray := TRUE;
END_METHOD
METHOD SortPartition(arr: ARRAY[1..100] OF INT; low: INT; high: INT)
VAR
pivot: INT;
partitionIndex: INT;
END_VAR
IF low < high THEN
pivot := Partition(arr, low, high, low); // Choosing pivot as the first element
SortPartition(arr, low, pivot - 1);
SortPartition(arr, pivot + 1, high);
END_IF;
END_METHOD
METHOD Partition(arr: ARRAY[1..100] OF INT; low: INT; high: INT; pivotIndex: INT) : INT
VAR
pivotValue: INT;
i: INT;
partitionIndex: INT;
temp: INT;
END_VAR
// Swap pivot element to the end
temp := arr[high];
arr[high] := arr[pivotIndex];
arr[pivotIndex] := temp;
pivotValue := arr[high];
partitionIndex := low;
FOR i := low TO high - 1 DO
IF arr[i] <= pivotValue THEN
// Swap elements
temp := arr[i];
arr[i] := arr[partitionIndex];
arr[partitionIndex] := temp;
partitionIndex := partitionIndex + 1;
END_IF;
END_FOR;
// Move pivot element to its correct position
temp := arr[partitionIndex];
arr[partitionIndex] := arr[high];
arr[high] := temp;
Partition := partitionIndex;
END_METHOD
END_FUNCTION_BLOCK
All of this code was generated in about one minute. As can be seen, ChatGPT generated roughly 60 lines of code not counting the code that utilizes the example. The only overhead that would be required by the programmer is to create the necessary files and paste the code in. In terms of efficiency, ChatGPT rendered the Quicksort algorithm which has a Big O of n*log(n). So, in the end, the programmer is probably looking at around five minutes' worth of work to implement an efficient algorithm that would otherwise take up to half an hour by hand.
As can be seen, the key to using ChatGPT as a programming assistant is simply learning to talk to it. Unlike programming languages that have a unique syntax, ChatGPT can interpret normal, human language. However, to get the most out of ChatGPT the programmer needs to learn to ask detailed questions. The general rule of thumb is the more detailed a statement is the more detailed the solution will be. As was seen, in the examples, all the code was produced with a single sentence. Depending on the resolution needed, a simple query can produce great results, but it should be noted that if specs like specific ports, and so on need to be addressed the user should specify those. Though novel now, it is likely that the art of talking to these systems will soon appear to generate optimal code with a query.
In all, ChatGPT can be used as a tool to help speed up the development process. As was explored with the motor health code block and the sorting algorithm, ChatGPT can turn a simple phrase into workable code that would take a human a considerable amount of time to type out. In short, even if a PLC programmer is knowledgeable of both programming principles, algorithms, and other computer science concepts they will always be bottlenecked by having to implement the code if they cannot simply cut and paste it from another source.
When used in a way that was explored, ChatGPT is a great productivity tool. It can be used to greatly reduce the amount of time needed to implement and if necessary find a solution. Overall, ChatGPT needs guidance to arrive at a proper solution and the person driving the AI needs to be competent enough to implement the solution. However, when in the right hands ChatGPT and similar AI systems can greatly improve development time.
M.T. White has been programming since the age of 12. His fascination with robotics flourished when he was a child programming microcontrollers such as Arduino. M.T. currently holds an undergraduate degree in mathematics, and a master's degree in software engineering, and is currently working on an MBA in IT project management. M.T. is currently working as a software developer for a major US defense contractor and is an adjunct CIS instructor at ECPI University. His background mostly stems from the automation industry where he programmed PLCs and HMIs for many different types of applications. M.T. has programmed many different brands of PLCs over the years and has developed HMIs using many different tools.
Author of the book: Mastering PLC Programming