The __init__ method
In Exercise 72 – creating a Pet class, you used the Pet
class to create a Pet
object called chubbles
in the following manner:
chubbles = Pet()
Here, you’ll explore more about what happens when you create objects from a class in this manner.
Python has a special method called __init__
, which is called when you initialize an object from one of our class templates. For example, building on the previous exercise, suppose you wanted to specify the height of a pet. You would add an __init__
method as follows:
class Pet:
"""
A class to capture useful information regarding my pets, just in case
I lose track of them.
"""
def __init__(self, height):
self.height = height
is_human = False
owner = 'Michael Smith'
The init
method takes the height value and assigns it as an attribute of our new object. You can test this as follows:
chubbles = Pet(height=5)
chubbles.height
This will give us the following output:
out: 5
Exercise 73 – creating a Circle class
The aim of this exercise is to use the init
method. You will create a new class called Circle
with an init
method that allows us to specify the radius and color of a new Circle
object. You then use this class to create two circles:
- Create a
Circle
class with a class attribute calledis_shape
:class Circle:
is_shape = True
- Add an
init
method to our class, allowing us to specify the radius and color of the specific circle:class Circle:
is_shape = True
def __init__(self, radius, color):
self.radius = radius
self.color = color
- Initialize two new
Circle
objects with different radii and colors:first_circle = Circle(2, 'blue')
second_circle = Circle(3, 'red')
Let’s have a look at some of the attributes of the Circle
objects:
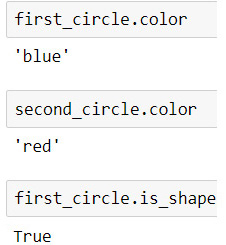
Figure 5.4 – Checking the attributes of our circles
In this exercise, you learned how to use the init
method to set instance attributes.
Note
Any Circle
objects created from our Circle
class will always have is_shape = True
but may have different radii and colors. This is because is_shape
is a class attribute defined outside of the init
method, and radius
and color
are instance attributes set in the init
method.
Keyword arguments
As we learned in Chapter 3, Executing Python – Programs, Algorithms, and Functions, in the Basic functions section, there are two types of arguments that can go into functions – positional arguments and keyword arguments (kwargs
). Remember that positional arguments are listed first and must be specified when calling a function, whereas keyword arguments are optional:
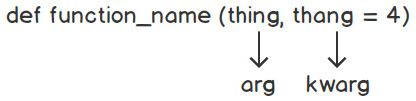
Figure 5.5 – Args and kwargs
The examples so far in this chapter have just contained positional arguments. However, you may want to provide a default value for an instance attribute. For example, you can take your previous example and add a default value for color
:
class Circle:
is_shape = True
def __init__(self, radius, color='red'):
self.radius = radius
self.color = color
Now, if you initialize a circle without specifying a color, it will default to red:
my_circle = Circle(23)
my_circle.color
You will get the following output:
'red'
Exercise 74 – the Country class with keyword arguments
The aim of this exercise is to use keyword arguments to allow optional instance attribute inputs to be specified in the init
function.
You create a class called Country
, where there are three optional attributes that can be passed into the init
method:
- Create the
Country
class with three keyword arguments to capture details about theCountry
object:class Country:
def __init__(self, name='Unspecified', population=None, size_kmsq=None):
self.name = name
self.population = population
self.size_kmsq = size_kmsq
- Initialize a new
Country
, noting that the order of parameters does not matter because you are using named arguments:usa = Country(name='United States of America', size_kmsq=9.8e6)
Note
Here, e
is shorthand for “10 to the power of” – for instance, 2e4 == 2 x 10 ^ 4 == 20,000.
- Use the
__dict__
method to view a list of the attributes of theusa
object:usa.__dict__
You will get the following output:

Figure 5.6 – Dictionary output of our usa object
In this exercise, you learned how keyword arguments can be used when initializing an object with a class.