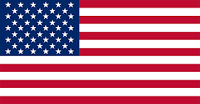

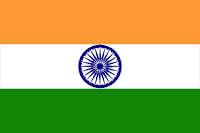
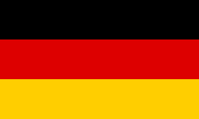
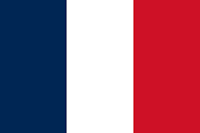

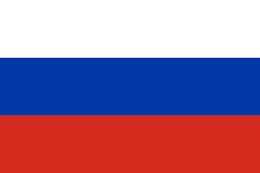
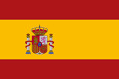



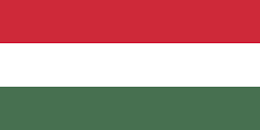

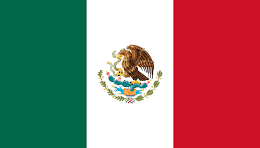
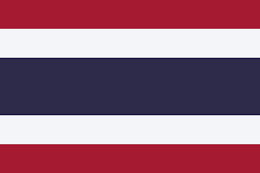
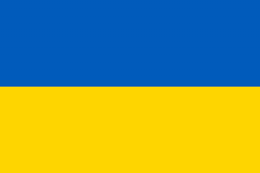
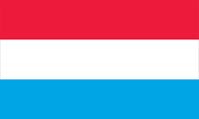

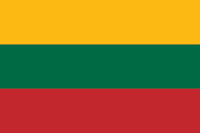

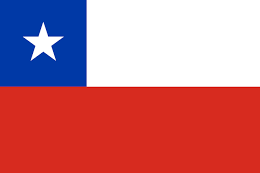

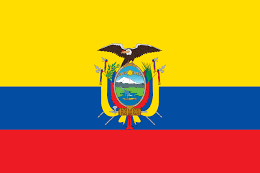

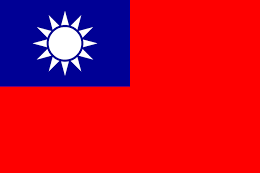

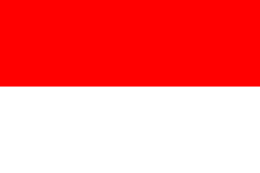
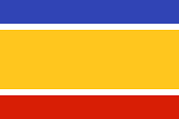
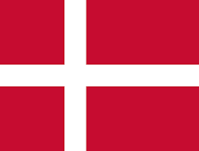
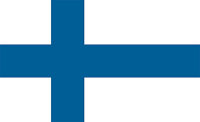


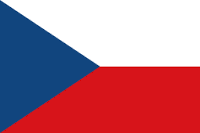
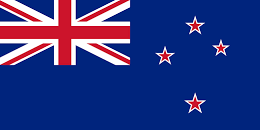
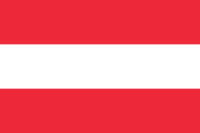
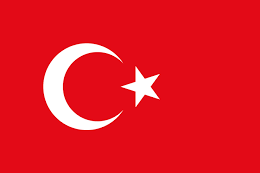
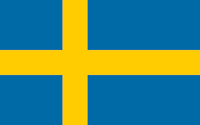
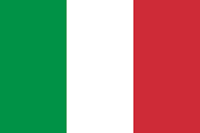
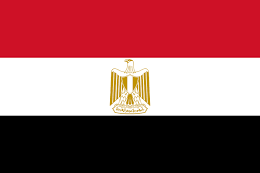

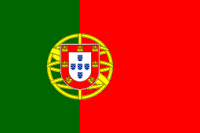
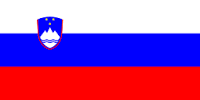

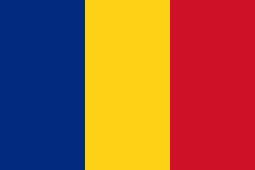
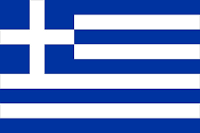

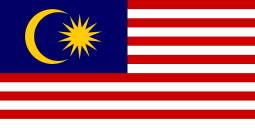
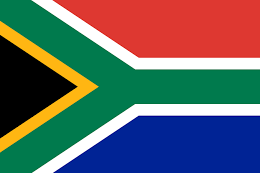

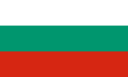
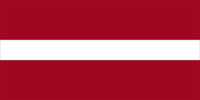


In this article written by Matthew Leibowitz, author of the book Xamarin Mobile Development for Android Cookbook, wants us to learn about the Android version that can be used as support for your project.
(For more resources related to this topic, see here.)
As the Android operating system evolves, many new features are added and older devices are often left behind.
In order to add the new features of the later versions of Android to the older versions of Android, all we need to do is add a small package:
If we want to add support for the new UI paradigm that uses fragments and action bars, we need to install two of the Android support packages:
There are several support library packages, each adding other types of forward compatibility, but these two are the most commonly used.
public class MyActivity : AppCompatActivity {
}
[Activity(..., Theme = "@style/Theme.AppCompat", ...)]
SupportActionBar.Title = "Xamarin Cookbook";
By simply using the action bar, all the options menu items are added as action items. However, all of them are added under the action bar overflow menu:
<menu ... >
<item
android_id="@+id/action_refresh"
android_icon="@drawable/ic_action_refresh"
android_title="@string/action_refresh"/>
</menu>
To get the menu items out of the overflow and onto the actual action bar, we can customize the items to be displayed and how they are displayed:
<menu ... >
<item ... app_showAsAction="ifRoom"/>
</menu>
<menu ... >
<item ... app_showAsAction="ifRoom|collapseActionView"/>
</menu>
<menu ... >
<item ...
app_actionViewClass="android.support.v7.widget.SearchView"/>
</menu>
<menu ... >
<item ... app_actionLayout="@layout/action_rating"/>
</menu>
As Android is developed, new features are added and designs change. We want to always provide the latest features to our users, but some users either haven't upgraded or can't upgrade to the latest version of Android.
Xamarin.Android provides three version numbers to specify which types can be used and how they can be used. The target framework version specifies what types are available for consumption as well as what toolset to use during compilation. This should be the latest as we always want to use the latest tools.
However, this will make some types and members available to apps even if they aren't actually available on the Android version that the user is using. For example, it will make the ActionBar type available to apps running on Android version 2.3. If the user were to run the app, it would probably crash.
In these instances, we can set the minimum Android version to be a version that supports these types and members. But, this will then reduce the number of devices that we can install our app on. This is why we use the support libraries; they allow the types to be used on most versions of Android.
Setting the minimum Android version for an app will prevent the app from being installed on devices with earlier versions of the OS.
By including the Android Support Libraries in our app, we can make use of the new features but still support the old versions.
Types from the Android Support Library are available to almost all versions of Android currently in use.
The Android Support Libraries provide us with a type that we know we can use everywhere, and then that base type manages the features to ensure that they function as expected. For example, we can use the ActionBar type on most versions of Android because the support library made it available through the AppCompatActivity type.
Because the AppCompatActivity type is an adaptive extension for the traditional Activity type, we have to use a different theme. This theme adjusts so that the new look and feel of the UI gets carried all the way back to the old Android versions.
When using the AppCompatActivity type, the activity theme must be one of the AppCompat theme variations.
There are a few differences in the use when using the support library. With native support for the action bar, the AppCompatActivity type has a property named ActionBar; however, in the support library, the property is named SupportActionBar. This is just a property name change but the functionality is the same.
Sometimes ,features have to be added to the existing types that are not in the support libraries. In these cases, static methods are provided. The native support for custom views in menu items includes a method named SetActionView():
menuItem.SetActionView(someView);
This method does not exist on the IMenuItem type for the older versions of Android, so we make use of the static method on the MenuItemCompat type:
MenuItemCompat.SetActionView(menuItem, someView);
While adding an action bar on older Android versions, it is important to inherit it from the AppCompatActivity type. This type includes all the logic required for including an action bar in the app. It also provides many different methods and properties for accessing and configuring the action bar. In newer versions of Android, all the features are included in the Activity type.
Although the functionality is the same, we do have to access the various pieces using the support members when using the support libraries. An example would be to use the SupportActionBar property instead of the ActionBar property. If we use the ActionBar property, the app will crash on devices that don't natively support the ActionBar property.
In order to render the action bar, the activity needs to use a theme that contains a style for the action bar or one that inherits from such a theme. For the older versions of Android, we can use the AppCompat themes, such as Theme.AppCompat.
With the release of Android version 5.0, Google introduced a new style of action bar. The new Toolbar type performs the same function as the action bar but can be placed anywhere on the screen. The action bar is always placed at the top of the screen, but a toolbar is not restricted to that location and can even be placed inside other layouts.
To make use of the Toolbar type, we can either use the native type, or we can use the type found in the support libraries. Like any Android View, we can add the ToolBar type to the layout:
<android.support.v7.widget.Toolbar
android_id="@+id/my_toolbar"
android_layout_width="match_parent"
android_layout_height="?attr/actionBarSize"
android_background="?attr/colorPrimary"
android_elevation="4dp"
android_theme="@style/ThemeOverlay.AppCompat.ActionBar"
app_popupTheme="@style/ThemeOverlay.AppCompat.Light"/>
The difference is in how the activity is set up. First, as we are not going to use the default ActionBar property, we can use the Theme.AppCompat.NoActionBar theme. Then, we have to let the activity know which view is used as the Toolbar type:
var toolbar = FindViewById<Toolbar>(Resource.Id.toolbar);
SetSupportActionBar(toolbar);
Action item buttons are just traditional options menu items but are optionally always visible on the action bar.
The underlying logic to handle item selections is the same as that for the traditional options menu. No change is required to be made to the existing code inside the OnOptionsItemSelected() method.
The value of the showAsAction attribute can be ifRoom, never, or always. This value can optionally be combined, using a pipe, with withText and/or collapseActionView.
Besides using the Android Support Libraries to handle different versions, there is another way to handle different versions at runtime. Android provides the version number of the current operating system through the Build.VERSION type.
This type has a property, SdkInt, which we use to detect the current version. It represents the current API level of the version. Each version of Android has a series of updates and new features. For example, Android 4 has numerous updates since its initial release, new features being added each time.
Sometimes, the support library cannot cover all the cases, and we have to write specific code for particular versions:
int apiLevel = (int)Build.VERSION.SdkInt;
if (Build.VERSION.SdkInt >= BuildVersionCodes.IceCreamSandwich) {
// Android version 4.0 and above
}
else {
// Android versions below version 4.0
}
Although the preceding can be done, it introduces spaghetti code and should be avoided. In addition to different code, the app may behave differently on different versions, even if the support library could have handled it. We will now have to manage these differences ourselves each time a new version of Android is released.
In this article, we learned that as the technology grows, new features are added and released in Android and older devices are often left behind. Thus, using the given steps we can add the new features of the later versions of Android to the older versions of Android, all we need to do is add packages by following the simple steps given in here.
Further resources on this subject: