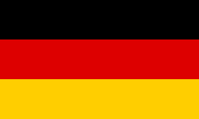




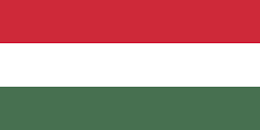

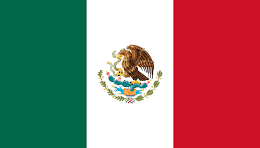
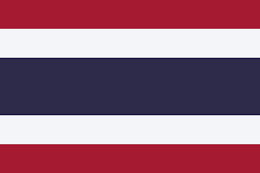
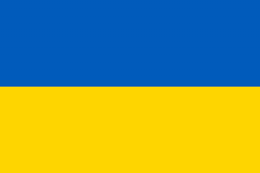
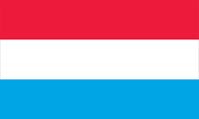

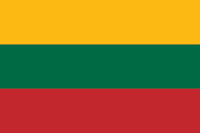

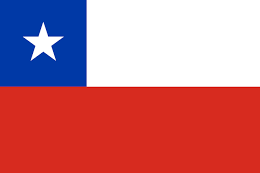
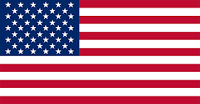

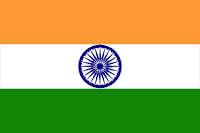
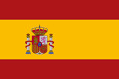

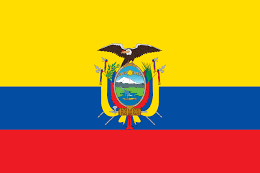

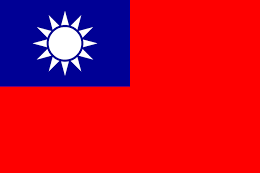

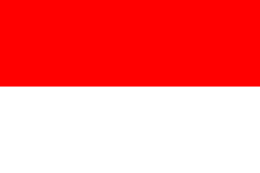
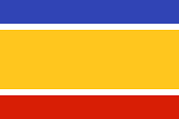
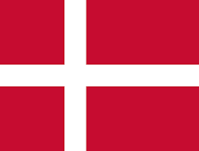
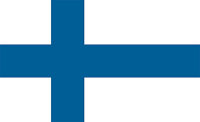


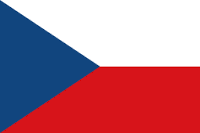
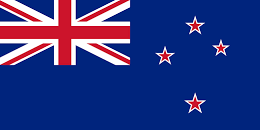
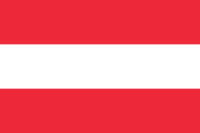
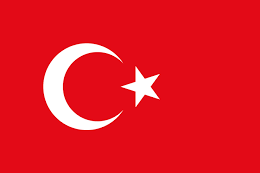
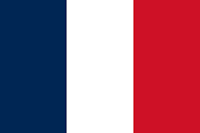
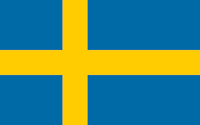
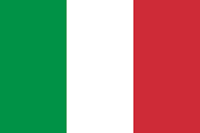
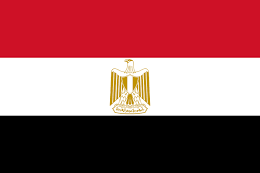

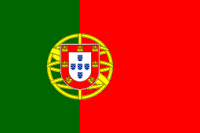
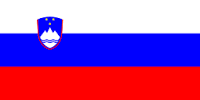

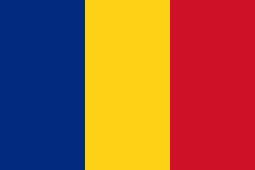
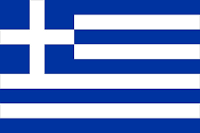

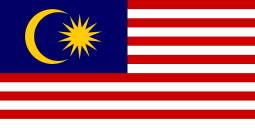
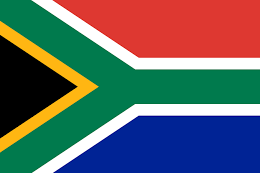

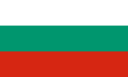
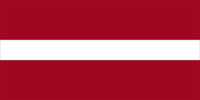


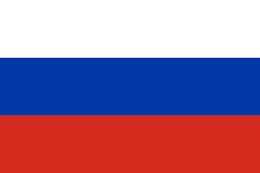
(For more resources related to this topic, see here.)
All Kendo Mobile widgets inherit from the base class kendo.mobile.ui.Widget, which is inherited from the base class of all Kendo widgets (both Web and Mobile), kendo.ui.Widget. The complete inheritance chain of the mobile widget class is shown in the following figure:
kendo.Class acts as the base class for most of the Kendo UI objects while the kendo.Observable object contains methods for events. kendo.data.ObservableObject which is the building block of Kendo MVVM, is inherited from kendo.Observable.
From the inheritance chain, all Kendo Mobile widgets inherit a set of common methods. A thorough understanding of these methods is required while building highly performing, complex mobile apps.
bind
The bind() method defined in the kendo.Observable class, attaches a handler to an event. Using this method we can attach custom methods to any mobile widget. The bind() method takes the following two input parameters:
The following example shows how to create a new mobile widget and attach a custom event to the widget:
//create a new mobile widget var mobileWidget = new kendo.mobile.ui.Widget(); //attach a custom event mobileWidget.bind("customEvent", function(e) { // mobileWidget object can be accessed
inside this function as //'e.sender' and 'this'. console.log('customEvent fired'); });
The event data is available in the object e. The object which raised the event is accessible inside the function as e.sender or using the this keyword.
trigger
The trigger() method executes all event handlers attached to the fired event. This method has two input parameters:
Let's see how trigger works by modifying the code sample provided for bind:
//create a mobile widget var mobileWidget = new kendo.mobile.ui.Widget(); //attach a custom event mobileWidget.bind("customEvent", function(e) { // mobileWidget object can be accessed //inside this function as //'e.sender' and 'this' . console.log('customEvent fired'); //read event specific data if it exists if(e.eventData !== undefined){ console.log('customEvent fired with data: ' + e.eventData); } }); //trigger the event with some data mobileWidget.trigger("customEvent", { eventData:'Kendo UI is cool!' });
Here we are triggering the custom event which is attached using the bind() method and sending some data along. This data is read inside the event and written to the console.
When this code is run, we can see the following output in the console:
customEvent fired customEvent fired with data: Kendo UI is cool!
unbind
The unbind() method detaches a previously attached event handler from the widget. It takes the following input parameters:
The following code attaches an event to a widget and detaches it when the event is triggered:
//create a mobile widget var mobileWidget = new kendo.mobile.ui.Widget(); //attach a custom event mobileWidget.bind("customEvent", function(e) { console.log('customEvent fired'); this.unbind("customEvent"); }); //trigger the event first time mobileWidget.trigger("customEvent"); //trigger the event second time mobileWidget.trigger("customEvent");
Output:
customEvent fired
As seen from the output, even though we trigger the event twice, only on the first time is the event handler invoked.
one
The one() method is identical to the bind() method only with one exception; the handler is unbound after its first invocation. So the handler will be fired only once.
To see this method in action, let's add a count variable to the existing sample code and track the number of times the handler is invoked. For this we will bind the event handler with one() and then trigger the event twice as shown in the following code:
//create a mobile widget var mobileWidget = new kendo.mobile.ui.Widget(); var count = 0; //attach a custom event mobileWidget.one("customEvent", function(e) { count++; console.log('customEvent fired. count: ' + count); }); //trigger the event first time mobileWidget.trigger("customEvent"); //trigger the event second time mobileWidget.trigger("customEvent");
Output:
customEvent fired. count: 1
If you replace the one() method with the bind() method, you can see that the handler will be invoked twice.
destroy
The destroy() method is inherited from the kendo.ui.Widget base object. The destroy() method kills all the event handler attachments and removes the widget object in the jquery.data() attribute so that the widget can be safely removed from the DOM without memory leaks. If there is a child widget available, the destroy() method of the child widget will also be invoked.
Let's see how the destroy() method works using the Kendo Mobile Button widget and using your browser's developer tools' console. Create an HTML file, add the following code along with Kendo UI Mobile file references in the file, and open it in your browser:
<div data-role="view" > <a class="button" data-role="button" id="btnHome"
data-click="buttonClick">Home</a> </div> <script> var app = new kendo.mobile.Application(document.body); function buttonClick(e){ console.log('Inside button click event handler...'); $("#btnHome").data().kendoMobileButton.destroy(); } </script>
In this code block, we created a Kendo Button widget and on the click event, we are invoking the destroy() method of the button.
Now open up your browser's developer tools' Console window, type $("#btnHome").data() and press Enter .
Now if you click on the Object link shown in the earlier screenshot, a detailed view of all properties can be seen:
Now click on kendoMobilebutton once and then again in the Console , type $("#btnHome").data() and hit Enter . Now we can see that the kendoMobileButton object is removed from the object list:
Even though the data object is gone, the button stays in the DOM without any data or events associated with it.
view
The view() method is specific to mobile widgets and it returns the view object in which the widget is loaded.
In the previous example, we can assign an ID, mainView, to the view and then retrieve it in the button's click event using this.view().id as shown in the following code snippet:
<div data-role="view" id="mainView" > <a class="button" data-role="button" id="btnHome"
data-click="buttonClick">Home</a> </div> <script> var app = new kendo.mobile.Application(document.body); function buttonClick(e){ console.log("View id: " + this.view().id ); } </script>
Output:
View id: #mainView